This project aims to make a smart home safety system for the kitchen to detect LPG gas leaks and prevent explosions. It uses an Arduino Uno, an MQ-2 gas sensor, a relay module, an exhaust fan, and a buzzer. When the system detects a gas leak, it automatically turns on the buzzer and the exhaust fan to keep the area safe by letting out the gas.
Components
- Arduino Uno
- MQ-2 Gas Sensor
- Relay Module
- Exhaust Fan
- Buzzer
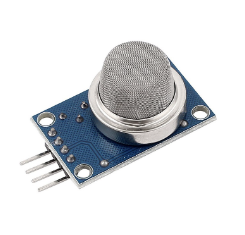
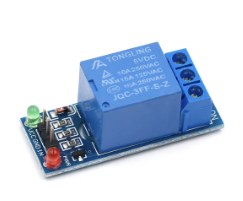
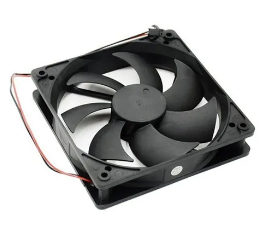
Circuit Connections
- MQ-2 Gas Sensor: Connect VCC to 5V, GND to GND, and A0 (analog output) to an analog pin (e.g., A3) on the Arduino.
- Relay Module: Connect the control signal pin to a digital pin (e.g., D3) on the Arduino, VCC to 5V, and GND to GND.
- Exhaust Fan: Connect to the relay module.
- Buzzer: Connect positive to a digital pin (e.g., D2) and negative to GND.
Circuit diagram is given below:
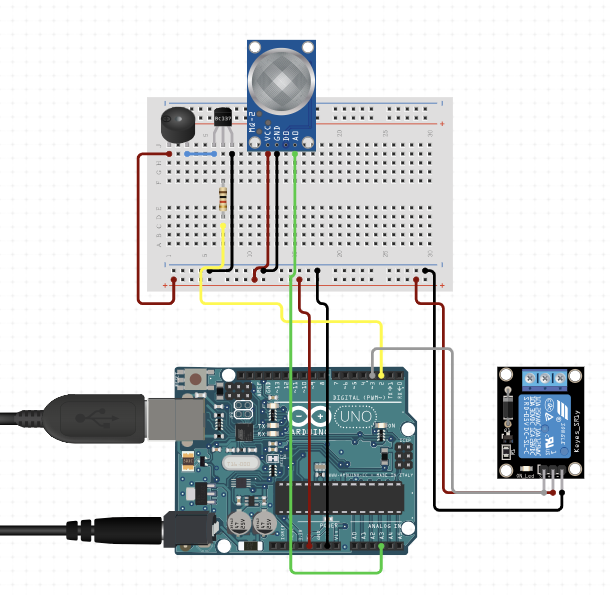
The code is given below:
// Define pins
const int gasSensorPin = A3; // Analog pin for MQ-2 gas sensor
const int relayPin = 3; // Digital pin for Relay Module
const int buzzerPin = 2; // Digital pin for Buzzer
// Threshold value for gas detection
const int gasThreshold = 300; // Adjust based on calibration
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Set pin modes
pinMode(gasSensorPin, INPUT);
pinMode(relayPin, OUTPUT);
pinMode(buzzerPin, OUTPUT);
// Ensure the fan and buzzer are off initially
digitalWrite(relayPin, LOW);
digitalWrite(buzzerPin, LOW);
}
void loop() {
// Read the gas sensor value
int gasValue = analogRead(gasSensorPin);
Serial.print("Gas Sensor Value: ");
Serial.println(gasValue);
// Check if gas value exceeds the threshold
if (gasValue > gasThreshold) {
// Gas leak detected, activate buzzer and exhaust fan
digitalWrite(relayPin, HIGH); // Turn on exhaust fan
digitalWrite(buzzerPin, HIGH); // Turn on buzzer
} else {
// No gas leak, turn off buzzer and exhaust fan
digitalWrite(relayPin, LOW); // Turn off exhaust fan
digitalWrite(buzzerPin, LOW); // Turn off buzzer
}
// Short delay before next reading
delay(1000);
}
Benefits
- Automatic Gas Leak Detection: Ensures quick response to gas leaks, reducing the risk of explosion.
- Ventilation System: Automatically activates to expel leaked gas, improving safety.
- Audible Alert: The buzzer provides an immediate warning to occupants, ensuring they can take further action if needed.
Application:
1. Residential Homes
- Kitchens: Provides an essential safety measure for households using LPG for cooking, ensuring immediate detection and mitigation of gas leaks.
- Utility Rooms: Monitors areas where gas appliances like water heaters or dryers are located, enhancing overall home safety.
2. Commercial Kitchens
- Restaurants and Cafes: Ensures the safety of commercial cooking environments where large amounts of LPG are used, protecting both staff and customers.
- Catering Services: Enhances safety in mobile kitchens used by catering companies, preventing gas leaks during operations.
3. Industrial Settings
- Manufacturing Plants: Monitors for gas leaks in facilities where LPG is used in processes, reducing the risk of industrial accidents.
- Warehouses: Protects storage areas where LPG cylinders are kept, ensuring any leaks are quickly addressed.
4. Educational Institutions
- School and University Laboratories: Ensures safety in laboratories where LPG is used for experiments, protecting students and staff.
- Cafeterias: Provides an additional safety layer in school and university cafeterias using LPG for cooking.
5. Healthcare Facilities
- Hospitals and Clinics: Protects areas where LPG is used for medical equipment or in kitchens, ensuring the safety of patients and staff.
- Nursing Homes: Ensures a safe cooking environment, protecting elderly residents from the dangers of gas leaks.