This innovative project is designed to provide continuous and efficient dust cleaning using Arduino Uno. By combining two DC motors with brushes and a fan acting as a vacuum cleaner, this system offers an automated solution for maintaining cleanliness in various environments. Swachh Bharat Truck project is instrumental in public spaces, commercial facilities, and homes, reducing the need for manual labor and enhancing overall hygiene.
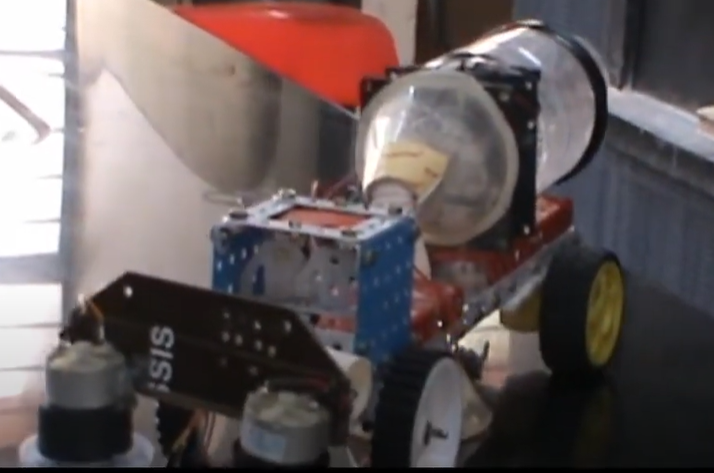
Components Required
- Arduino Uno
- 2 DC Motors
- 1 Fan (DC motor for vacuum)
- Motor Driver (e.g., L298N)
- Power supply for motors and fan
- Breadboard
- Jumper wires
Circuit Connections
- Motors:
- Motor 1:
- Connect Motor 1’s terminals to Motor Driver outputs (e.g., OUT1 and OUT2 of L298N).
- Motor Driver IN1 to Arduino digital pin 2.
- Motor Driver IN2 to Arduino digital pin 3.
- Motor 2:
- Connect Motor 2’s terminals to Motor Driver outputs (e.g., OUT3 and OUT4 of L298N).
- Motor Driver IN3 to Arduino digital pin 4.
- Motor Driver IN4 to Arduino digital pin 5.
- Motor 1:
- Fan (Vacuum Cleaner):
- Connect the fan’s power leads directly to the appropriate power supply, ensuring correct voltage and current ratings.
- Optionally, control the fan using a relay connected to Arduino digital pin 6 if fan switching is desired.
- Power Supply:
- Ensure the power supply can handle the combined load of both motors and the fan.
- Connect the motor driver’s VCC and GND to the power supply and Arduino GND.
Circuit diagram is given below:
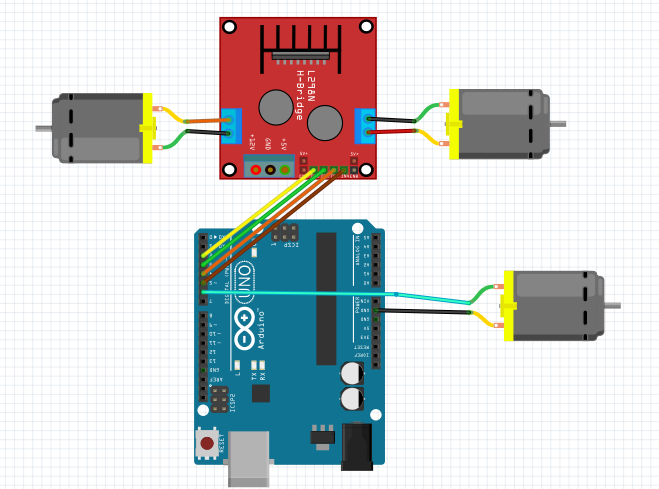
Working
- Motor Control:
- The Arduino Uno controls two DC motors to rotate in opposite directions, enabling the brushes to sweep dust effectively.
- The motor driver receives signals from Arduino pins to manage motor rotation.
- Fan Operation:
- The fan creates suction to vacuum dust into a collection tank, operating continuously to ensure persistent cleaning.
The code is given below:
// Motor control pins
const int motor1Pin1 = 2; // Motor 1 pin 1 connected to Arduino digital pin 2
const int motor1Pin2 = 3; // Motor 1 pin 2 connected to Arduino digital pin 3
const int motor2Pin1 = 4; // Motor 2 pin 1 connected to Arduino digital pin 4
const int motor2Pin2 = 5; // Motor 2 pin 2 connected to Arduino digital pin 5
// Fan control pin
const int fanPin = 6; // Fan control pin connected to Arduino digital pin 6
void setup() {
// Initialize motor control pins as outputs
pinMode(motor1Pin1, OUTPUT);
pinMode(motor1Pin2, OUTPUT);
pinMode(motor2Pin1, OUTPUT);
pinMode(motor2Pin2, OUTPUT);
// Initialize fan control pin as output
pinMode(fanPin, OUTPUT);
// Start with motors off
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, LOW);
// Start fan
digitalWrite(fanPin, HIGH); // Assuming HIGH turns on the fan
}
void loop() {
// Control motors to rotate in opposite directions
digitalWrite(motor1Pin1, HIGH); // Motor 1 direction 1
digitalWrite(motor1Pin2, LOW); // Motor 1 direction 2
digitalWrite(motor2Pin1, LOW); // Motor 2 direction 1
digitalWrite(motor2Pin2, HIGH); // Motor 2 direction 2
// Continuous operation until power is cut
}
Features
- Continuous Operation:
- The system operates nonstop until the power supply is cut, ensuring ongoing cleanliness.
- Automated Cleaning:
- Reduces manual labor and enhances hygiene in public spaces, commercial facilities, and homes.
- Efficient Dust Collection:
- The combination of brushing and vacuuming ensures thorough dust removal.
- Adaptability:
- It can be used in various environments, including industrial settings, to maintain machinery and production areas.