The Smart Door Lock is an innovative security solution designed to enhance the safety and convenience of your home or office. This advanced lock system integrates an Arduino Uno microcontroller, an I2C LCD display, a servo motor, and a keypad module to provide a seamless and secure access control system.
Key Features:
1. Arduino Uno Microcontroller:
- Central Processing Unit: The Arduino Uno acts as the brain of the smart door lock system, managing all inputs and outputs. It processes data from the keypad and controls the servo motor to lock or unlock the door.
2. I2C LCD Display:
- User Interface: The I2C LCD display provides a user-friendly interface for interacting with the lock system. It displays prompts for entering the access code, status messages, and alerts.
- Efficient Communication: Using I2C protocol for communication, it reduces the number of pins required, making the setup simpler and more efficient.
3. Servo Motor:
- Locking Mechanism: The servo motor is responsible for physically locking and unlocking the door. Controlled by the Arduino, it rotates to move the locking bolt into place or retract it.
- Precise Control: The servo motor allows for precise control over the lock mechanism, ensuring reliable operation.
4. Keypad Module:
- User Input: The keypad module is used for entering the access code. It allows users to input their unique PIN to unlock the door.
- Security: The use of a keypad enhances security by allowing only those with the correct code to gain access.
- How It Works:
- Initialization:
- When the system is powered on, the Arduino initializes the components, including the I2C LCD display and the servo motor.
- The LCD displays a welcome message and prompts the user to enter their access code.
- User Authentication:
- The user enters their access code using the keypad.
- The Arduino reads the input from the keypad and compares it to the stored access code.
- Access Granted/Denied:
- If the entered code is correct, the Arduino sends a signal to the servo motor to unlock the door and displays a success message on the LCD.
- If the entered code is incorrect, the LCD displays an error message and prompts the user to try again.
- Locking the Door:
- The door can be locked by either re-entering the access code or automatically after a set period.
- The Arduino sends a signal to the servo motor to lock the door, ensuring the security of the premises.
- Status Display:
- The LCD continuously displays the status of the door (locked or unlocked) and any error messages if the system detects an incorrect code or malfunction.
COMPONENTS REQUIRED:
- Arduino Uno
- I2C LCD
- Keypad
- Buzzer
- Servo motor
- Breadboard
- Male to Female jumper wires x 16
- Male to Male jumper wires x 10
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <Servo.h>
#include <Keypad.h>
// Initialize I2C LCD
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Initialize Servo Motor
Servo myServo;
// Define keypad pins and layout
const byte ROWS = 4;
const byte COLS = 4;
char keys[ROWS][COLS] = {
{'1','2','3','A'},
{'4','5','6','B'},
{'7','8','9','C'},
{'*','0','#','D'}
};
byte rowPins[ROWS] = {9, 8, 7, 6};
byte colPins[COLS] = {5, 4, 3, 2};
Keypad keypad = Keypad(makeKeymap(keys), rowPins, colPins, ROWS, COLS);
String correctCode = "1234"; // Example access code
String enteredCode = "";
void setup() {
// Set up LCD
lcd.init();
lcd.backlight();
lcd.setCursor(0, 0);
lcd.print("Smart Door Lock");
// Set up servo motor
myServo.attach(10); // Servo motor pin
lockDoor();
// Initial display message
lcd.setCursor(0, 1);
lcd.print("Enter Code:");
}
void loop() {
char key = keypad.getKey();
if (key) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Enter Code:");
enteredCode += key;
lcd.setCursor(0, 1);
lcd.print(enteredCode);
if (key == '#') {
if (enteredCode.substring(0, enteredCode.length()-1) == correctCode) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Access Granted");
unlockDoor();
delay(5000); // Door remains unlocked for 5 seconds
lockDoor();
} else {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Access Denied");
delay(2000);
}
enteredCode = ""; // Reset entered code
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Enter Code:");
}
}
}
void lockDoor() {
myServo.write(0); // Position servo to lock
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Door Locked");
}
void unlockDoor() {
myServo.write(90); // Position servo to unlock
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Door Unlocked");
}
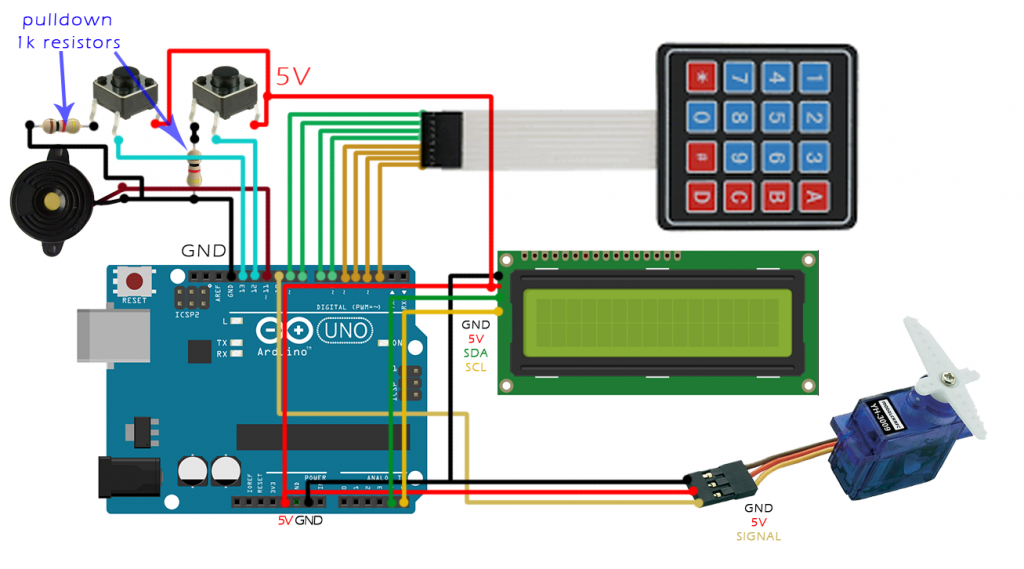