Smart Room device project uses infrared sensors to turn on the room light when someone enters and off when they leave. It also includes Bluetooth control: sending ‘a’ turns on an LED bulb, and ‘b’ turns it off. This setup makes it easy to manage lighting both automatically and remotely for convenience and energy savings.
Components:
- Arduino Uno – 1
- IR Sensors (e.g., PIR sensors) – 2
- Bluetooth Module (e.g., HC-05 or HC-06) – 1
- Relay Module – 1
- AC Bulb (or Lamp) – 1
- Jumper Wires – Several
- Breadboard – Optional
- Power Supply – For Arduino and relay
Connections:
Arduino to IR Sensors:
- IR Sensor 1:
- VCC to 5V
- GND to GND
- Signal pin to digital pin 2
- IR Sensor 2:
- VCC to 5V
- GND to GND
- Signal pin to digital pin 3
Arduino to Bluetooth Module (HC-05/HC-06):
- Bluetooth TX to Arduino RX (pin 0)
- Bluetooth RX to Arduino TX (pin 1)
- Bluetooth VCC to 5V
- Bluetooth GND to GND
Arduino to Relay Module:
- Relay IN to digital pin 13
- Relay VCC to 5V
- Relay GND to GND
Relay Module to AC Bulb:
- Common (COM) to one terminal of the bulb
- Normally Open (NO) to the live wire from the power source
- Other terminal of the bulb to neutral wire from the power source
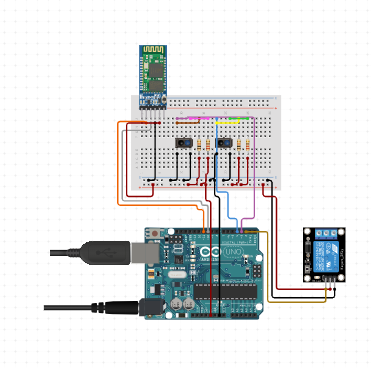
// Include SoftwareSerial library for Bluetooth communication
#include <SoftwareSerial.h>
// Define Bluetooth serial pins
#define bluetoothTx 11 // Arduino digital pin D1 to Bluetooth Rx
#define bluetoothRx 10 // Arduino digital pin D0 to Bluetooth Tx
// Initialize SoftwareSerial object
SoftwareSerial bluetooth(bluetoothTx, bluetoothRx);
// Define IR sensor pins
const int irSensor1 = 3; // IR sensor 1 connected to digital pin D2
const int irSensor2 = 4; // IR sensor 2 connected to digital pin D3
const int relayPin = 2; // Relay pin connected to digital pin D13
void setup() {
// Initialize serial communication for debugging
Serial.begin(9600);
// Set IR sensor pins as inputs
pinMode(irSensor1, INPUT);
pinMode(irSensor2, INPUT);
// Set relay pin as output
pinMode(relayPin, OUTPUT);
// Initialize Bluetooth communication
bluetooth.begin(9600);
}
void loop() {
// Check IR sensor inputs
bool personInside = digitalRead(irSensor1) || digitalRead(irSensor2);
// If person detected, turn on relay (and hence the bulb)
if (personInside) {
digitalWrite(relayPin, HIGH);
Serial.println("Light ON - Person detected!");
} else {
digitalWrite(relayPin, LOW);
Serial.println("Light OFF - No person detected.");
}
// Check for Bluetooth commands
if (bluetooth.available() > 0) {
char command = bluetooth.read();
if (command == 'a') {
digitalWrite(relayPin, HIGH); // Turn relay ON (bulb ON)
Serial.println("Bulb ON - Bluetooth command 'a'");
} else if (command == 'b') {
digitalWrite(relayPin, LOW); // Turn relay OFF (bulb OFF)
Serial.println("Bulb OFF - Bluetooth command 'b'");
}
}
delay(100); // Delay to stabilize readings and prevent rapid serial input checking
}