This project uses a YF-S201 flow sensor, an I2C LCD display, and an Arduino to see which uses more water: a bucket or a shower. The flow sensor measures how much water is flowing, and the Arduino calculates the total amount. The I2C LCD display shows the results in real-time. Students can easily switch between measuring water for a bucket or a shower. This helps understand and compare water usage, promoting better water-saving habits.
Components Required
- Arduino Board (Arduino Uno) – 1
- YF-S201 Flow Sensor – 1
- I2C LCD Display (16×2) – 1
- Breadboard – 1
- Connecting Wires-1012(M-M and M-F)
Connections
- YF-S201 Flow Sensor
- Red Wire (VCC): Connect to Arduino 5V
- Black Wire (GND): Connect to Arduino GND
- Yellow Wire (Signal): Connect to Arduino digital pin D2
- I2C LCD Display
- GND: Connect to Arduino GND
- VCC: Connect to Arduino 5V
- SDA: Connect to Arduino A4
- SCL: Connect to Arduino A5
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Initialize the I2C LCD with I2C address 0x27 and 16x2 display
LiquidCrystal_I2C lcd(0x27, 16, 2);
const int flowSensorPin = 2;
const int buttonPin = 3;
volatile int pulseCount = 0;
unsigned long oldTime = 0;
float flowRate = 0.0;
float totalLitersBucket = 0.0;
float totalLitersShower = 0.0;
bool measureBucket = true; // true for bucket, false for shower
void setup() {
pinMode(flowSensorPin, INPUT_PULLUP);
pinMode(buttonPin, INPUT_PULLUP); // Enable internal pull-up resistor
lcd.init();
lcd.backlight();
lcd.print("Measuring Bucket");
attachInterrupt(digitalPinToInterrupt(flowSensorPin), pulseCounter, FALLING);
Serial.begin(9600);
}
void loop() {
// Check if the button is pressed
if (digitalRead(buttonPin) == LOW) {
delay(200); // Debounce delay
if (measureBucket) {
measureBucket = false;
lcd.clear();
lcd.print("Measuring Shower");
} else {
measureBucket = true;
lcd.clear();
lcd.print("Measuring Bucket");
}
delay(200); // Debounce delay
}
if ((millis() - oldTime) > 1000) { // Only process counters once per second
detachInterrupt(digitalPinToInterrupt(flowSensorPin));
flowRate = ((1000.0 / (millis() - oldTime)) * pulseCount) / 7.5; // In Liters per minute
oldTime = millis();
pulseCount = 0;
if (measureBucket) {
totalLitersBucket += flowRate / 60.0; // Convert to liters per second
lcd.setCursor(0, 1);
lcd.print("Bucket: ");
lcd.print(totalLitersBucket);
lcd.print(" L ");
} else {
totalLitersShower += flowRate / 60.0; // Convert to liters per second
lcd.setCursor(0, 1);
lcd.print("Shower: ");
lcd.print(totalLitersShower);
lcd.print(" L ");
}
Serial.print("Flow rate: ");
Serial.print(flowRate);
Serial.print(" L/min ");
Serial.print("Total liters bucket: ");
Serial.print(totalLitersBucket);
Serial.print(" L ");
Serial.print("Total liters shower: ");
Serial.print(totalLitersShower);
Serial.println(" L");
attachInterrupt(digitalPinToInterrupt(flowSensorPin), pulseCounter, FALLING);
}
}
void pulseCounter() {
pulseCount++;
}
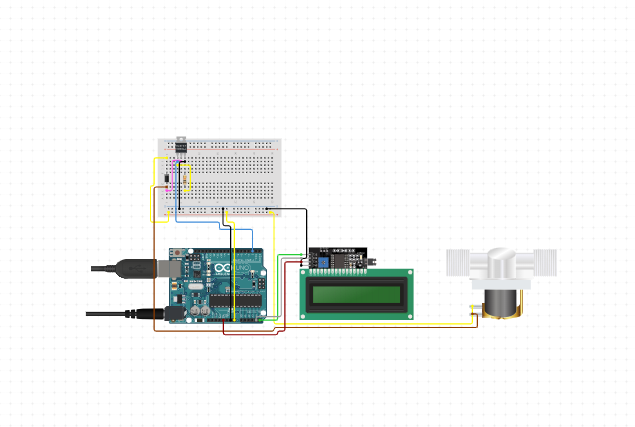