This project is made to help blind and dumb individuals by using simple robotics with everyday functionality. It consists of Arduino Uno, ultrasonic sensors, LCD display, pushbuttons and buzzer. This project is not only practical but also makes learning robotics fun for kids by allowing them to see the real-world applications of their creations.
The system is controlled by an Arduino Uno, which processes inputs from the buttons and ultrasonic sensors. The Arduino then displays the appropriate message on the LCD or activates the buzzer as needed. This combination of components ensures that the device is responsive and reliable, providing immediate feedback to the user.
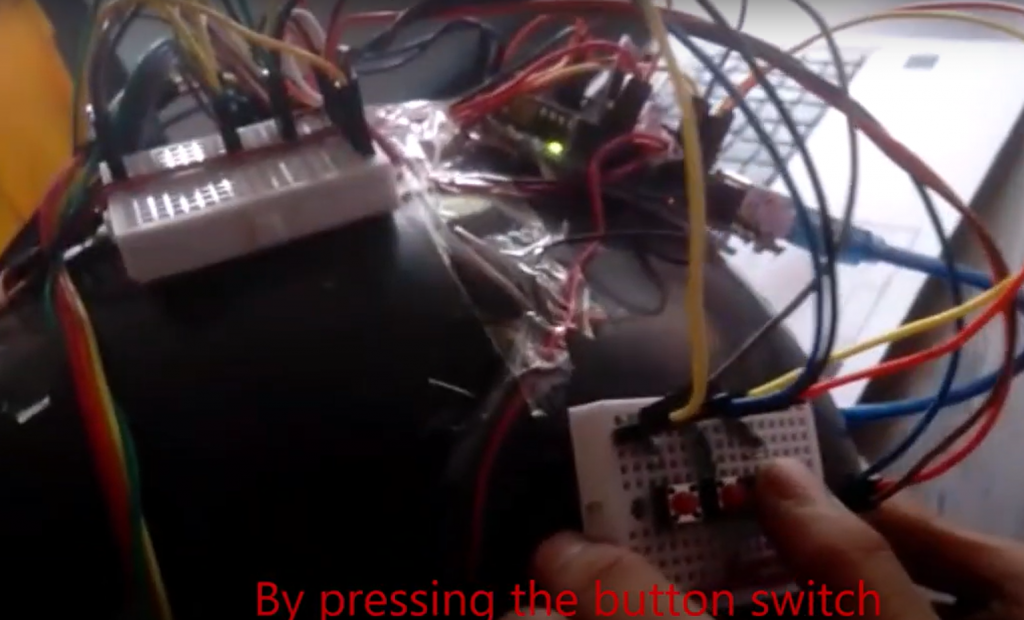
The project features three ultrasonic sensors mounted on a helmet to detect obstacles within 5 cm. If any sensor detects an obstacle, it triggers a buzzer to alert the wearer, helping blind individuals navigate their environment safely.
The device consists of three push buttons, each assigned a specific message: “I’m hungry,” “I’m thirsty,” and “I need help.” When a button is pressed, the corresponding message is displayed on a 16×2 I2C LCD screen. This enables mute individuals to communicate their needs easily.
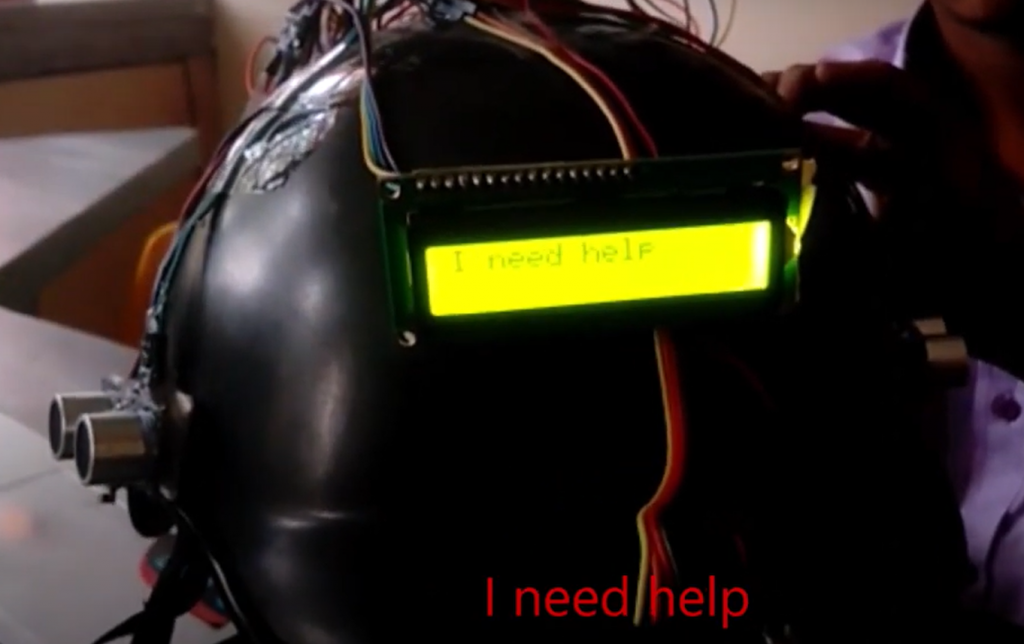
The circuit diagram is given below. You can make the connections according to the diagram.
