This project involves creating an alcohol detector car using an MQ sensor, Arduino, and a DC motor. The MQ sensor detects the presence of alcohol, and if alcohol is detected, the Arduino will stop the DC motor, effectively halting the car. This setup aims to promote safety by preventing the car from operating when alcohol is detected, thereby reducing the risk of accidents caused by drunk driving.
// Define pin assignments
const int mqPin = A3; // Analog pin for MQ sensor
const int MOTORPin = 5; // LED pin (built-in LED on most Arduino boards)
// Define threshold value for alcohol detection
const int alcoholThreshold = 300; // Adjust this value based on your sensor calibration
void setup() {
Serial.begin(9600); // Initialize serial communication for debugging
pinMode(mqPin, INPUT); // MQ sensor pin as input
pinMode(MOTORPin, OUTPUT);// LED pin as output
}
void loop() {
// Read sensor value
int alcoholLevel = analogRead(mqPin);
// Print alcohol level (for debugging purposes)
Serial.print("Alcohol Level: ");
Serial.println(alcoholLevel);
// Check if alcohol level exceeds threshold
if (alcoholLevel > alcoholThreshold) {
digitalWrite(MOTORPin, LOW); // Turn on LED if alcohol detected
} else {
digitalWrite(MOTORPin, HIGH); // Turn off LED if no alcohol detected
}
delay(1000); // Adjust delay as needed
}
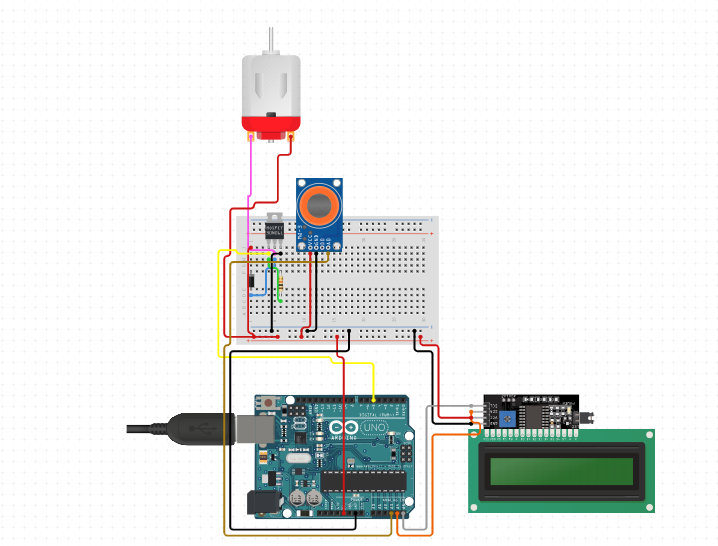