Description:
This project is designed to assist blind people by helping them identify obstacles and navigate stairs safely. The system uses an ultrasonic sensor, a vibro motor, and a buzzer to provide alerts. The ultrasonic sensor detects objects and changes in the environment, such as stairs, and the vibro motor and buzzer give tactile and auditory feedback to the user.
When the blind person walks, the ultrasonic sensor continuously measures the distance to objects in front of them. If an obstacle is detected within a certain range, the vibro motor is activated, causing it to vibrate. This vibration alerts the user to the presence of an obstacle, allowing them to take necessary action to avoid it. The vibration intensity can be adjusted to indicate how close the obstacle is, with stronger vibrations indicating closer proximity.
Additionally, the system helps with stair navigation. If the ultrasonic sensor detects that the ground level has dropped suddenly (indicating the presence of stairs), the buzzer is activated. The buzzer produces a beeping sound, alerting the user that they are approaching stairs. This auditory signal is crucial for blind people as it warns them to step carefully to avoid tripping or falling.
The components are simple and lightweight, making the system easy to integrate into a pair of shoes or a handheld device. The Arduino microcontroller processes the data from the ultrasonic sensor and controls the vibro motor and buzzer accordingly. The entire setup is powered by a small battery or adapter, ensuring portability.
Components Needed:
- Arduino Uno
- Ultrasonic Sensor (HC-SR04)
- Vibro Motor
- Buzzer
- Breadboard and jumper wires[ Male to Male X4, Male to Female X6]
- Adapter for powering the Arduino
Circuit Connections:
- Ultrasonic Sensor (HC-SR04):
- VCC to Arduino 5V
- GND to Arduino GND
- Trig to Arduino pin 9
- Echo to Arduino pin 10
- Vibro Motor:
- One terminal to Arduino pin 6
- The other terminal to Arduino GND
- Buzzer:
- Positive terminal to Arduino pin 7
- Negative terminal to Arduino GND
// Pin definitions
const int trigPin = 9;
const int echoPin = 10;
const int vibroMotorPin = 6;
const int buzzerPin = 7;
// Thresholds for obstacle and floor detection (in centimeters)
const int obstacleThreshold = 30; // Distance to trigger the vibro motor
const int floorThreshold = 10; // Minimum distance to detect the floor
void setup() {
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(vibroMotorPin, OUTPUT);
pinMode(buzzerPin, OUTPUT);
Serial.begin(9600);
}
void loop() {
// Read distance from ultrasonic sensor
long distance = getDistance();
Serial.print("Distance: ");
Serial.println(distance);
if (distance <= obstacleThreshold && distance > 0) {
// If an obstacle is near, vibrate the motor
digitalWrite(vibroMotorPin, HIGH);
} else {
// Turn off the vibro motor
digitalWrite(vibroMotorPin, LOW);
}
if (distance > floorThreshold || distance <= 0) {
// If the floor is not detected or out of range, beep the buzzer
digitalWrite(buzzerPin, HIGH);
} else {
// Turn off the buzzer
digitalWrite(buzzerPin, LOW);
}
delay(200); // Short delay before the next measurement
}
long getDistance() {
// Send a pulse from the ultrasonic sensor
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Measure the duration of the pulse
long duration = pulseIn(echoPin, HIGH);
// Calculate the distance in centimeters
long distance = (duration / 2) / 29.1;
return distance;
}
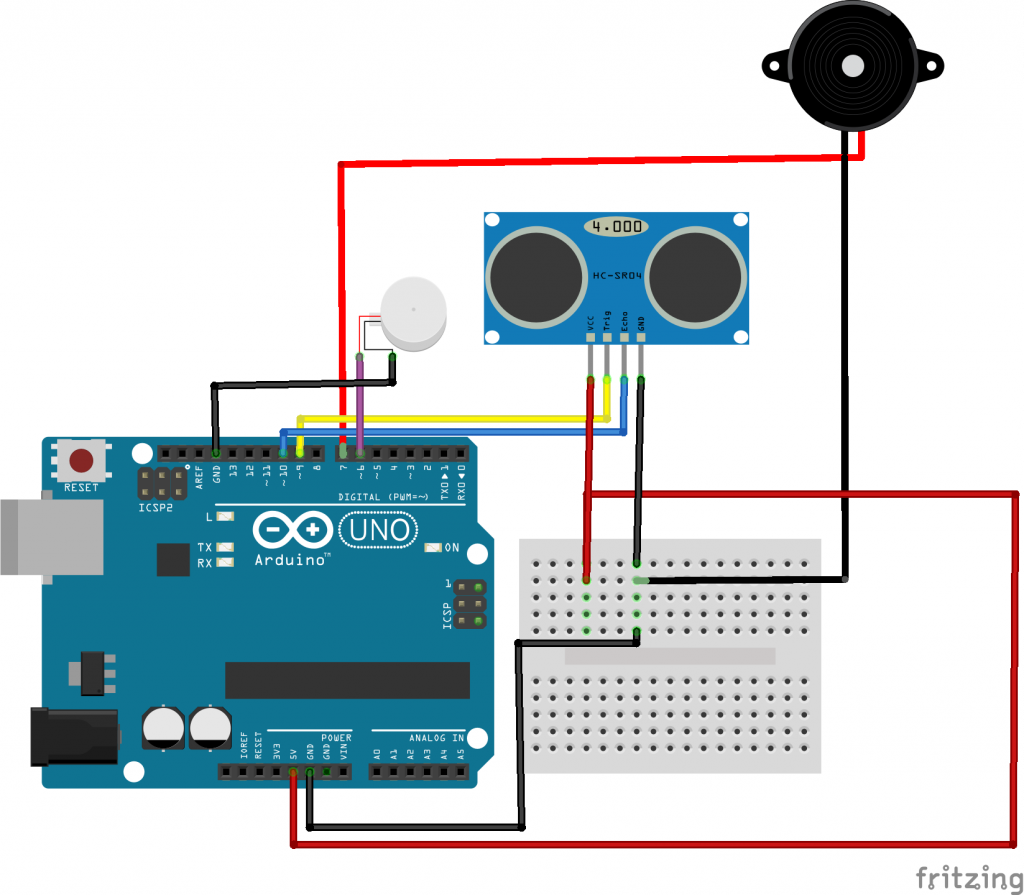