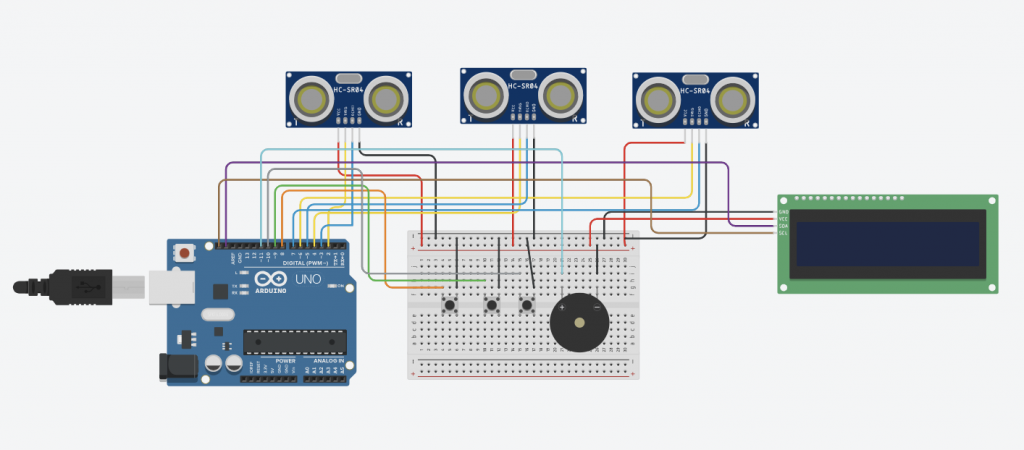
Before uploading the code, you have to download the following libraries:
1. LiquidCrystal_I2C.h
2. Wire.h
3. NewPing.h
The Code is given below:
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <NewPing.h>
// Define pin numbers
const int buttonPin1 = 8;
const int buttonPin2 = 9;
const int buttonPin3 = 10;
const int buzzerPin = 11;
const int trigPin1 = 2;
const int echoPin1 = 3;
const int trigPin2 = 4;
const int echoPin2 = 5;
const int trigPin3 = 6;
const int echoPin3 = 7;
// Initialize the LCD library with the I2C address
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Define ultrasonic sensor parameters
#define MAX_DISTANCE 200 // Maximum distance we want to measure (in cm)
NewPing sonar1(trigPin1, echoPin1, MAX_DISTANCE);
NewPing sonar2(trigPin2, echoPin2, MAX_DISTANCE);
NewPing sonar3(trigPin3, echoPin3, MAX_DISTANCE);
// Variable to store the last displayed message
String lastMessage = "";
void setup() {
// Initialize the LCD
lcd.begin();
lcd.backlight();
// Set button pins as input
pinMode(buttonPin1, INPUT_PULLUP);
pinMode(buttonPin2, INPUT_PULLUP);
pinMode(buttonPin3, INPUT_PULLUP);
// Set buzzer pin as output
pinMode(buzzerPin, OUTPUT);
// Print a message to the LCD.
lcd.print("Ready");
lastMessage = "Ready";
}
void loop() {
// Check button states
bool buttonPressed = false;
if (digitalRead(buttonPin1) == LOW) {
lcd.clear();
lcd.print("I'm hungry");
delay(1000); // Debounce delay
lastMessage = "I'm hungry";
buttonPressed = true;
} else if (digitalRead(buttonPin2) == LOW) {
lcd.clear();
lcd.print("I'm thirsty");
delay(1000); // Debounce delay
lastMessage = "I'm thirsty";
buttonPressed = true;
} else if (digitalRead(buttonPin3) == LOW) {
lcd.clear();
lcd.print("I need help");
delay(1000); // Debounce delay
lastMessage = "I need help";
buttonPressed = true;
}
// Check ultrasonic sensors
int distance1 = sonar1.ping_cm();
int distance2 = sonar2.ping_cm();
int distance3 = sonar3.ping_cm();
bool objectDetected = (distance1 > 0 && distance1 < 5) || (distance2 > 0 && distance2 < 5) || (distance3 > 0 && distance3 < 5);
if (objectDetected) {
digitalWrite(buzzerPin, HIGH);
} else {
digitalWrite(buzzerPin, LOW);
}
// Show "I'm okay" when no buttons are pressed and no object is detected
if (!buttonPressed && !objectDetected) {
if (lastMessage != "I'm okay") {
lcd.clear();
lcd.print("I'm okay");
lastMessage = "I'm okay";
}
}
// Small delay to prevent bouncing issues
delay(50);
}
Application:
Communication Aid for the Speech and Hearing Impaired:
- Visual Communication: The LCD display provides instant visual feedback based on button presses, allowing users to convey basic needs like hunger, thirst, or a request for assistance.
- Independence: Enables users to communicate their immediate needs without relying on verbal communication, enhancing their independence in daily activities.
Obstacle Detection and Safety Enhancement:
- Object Detection: Ultrasonic sensors detect nearby objects within a 5cm range, triggering the buzzer to alert users to potential obstacles.
- Navigation Assistance: Alerts users to obstacles around them, aiding in safe navigation in unfamiliar or crowded environments.
Emergency Alert System:
- Help Request: The “I need help” message displayed on the LCD can act as an emergency alert signal.
- Buzzer Activation: The buzzer’s sound can attract attention and signal others nearby that assistance is needed.
Assistive Technology for Accessibility:
- Accessibility Enhancement: Provides essential communication and safety features tailored to the needs of individuals with visual and speech impairments.
- User-Friendly Interface: Simple buttons press interactions and clear visual feedback make the system easy to use and understand.