The Elevated Ambulance Guidance System is designed to provide efficient and adaptive lighting to guide ambulances during nighttime, ensuring safety and visibility while conserving energy. This smart lighting system uses an Arduino Uno, IR sensors, and LEDs to dynamically light up the path as the ambulance moves, reducing unnecessary power consumption.
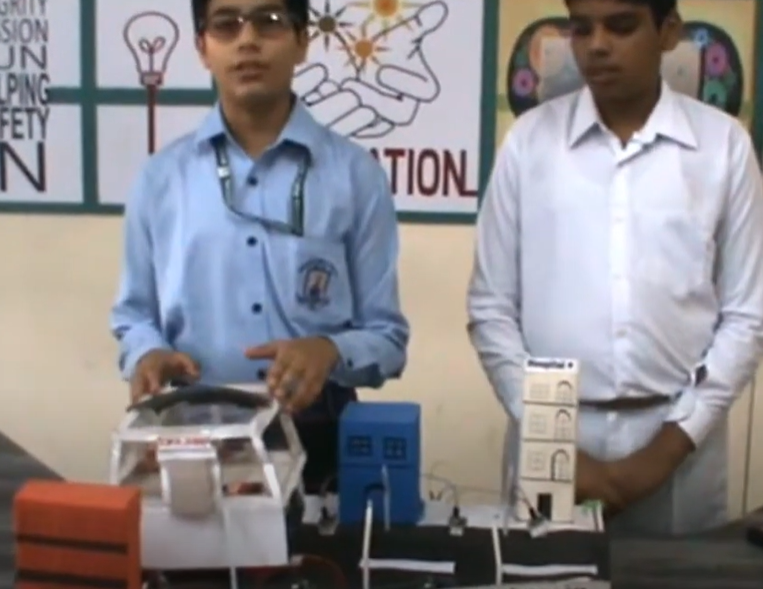
Components Required
- Arduino Uno
- 4 IR Sensors
- 4 LEDs
- 4 Resistors (220 ohms)
- Jumper wires
- Breadboard
Circuit Connection
- IR Sensors:
- IR1: Output pin to Arduino digital pin 2
- IR2: Output pin to Arduino digital pin 3
- IR3: Output pin to Arduino digital pin 4
- IR4: Output pin to Arduino digital pin 5
- LEDs:
- LED1: Anode to Arduino digital pin 8, cathode to ground through a 220-ohm resistor
- LED2: Anode to Arduino digital pin 9, cathode to ground through a 220-ohm resistor
- LED3: Anode to Arduino digital pin 10, cathode to ground through a 220-ohm resistor
- LED4: Anode to Arduino digital pin 11, cathode to ground through a 220-ohm resistor
The circuit diagram is given below:
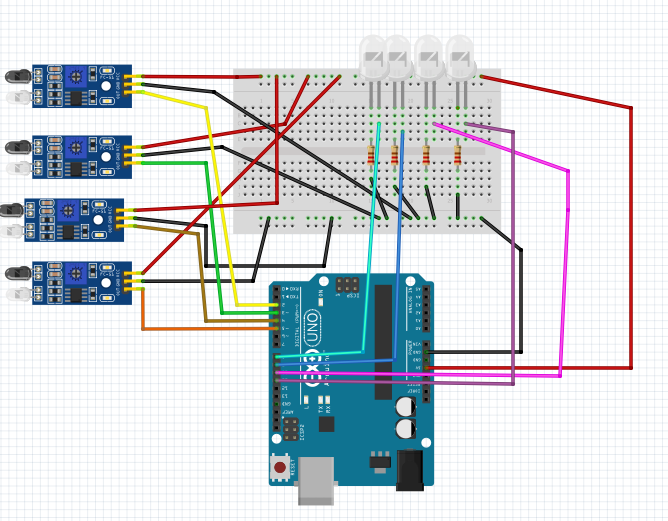
Working
The system ensures the first and last LEDs are always on, marking the beginning and end of the path. The second LED lights up when either the first or second IR sensor detects the ambulance, and the third LED lights up when either the third or fourth IR sensor detects the ambulance. This adaptive lighting approach illuminates only the necessary sections of the path, enhancing visibility and saving energy.
