description:
A smart waste management system for canals and rivers helps keep our waterways clean. Imagine there’s a device floating in the water that acts like a smart robot. This robot uses special sensors called ultrasonic sensors to “see” any trash floating in the water. These sensors send out sound waves (like a bat uses to see in the dark) and can tell if there’s garbage by measuring how long it takes for the sound to bounce back.Once the ultrasonic sensor finds trash, the robot uses a servo motor to move a small arm or net to grab the trash. The servo motor acts like the robot’s muscles, moving the arm precisely to scoop up the garbage and place it in a container.This system works continuously to detect and collect trash, ensuring that canals and rivers stay clean without needing people to constantly monitor and clean them. It helps protect fish, plants, and other wildlife by keeping their homes clean and safe. Plus, it makes our environment more beautiful and healthier for everyone to enjoy. This smart technology is an excellent example of how we can use science and engineering to solve real-world problems and take care of our planet.
Components Needed
- Arduino Board (e.g., Arduino Uno) – 1 piece
- Ultrasonic Sensor (e.g., HC-SR04) – 1 piece
- Servo Motor (Sg90s) – 2 Pieces
- Breadboard and Jumper Wires [Male to Male X 12]
Connections
- Ultrasonic Sensor (HC-SR04):
- VCC to 5V on the Arduino.
- GND to GND on the Arduino.
- Trigger the digital pin 3 on the Arduino.
- Echo to the digital pin 2 on the Arduino.
- Servo Motors:
- Servo 1: Signal to digital pin 4 on the Arduino, VCC to 5V, and GND to GND.
- Servo 2: Signal to digital pin 5 on the Arduino, VCC to 5V, and GND to GND.
Source code:
#include <Servo.h>
// Define pins for ultrasonic sensor
const int trigPin = 3;
const int echoPin = 2;
// Define servo motors
Servo servo1;
Servo servo2;
// Define distance threshold
const int distanceThreshold = 20; // distance in cm
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Set up ultrasonic sensor pins
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
// Attach servos to pins
servo1.attach(4);
servo2.attach(5);
// Initialize servos to 0 degrees
servo1.write(0);
servo2.write(0);
}
void loop() {
// Measure distance
long duration, distance;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1; // Convert to cm
// Print distance for debugging
Serial.print("Distance: ");
Serial.println(distance);
// Check if distance is less than threshold
if (distance < distanceThreshold) {
// Move servos to 90 degrees
servo1.write(90);
delay(500); // Wait for servo to move
servo2.write(90);
delay(500); // Wait for servo to move
// Optional: Return servos to initial position
delay(1000); // Wait for 1 second
servo1.write(0);
servo2.write(0);
}
delay(100); // Small delay to prevent excessive readings
}
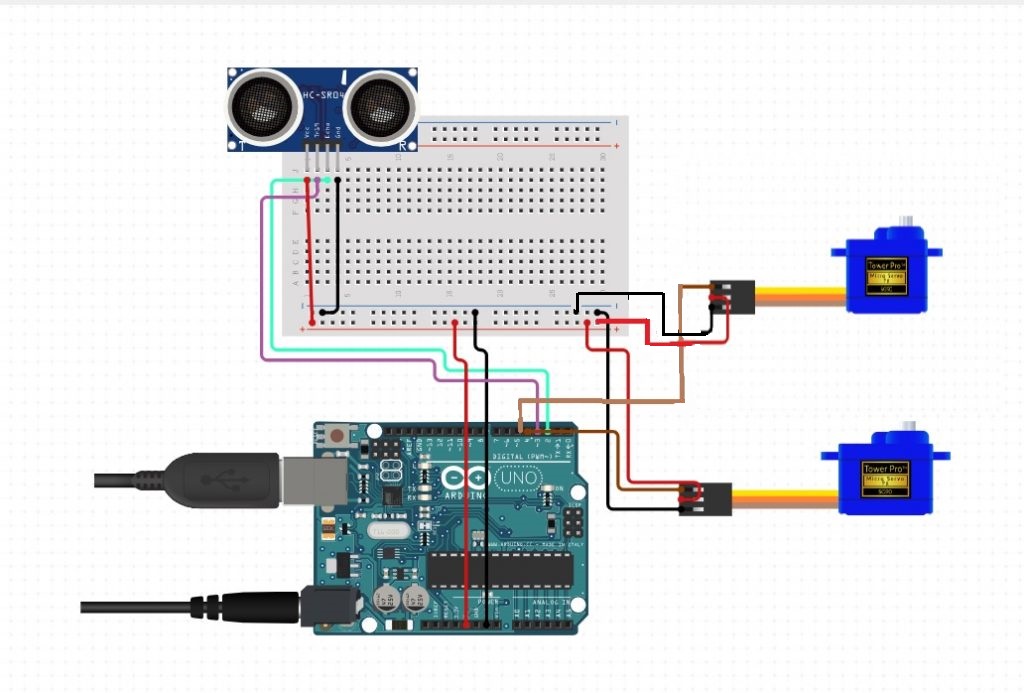