Project Description:
To design a heart rate detector that measures and displays the heart rate of a user in beats per minute (BPM) using an Arduino microcontroller and a pulse sensor, So that people can keep track of their heart rates and do the necessary exercises to improve their health.
Components Required:
- Arduino Uno With Cable x 1
- Pulse sensor x 1
- Jumper wires – Male To male x 2
– Male To Female x 7 - LCD I2C display x 1
- breadboard small x 1
Connections:
Connection of Arduino with LCD I2C:
- Arduino pin 5v to VCC through a breadboard
- Arduino pin GND to GND through a breadboard
- Arduino pin A4 to SDA LCD I2C pin
- Arduino pin A5 to SCL LCD I2C pin
Connection of Arduino to Pulse Sensor
- Arduino pin A3 to signal the pulse sensor
- Arduino pin VCC to GND through a breadboard
- Arduino pin GND to GND through a breadboard
Conclusion
By following these steps, you can successfully create a heart rate detector that accurately measures and displays the user’s heart rate. This project is a good introduction to learning sensor interfacing and provides practical experience in working with Arduino and sensors, with a simplified hardware setup.
Circuit diagram:
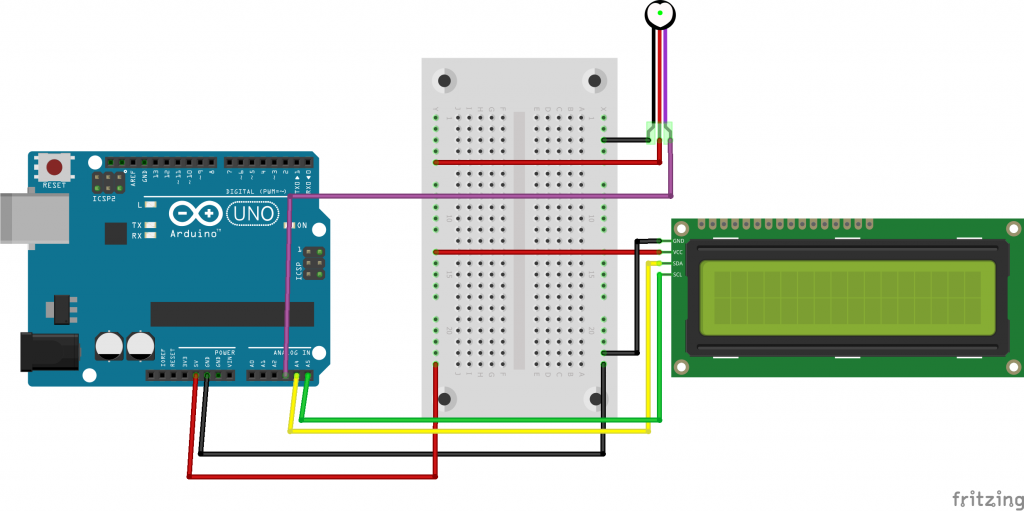
NOTE:
Before uploading and running the code IN ARDUINO IDE:
Go to > Sketch > Include Library > Manage Libraries… > Type the library name
OR Go to > Sketch > Include Library > Add .ZIP Library… download the zip library from the link given below.
1. PulseSensorPlayground – https://github.com/WorldFamousElectronics/PulseSensorPlayground
2. LiquidCrystal_I2C – https://github.com/fdebrabander/Arduino-LiquidCrystal-I2C-library
Code:
#include <PulseSensorPlayground.h>
#include <LiquidCrystal_I2C.h>
const int PULSE_SENSOR_PIN = A3;
const int LED_PIN = 13;
const int THRESHOLD = 550;
LiquidCrystal_I2C lcd(0x27, 16, 2);
PulseSensorPlayground pulseSensor;
void setup() {
Serial.begin(9600);
lcd.init(); //Start LCD Display
lcd.backlight();
pulseSensor.analogInput(PULSE_SENSOR_PIN);
pulseSensor.blinkOnPulse(LED_PIN);
pulseSensor.setThreshold(THRESHOLD);
if (pulseSensor.begin()) {
Serial.println("PulseSensor object created successfully!");
}
}
void loop() {
int currentBPM = pulseSensor.getBeatsPerMinute();
if (pulseSensor.sawStartOfBeat()) {
Serial.println("♥ A HeartBeat");
Serial.print("BPM: ");
Serial.println(currentBPM);
lcd.clear();
lcd.print(currentBPM);
}
delay(20);
}