The voice-controlled bionic arm project integrates advanced technologies to create an intuitive and accessible robotic arm controlled via voice commands. The core of this project is an Arduino UNO R3, which orchestrates the operation of servo motors and a voice recognition module. This setup allows users to control the bionic arm by simply speaking commands such as “open,” “close,” and “stop.”
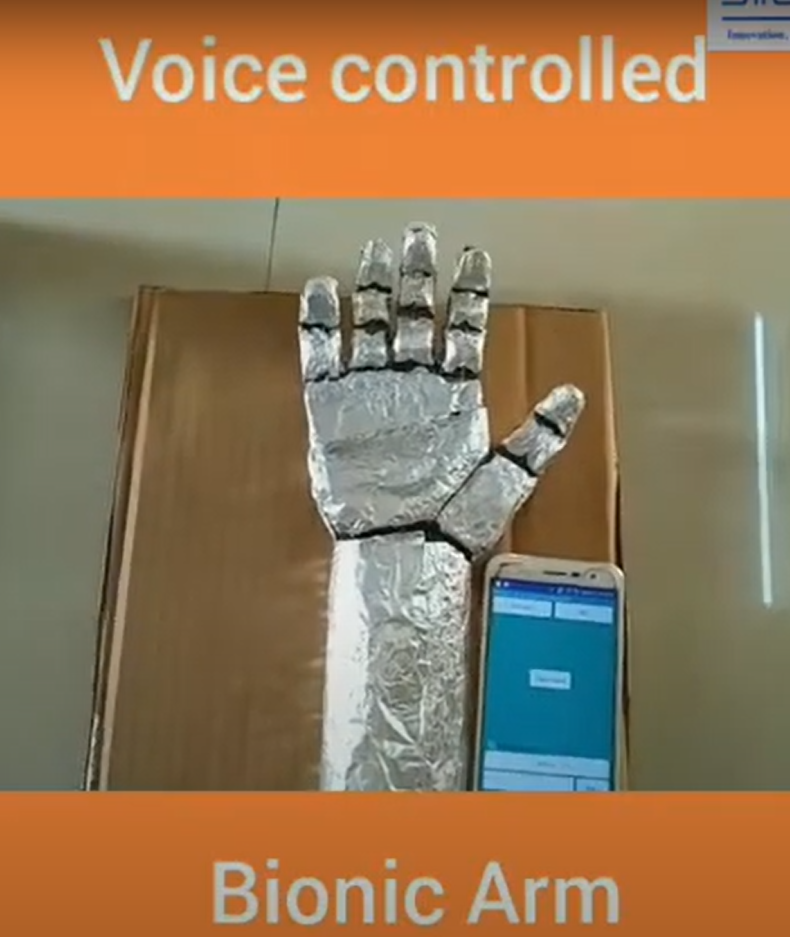
Component Required:
1.Arduino UNO R3
2.Voice Recognition Module
3.Servo Motor
Circuit diagram is given below:
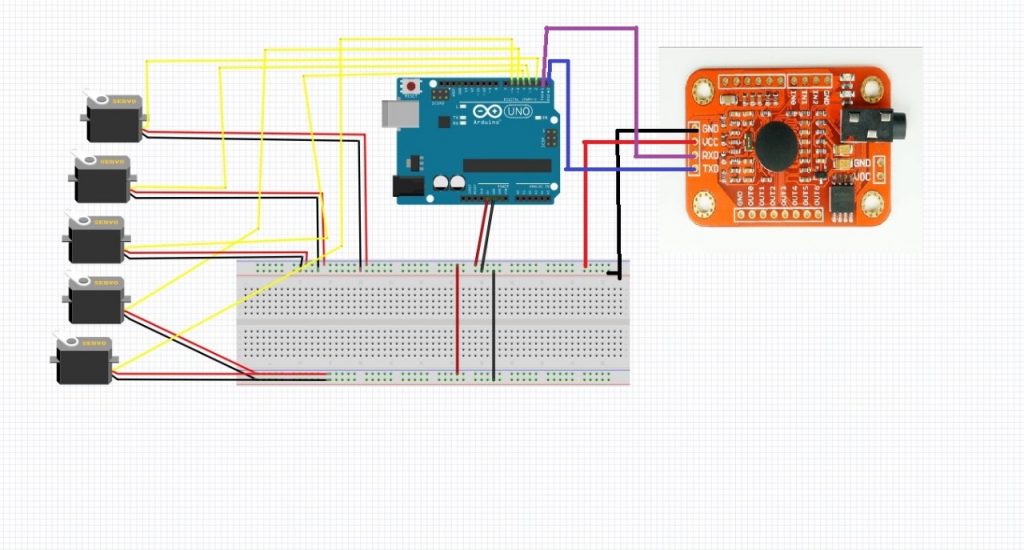
Working:
The voice recognition module, such as the Elechouse Voice Recognition Module V3, is connected to the Arduino using the SoftwareSerial library. It listens for pre-trained voice commands, translating them into specific actions performed by the servo motors. These servos are strategically placed to mimic the movements of a human arm, with each servo responsible for different joints and segments, enabling complex and precise motions.
The project also emphasizes proper power management. The servo motors, known for their high current consumption, are powered by an external power source to ensure stability and prevent overloading the Arduino. This setup is crucial for maintaining reliable performance during extended use.
The code is given below:
#include <Servo.h>
#include <SoftwareSerial.h>
Servo servo1;
Servo servo2;
Servo servo3;
Servo servo4;
Servo servo5;
SoftwareSerial voice(2, 3); // RX, TX
void setup() {
// Attach servos to pins
servo1.attach(2);
servo2.attach(3);
servo3.attach(4);
servo4.attach(5);
servo5.attach(6);
// Initialize serial communication with the voice module
voice.begin(9600);
Serial.begin(9600);
// Train the voice module with commands if necessary
// This will depend on the specific module and might need to be done beforehand
}
void loop() {
if (voice.available()) {
int command = voice.read();
Serial.println(command);
// Map commands to servo movements
switch (command) {
case 1: // Command 1: Move Servo 1
servo1.write(90); // Example position
break;
case 2: // Command 2: Move Servo 2
servo2.write(90); // Example position
break;
case 3: // Command 3: Move Servo 3
servo3.write(90); // Example position
break;
case 4: // Command 4: Move Servo 4
servo4.write(90); // Example position
break;
case 5: // Command 5: Move Servo 5
servo5.write(90); // Example position
break;
default:
// Handle unknown commands
break;
}
}
}
The codebase for this project is designed to be straightforward and modular, allowing for easy expansion with additional commands or more sophisticated movements. The system continuously listens for voice inputs, processes them, and actuates the servos accordingly.
This voice-controlled bionic arm not only showcases the practical applications of Arduino in robotics but also highlights the potential for creating assistive technologies that enhance human capabilities. It offers a glimpse into the future of intuitive, human-centric robotic control, making technology more accessible and user-friendly.