The Auto Irrigation System is a smart way to water plants automatically. It uses an Arduino Uno, a soil moisture sensor, and a water pump controlled by a relay. This system waters the plants when they need it, without any manual effort. It shows how sensor technology and automation can be used in everyday life, making the idea of robotics easy and fun to understand.
The system works by constantly checking how wet the soil is using a soil moisture sensor connected to the Arduino. The sensor measures the moisture in the soil and gives a value between 0 and 1023. The Arduino then converts this value into a percentage, from 0% to 100%.
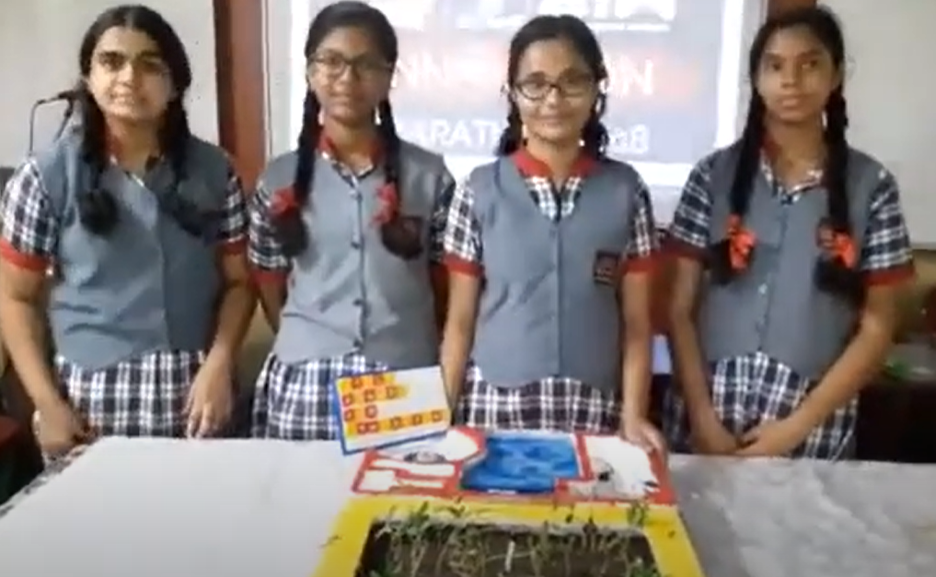
When the moisture level falls below a predefined threshold of 80%, the Arduino activates a relay, turning on the water pump to irrigate the soil. The pump remains on until the soil moisture reaches the threshold value, at which point the Arduino deactivates the relay, turning off the pump. This ensures efficient water usage and consistent soil hydration.
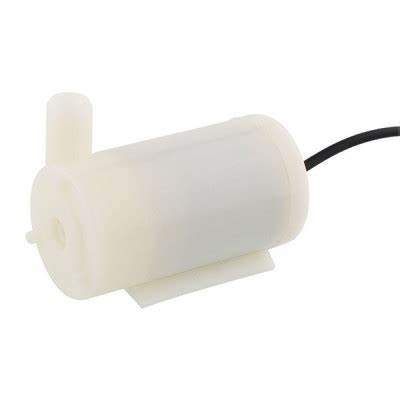
The circuit connections and code is given below. Add a water pump to the relay module.
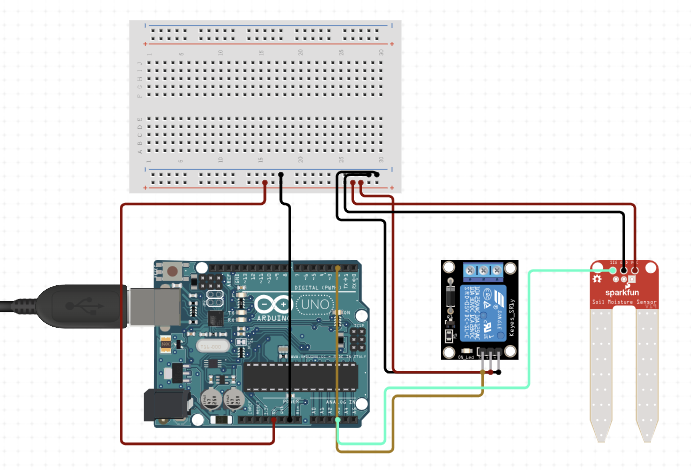
// Define pins
const int soilMoisturePin = A3; // Analog pin for soil moisture sensor
const int relayPin = 2; // Digital pin for relay
// Define threshold value (in percentage)
const int moistureThreshold = 80;
void setup() {
// Initialize serial communication for debugging
Serial.begin(9600);
// Initialize relay pin as output
pinMode(relayPin, OUTPUT);
// Turn off the relay initially
digitalWrite(relayPin, LOW);
}
void loop() {
// Read the soil moisture value (0-1023)
int moistureValue = analogRead(soilMoisturePin);
// Convert the moisture value to percentage (0-100)
int moisturePercentage = map(moistureValue, 0, 1023, 100, 0);
// Print the moisture percentage for debugging
Serial.print("Soil Moisture: ");
Serial.print(moisturePercentage);
Serial.println("%");
// Check if moisture level is below the threshold
if (moisturePercentage < moistureThreshold) {
// Turn on the pump
digitalWrite(relayPin, HIGH);
} else {
// Turn off the pump
digitalWrite(relayPin, LOW);
}
// Wait for a short period before taking the next reading
delay(1000);
}
Application:
Home Gardening:
- Ideal for automating watering tasks in home gardens, ensuring plants receive optimal moisture levels without manual intervention. This promotes healthier plant growth and reduces the risk of over or under-watering.
Commercial Agriculture:
- Used in large-scale agricultural settings to manage irrigation across fields or greenhouse environments. Automating watering processes improves efficiency, conserves water resources, and enhances crop yield and quality.
Botanical Gardens and Public Parks:
- Implemented to maintain green spaces and landscapes in botanical gardens, parks, and recreational areas. Automated irrigation ensures consistent plant care while reducing maintenance costs and labor.