The Life Band is an innovative project aimed at providing real-time location tracking and health monitoring to ensure immediate assistance during emergencies. This project illustrates the practical application of STEM education to create devices that can significantly enhance personal safety and well-being. By combining location tracking and health monitoring, the Life Band ensures users can get timely help during emergencies, showcasing the power of technology in solving real-life problems.
Components Required
- Arduino Uno x1
- GPS NEO 6m x1
- Pulse Rate Sensor x1
- Breadboard and Jumper Wires
Components and Their Functions
- GPS Neo-6M Module
- Function: The GPS Neo-6M module is used to determine the precise location of the user. It receives signals from satellites and calculates geographical coordinates.
- Working: The module communicates with the Arduino via serial communication, sending the latitude and longitude data which can be transmitted to a pre-defined contact in case of an emergency.
- Pulse Rate Sensor
- Function: This sensor measures the user’s heart rate. It detects the blood volume changes in the tissues with each heartbeat and converts this information into a digital signal.
- Working: The pulse rate sensor outputs analog signals that the Arduino reads, processes, and translates into beats per minute (BPM).
- Arduino Uno
- Function: The Arduino Uno serves as the central processor, managing inputs from the GPS module and the pulse rate sensor, processing the data, and performing actions based on the readings.
- Working: It runs the code to process sensor inputs and execute the appropriate response, such as sending location data when an abnormal heart rate is detected.
Component Connections
- GPS Neo-6M Module Connection
- TX (Transmitter): Connect to Arduino digital pin 10 (GPS_PIN_RX).
- RX (Receiver): Connect to Arduino digital pin 11 (GPS_PIN_TX).
- VCC (Power): Connect to 3.3V or 5V on the Arduino.
- GND (Ground): Connect to the GND pin on the Arduino.
- Pulse Rate Sensor Connection
- Signal Pin (SIG): Connect to analog pin A3 on the Arduino (HEARTPULSE_PIN_SIG).
- VCC (Power): Connect to 5V on the Arduino.
- GND (Ground): Connect to the GND pin on the Arduino.
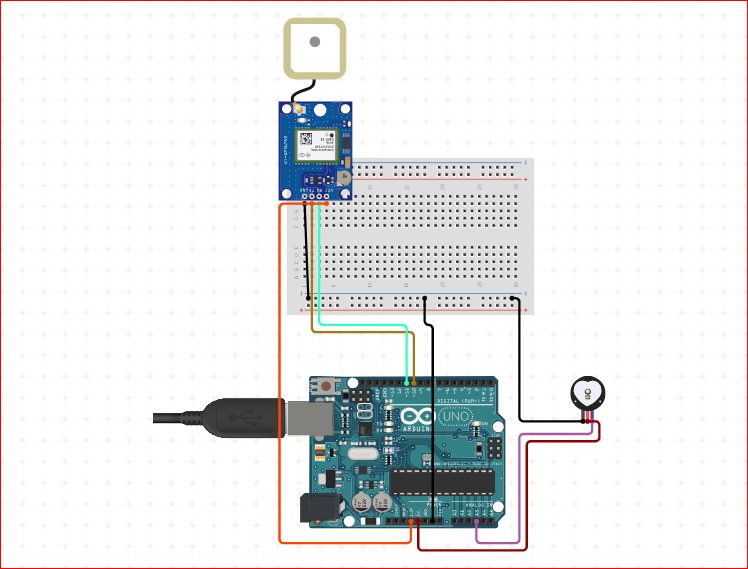
// Include Libraries
#include "Arduino.h"
#include "PulseSensorPlayground.h"
// Pin Definitions
#define GPS_PIN_TX 11
#define GPS_PIN_RX 10
#define HEARTPULSE_PIN_SIG A3
// Global variables and defines
// object initialization
PulseSensorPlayground pulseSensor;
// define vars for testing menu
const int timeout = 10000; // define timeout of 10 sec
char menuOption = 0;
long time0;
// Setup the essentials for your circuit to work. It runs first every time your circuit is powered with electricity.
void setup() {
// Setup Serial which is useful for debugging
// Use the Serial Monitor to view printed messages
Serial.begin(9600);
while (!Serial); // wait for serial port to connect. Needed for native USB
Serial.println("start");
// Initialize PulseSensor
pulseSensor.analogInput(HEARTPULSE_PIN_SIG);
pulseSensor.begin();
menuOption = menu();
}
// Main logic of your circuit. It defines the interaction between the components you selected. After setup, it runs over and over again, in an eternal loop.
void loop() {
if (menuOption == '1') {
// Disclaimer: The Ublox NEO-6M GPS Module is in testing and/or doesn't have code, therefore it may be buggy. Please be kind and report any bugs you may find.
} else if (menuOption == '2') {
// Heart Rate Pulse Sensor - Test Code
// Measure Heart Rate
int myBPM = pulseSensor.getBeatsPerMinute();
Serial.println(myBPM);
if (pulseSensor.sawStartOfBeat()) {
Serial.println("PULSE");
}
}
if (millis() - time0 > timeout) {
menuOption = menu();
}
}
// Menu function for selecting the components to be tested
// Follow serial monitor for instructions
char menu() {
Serial.println(F("\nWhich component would you like to test?"));
Serial.println(F("(1) Ublox NEO-6M GPS Module"));
Serial.println(F("(2) Heart Rate Pulse Sensor"));
Serial.println(F("(menu) send anything else or press on board reset button\n"));
while (!Serial.available());
// Read data from serial monitor if received
while (Serial.available()) {
char c = Serial.read();
if (isAlphaNumeric(c)) {
if (c == '1')
Serial.println(F("Now Testing Ublox NEO-6M GPS Module - note that this component doesn't have a test code"));
else if (c == '2')
Serial.println(F("Now Testing Heart Rate Pulse Sensor"));
else {
Serial.println(F("Illegal input!"));
return 0;
}
time0 = millis();
return c;
}
}
return 0; // Default return value if no valid input is received
}