The Smart Car Anti-Theft System protects the vehicle’s internal components, such as the battery and parts under the bonnet. Using an ADXL335 accelerometer, it detects unauthorized movements. An Arduino processes this data and, if tampering is detected, activates an LED and buzzer to provide immediate visual and audible alerts. This system ensures real-time notifications for the user, deterring theft and safeguarding valuable car parts effectively.
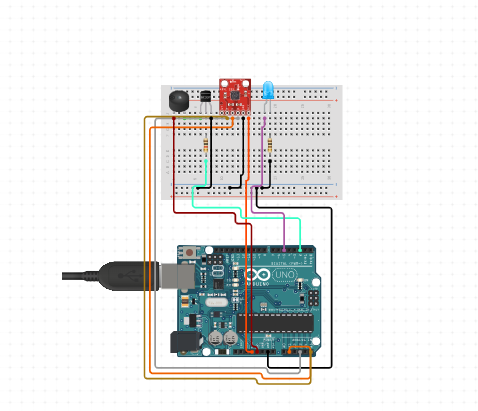
// Pin definitions
#define LED_PIN 5
#define BUZZER_PIN 2
#define X_PIN A0
#define Y_PIN A1
#define Z_PIN A2
// Threshold for detecting significant acceleration
#define THRESHOLD 400 // Adjust threshold as needed
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Initialize LED and Buzzer pins
pinMode(LED_PIN, OUTPUT);
pinMode(BUZZER_PIN, OUTPUT);
}
void loop() {
// Read accelerometer data
int x = analogRead(X_PIN);
int y = analogRead(Y_PIN);
int z = analogRead(Z_PIN);
// Print accelerometer data
Serial.print("X: ");
Serial.print(x);
Serial.print(" Y: ");
Serial.print(y);
Serial.print(" Z: ");
Serial.println(z);
// Check if acceleration on any axis exceeds threshold
if (abs(x - 512) > THRESHOLD || abs(y - 512) > THRESHOLD || abs(z - 512) > THRESHOLD) {
// Turn on LED and Buzzer if threshold exceeded
digitalWrite(LED_PIN, HIGH);
digitalWrite(BUZZER_PIN, HIGH);
} else {
// Turn off LED and Buzzer if below threshold
digitalWrite(LED_PIN, LOW);
digitalWrite(BUZZER_PIN, LOW);
}
// Delay before next reading
delay(100);
}