The Smart Handicapped Car project combines innovative technology such as the L298N motor driver, dual ultrasonic sensors, Arduino microcontrollers, and a DC motor. These components work together to detect obstacles and ensure safe navigation for disabled individuals. By stopping the car upon detecting obstacles, it guarantees safety and independence in mobility. This project introduces students to the integration of sensors and motor control systems, providing a clear and accessible way to understand how intelligent vehicles are designed and operate effectively in real-world scenarios.
Components/Circuit:
- L298N Motor Driver: Controls DC motor speed and direction.
- Ultrasonic Sensors: Detect obstacles for safe navigation.
- Arduino Microcontroller: Processes sensor data and controls the system.
- DC Motor: Propels the car and aids in mobility.
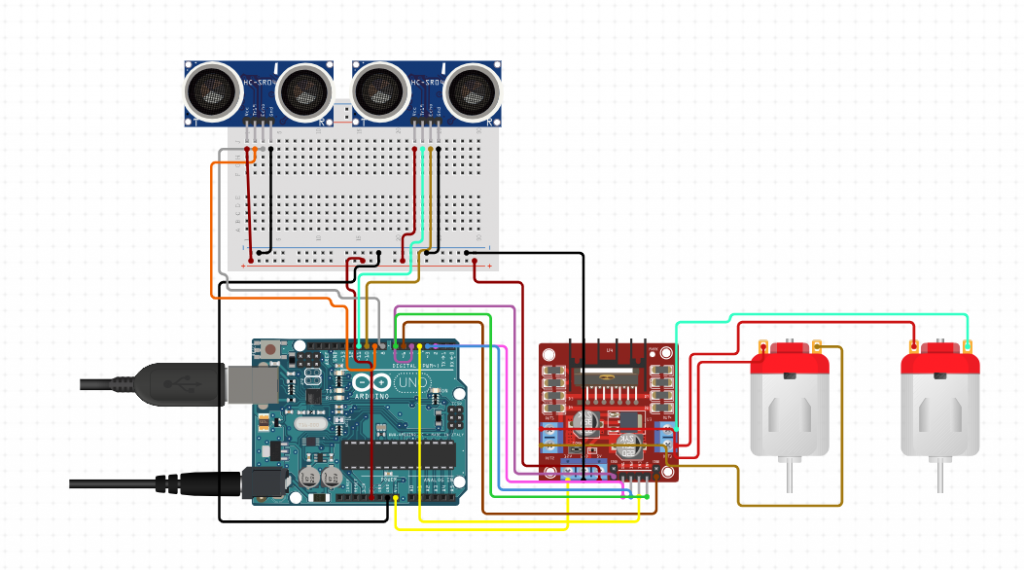
CODE
#include <NewPing.h>
#define TRIGGER_PIN_1 9 // Trig pin of first ultrasonic sensor
#define ECHO_PIN_1 8 // Echo pin of first ultrasonic sensor
#define TRIGGER_PIN_2 11 // Trig pin of second ultrasonic sensor
#define ECHO_PIN_2 10 // Echo pin of second ultrasonic sensor
#define MAX_DISTANCE 200 // Maximum distance for ultrasonic sensor
NewPing sonar1(TRIGGER_PIN_1, ECHO_PIN_1, MAX_DISTANCE);
NewPing sonar2(TRIGGER_PIN_2, ECHO_PIN_2, MAX_DISTANCE);
// Define motor driver pins
int enA = 5; // Motor 1 speed control pin (ENA)
int in1 = 2; // Motor 1 direction control pin (IN1)
int in2 = 3; // Motor 1 direction control pin (IN2)
int enB = 6; // Motor 2 speed control pin (ENB)
int in3 = 4; // Motor 2 direction control pin (IN3)
int in4 = 7; // Motor 2 direction control pin (IN4)
void setup() {
pinMode(enA, OUTPUT);
pinMode(in1, OUTPUT);
pinMode(in2, OUTPUT);
pinMode(enB, OUTPUT);
pinMode(in3, OUTPUT);
pinMode(in4, OUTPUT);
Serial.begin(9600);
}
void loop() {
int distance1 = sonar1.ping_cm();
int distance2 = sonar2.ping_cm();
Serial.print("Distance 1: ");
Serial.print(distance1);
Serial.print(" cm \t Distance 2: ");
Serial.print(distance2);
Serial.println(" cm");
if (distance1 <= 20) { // Adjust threshold distance as needed
stopMotors();
delay(1000); // Stop for 1 second
} else {
moveForward();
}
delay(100); // Adjust delay based on your requirements
}
void stopMotors() {
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
analogWrite(enA, 0); // Stop Motor 1
digitalWrite(in3, LOW);
digitalWrite(in4, LOW);
analogWrite(enB, 0); // Stop Motor 2
}
void moveForward() {
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
analogWrite(enA, 255); // Motor 1 forward
digitalWrite(in3, HIGH);
digitalWrite(in4, LOW);
analogWrite(enB, 255); // Motor 2 forward
}
How it Works:
- Obstacle Detection: Ultrasonic sensors detect obstacles in the car’s path.
- Motor Control: The L298N motor driver halts the car upon obstacle detection and controls the DC motor for propulsion.
- Safety Assurance: Ensures safe navigation and independence for disabled individuals.
Benefits:
- Enhanced Safety: Halts the car upon detecting obstacles, preventing accidents.
- Accessibility: Provides an accessible solution for disabled individuals to navigate safely.
- Independent Mobility: Enables safe and independent mobility for handicapped individuals.
- Sensor-Motor Integration: Demonstrates the integration of sensors and motor control for intelligent vehicle systems.
Application:
The Smart Handicapped Car is ideal for providing safe and accessible mobility solutions for disabled individuals. It showcases the potential of sensor technology and motor control integration in creating intelligent, obstacle-aware vehicles tailored for specific needs in transportation and accessibility.