The code is given below:
// Pin definitions
const int IR1_PIN = 2;
const int IR2_PIN = 3;
const int IR3_PIN = 4;
const int IR4_PIN = 5;
const int LED1_PIN = 8;
const int LED2_PIN = 9;
const int LED3_PIN = 10;
const int LED4_PIN = 11;
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Initialize IR sensor pins as inputs
pinMode(IR1_PIN, INPUT);
pinMode(IR2_PIN, INPUT);
pinMode(IR3_PIN, INPUT);
pinMode(IR4_PIN, INPUT);
// Initialize LED pins as outputs
pinMode(LED1_PIN, OUTPUT);
pinMode(LED2_PIN, OUTPUT);
pinMode(LED3_PIN, OUTPUT);
pinMode(LED4_PIN, OUTPUT);
// Turn on the first and last LEDs
digitalWrite(LED1_PIN, HIGH);
digitalWrite(LED4_PIN, HIGH);
}
void loop() {
// Read IR sensor values
int ir1State = digitalRead(IR1_PIN);
int ir2State = digitalRead(IR2_PIN);
int ir3State = digitalRead(IR3_PIN);
int ir4State = digitalRead(IR4_PIN);
// Debugging output
Serial.print("IR1: ");
Serial.print(ir1State);
Serial.print(" | IR2: ");
Serial.print(ir2State);
Serial.print(" | IR3: ");
Serial.print(ir3State);
Serial.print(" | IR4: ");
Serial.println(ir4State);
// Control LED2 based on IR1 and IR2
if (ir1State == HIGH || ir2State == HIGH) {
digitalWrite(LED2_PIN, HIGH);
} else {
digitalWrite(LED2_PIN, LOW);
}
// Control LED3 based on IR3 and IR4
if (ir3State == HIGH || ir4State == HIGH) {
digitalWrite(LED3_PIN, HIGH);
} else {
digitalWrite(LED3_PIN, LOW);
}
delay(100); // Short delay for stability
}
Features
- Adaptive Lighting: Lights up the path as the ambulance moves, based on IR sensor detection.
- Energy Efficiency: Reduces power consumption by illuminating only the required sections.
- Enhanced Safety: Provides clear guidance for ambulances during nighttime operations.
- Automated Control: Operates automatically, reducing the need for manual intervention.
Application:
Hospitals and Healthcare Facilities
In hospitals, the Elevated Ambulance Guidance System ensures that ambulances can navigate to emergency entrances swiftly and safely during nighttime hours. The adaptive lighting system provides clear guidance without excessive power consumption, optimizing operational efficiency and enhancing patient care.
Urban and Suburban Roads
On urban and suburban roads, the system improves emergency response times by guiding ambulances through intersections and complex road networks. It reduces the risk of delays and accidents by ensuring ambulances have well-lit pathways, thereby potentially saving lives during critical moments.
High-Traffic Areas and Events
During large-scale events or in high-traffic areas, such as concerts, festivals, or sporting events, the system facilitates efficient ambulance navigation. It ensures that emergency vehicles can access incident sites quickly and safely, even in crowded and dimly lit environments.
Industrial and Commercial Zones
In industrial complexes and commercial zones, where visibility may be limited at night, the system aids emergency responders by illuminating designated routes. This enhances safety for both ambulance crews and on-site personnel, ensuring rapid access to medical assistance when needed.
Rural and Remote Locations
In rural or remote locations with limited infrastructure and challenging terrain, the system provides essential guidance for ambulances navigating narrow roads or unfamiliar pathways. It enhances emergency preparedness and response capabilities in areas where visibility and accessibility are critical factors.