The Waste Segregator is like a high-tech trash sorter that makes recycling and managing waste super easy. It uses fancy sensors and motors to figure out if something is recyclable, organic (like food waste), or just regular trash. This helps us be kinder to the planet by making sure we recycle as much as possible and dispose of waste responsibly. It’s a cool example of how technology can make a big difference in protecting our environment and keeping our communities clean and healthy. Additionally, the Smart Segregator teaches us about the importance of recycling and taking care of our environment. It’s a fun and educational way to learn how our everyday actions, like sorting trash correctly, can have a positive impact on the world around us.
Components Required:
- Servo Motors: Two servo motors (servo1 and servo2) are used to control the movement of segregation bins based on the waste category detected.
- Ultrasonic Sensor: The ultrasonic sensor, connected to trigPin (pin 12) and echoPin (pin 11), measures the distance of waste items in the disposal area.
- Soil Moisture Sensor: The soil moisture sensor, connected to Pin (A0), determines the moisture level in the soil, possibly indicating the presence of organic waste.
Circuit Diagram and Setup:
- The setup involves connecting the components as follows:
- Attach servo1 to pin 8 and servo2 to pin 9 for controlling the segregation bins.
- Connect the ultrasonic sensor to trigPin and echoPin for distance measurement.
- Connect the soil moisture sensor to potPin to detect soil moisture levels.
- Initialize the sensors and servo motors in the setup() function.
- In the loop() function, the system continuously measures the distance using the ultrasonic sensor and the soil moisture using the soil moisture sensor.
- Based on the measured distance and moisture level, the system moves the servo motors to segregate waste into appropriate bins (e.g., wet waste triggering servo movement to 180 degrees, dry waste to 0 degrees).
- The system then resets the servo motors to 90 degrees for the next cycle.
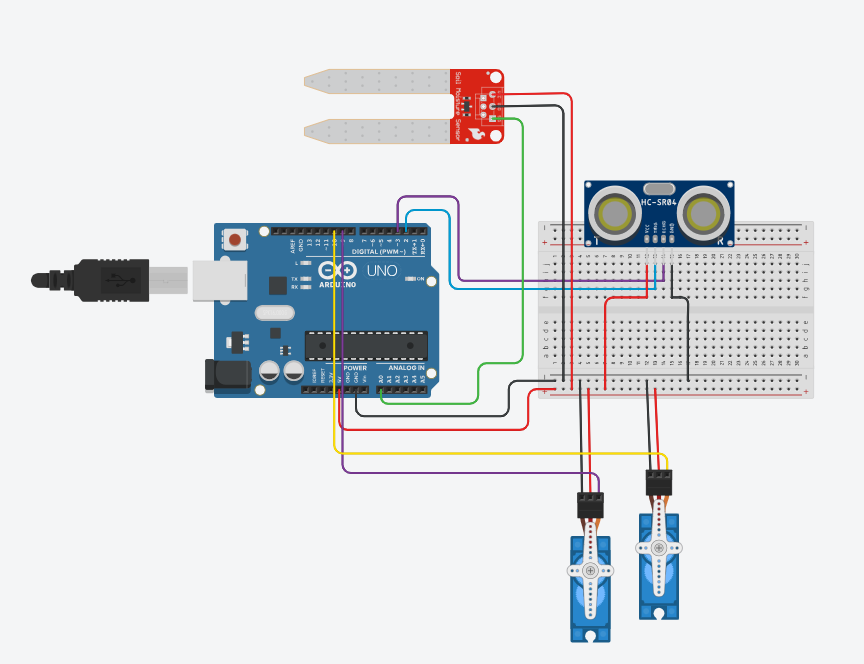
Initialization:
- Setup and configure all hardware components, including servo motors, sensors, and microcontrollers.
- Define pin assignments, communication protocols, and initial parameter values.
- Implement calibration routines for sensors to ensure accurate readings.
Sensor Reading:
- Continuously read data from the ultrasonic sensor to measure the distance of waste items.
- Monitor soil moisture levels using the soil moisture sensor at regular intervals.
- Apply filtering and averaging techniques to improve sensor accuracy and reliability.
Decision Making:
- Develop algorithms to analyze sensor data and make decisions based on predefined thresholds.
- Determine waste categories (recyclable, organic, non-recyclable) based on distance and moisture level readings.
- Incorporate logic for exception handling and error detection to ensure robust decision-making.
Servo Control:
- Control servo motors (servo1 and servo2) based on decision outcomes to move segregation bins.
- Map decision results to servo positions (e.g., 0 degrees for non-recyclable waste, 180 degrees for recyclable waste).
- Implement smooth servo movements and position feedback mechanisms for accurate bin positioning.
- CODE
#include <Servo.h>
Servo servo1; // Servo motor for compartment 1
Servo servo2; // Servo motor for compartment 2
int trigPin = 2; // Ultrasonic sensor trigger pin
int echoPin = 3; // Ultrasonic sensor echo pin
int moisturePin = A0; // Soil moisture sensor pin
int thresholdMoisture = 500; // Threshold for organic waste detection
void setup() {
servo1.attach(9); // Attach servo1 to pin 9
servo2.attach(10); // Attach servo2 to pin 10
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
Serial.begin(9600);
}
void loop() {
// Measure distance using ultrasonic sensor
long duration, distance;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1; // Convert duration to distance in cm
// Read soil moisture sensor
int moistureValue = analogRead(moisturePin);
// Segregate waste based on distance and moisture level
if (distance < 20) { // Object detected within 20cm
if (moistureValue > thresholdMoisture) {
// Organic waste detected, open compartment 1
servo1.write(90); // Open compartment 1
delay(2000); // Wait for waste to be deposited
servo1.write(0); // Close compartment 1
} else {
// Inorganic waste detected, open compartment 2
servo2.write(90); // Open compartment 2
delay(2000); // Wait for waste to be deposited
servo2.write(0); // Close compartment 2
}
delay(1000); // Delay before next cycle
}
}
Testing and Calibration:
- Conduct initial testing to validate sensor readings, decision logic, and servo movements.
- Perform calibration procedures to fine-tune sensor thresholds, servo positions, and system responsiveness.
- Utilize testing environments with simulated waste scenarios to evaluate system performance