The Smart E-Book project is an interactive and educational tool designed to display information using an Arduino Uno, five push buttons, and an I2C LCD. This project aims to provide a versatile and engaging way to access information or educational content at the press of a button. The Arduino Uno serves as the project’s microcontroller, orchestrating the inputs from the push buttons and controlling the I2C LCD display. Each of the five push buttons is assigned a specific function, allowing users to navigate through different sections of the e-book or access various pieces of information. The I2C LCD, typically a 16×2 display, is used to present the information in a clear and readable format. For instance, pressing Button 1 might display a welcome message or the table of contents, Button 2 could show information about a particular topic, Button 3 might display examples or exercises, Button 4 could be used for displaying additional resources or references, and Button 5 could provide a summary or conclusion. This setup allows users to easily navigate and access the content they need. This project not only demonstrates the capabilities of the Arduino in handling multiple inputs and outputs but also serves as a practical application for creating interactive educational tools. The Smart E-Book is perfect for use in classrooms, libraries, and personal learning environments, making information accessible and engaging through simple, interactive controls. |
Arduino Uno
Five push buttons
I2C 16×2 LCD
Breadboard and jumper wires – 4x M2F , 12 X M2M.
Resistors (for debouncing the push buttons)
Power supply or USB cable
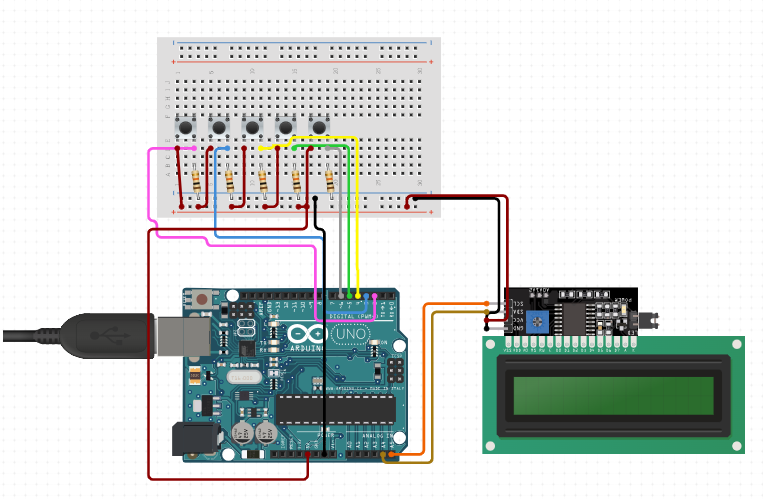
// Include Libraries
#include "Arduino.h"
#include "LiquidCrystal_PCF8574.h"
#include "Button.h"
// Pin Definitions
#define PUSHBUTTON_1_PIN_2 2
#define PUSHBUTTON_2_PIN_2 3
#define PUSHBUTTON_3_PIN_2 4
#define PUSHBUTTON_4_PIN_2 5
#define PUSHBUTTON_5_PIN_2 6
// Global variables and defines
// There are several different versions of the LCD I2C adapter, each might have a different address.
// Try the given addresses by Un/commenting the following rows until LCD works follow the serial monitor prints.
// To find your LCD address go to: http://playground.arduino.cc/Main/I2cScanner and run example.
#define LCD_ADDRESS 0x3F
//#define LCD_ADDRESS 0x27
// Define LCD characteristics
#define LCD_ROWS 2
#define LCD_COLUMNS 16
#define SCROLL_DELAY 150
#define BACKLIGHT 255
// object initialization
LiquidCrystal_PCF8574 lcdI2C;
Button pushButton_1(PUSHBUTTON_1_PIN_2);
Button pushButton_2(PUSHBUTTON_2_PIN_2);
Button pushButton_3(PUSHBUTTON_3_PIN_2);
Button pushButton_4(PUSHBUTTON_4_PIN_2);
Button pushButton_5(PUSHBUTTON_5_PIN_2);
// define vars for testing menu
const int timeout = 10000; //define timeout of 10 sec
char menuOption = 0;
long time0;
// Setup the essentials for your circuit to work. It runs first every time your circuit is powered with electricity.
void setup()
{
// Setup Serial which is useful for debugging
// Use the Serial Monitor to view printed messages
Serial.begin(9600);
while (!Serial) ; // wait for serial port to connect. Needed for native USB
Serial.println("start");
// initialize the lcd
lcdI2C.begin(LCD_COLUMNS, LCD_ROWS, LCD_ADDRESS, BACKLIGHT);
pushButton_1.init();
pushButton_2.init();
pushButton_3.init();
pushButton_4.init();
pushButton_5.init();
menuOption = menu();
}
// Main logic of your circuit. It defines the interaction between the components you selected. After setup, it runs over and over again, in an eternal loop.
void loop()
{
if(menuOption == '1') {
// LCD 16x2 I2C - Test Code
// The LCD Screen will display the text of your choice.
lcdI2C.clear(); // Clear LCD screen.
lcdI2C.print(" Circuito.io "); // Print print String to LCD on first line
lcdI2C.selectLine(2); // Set cursor at the begining of line 2
lcdI2C.print(" Rocks! "); // Print print String to LCD on second line
delay(1000);
}
else if(menuOption == '2') {
// Mini Pushbutton Switch #1 - Test Code
//Read pushbutton state.
//if button is pressed function will return HIGH (1). if not function will return LOW (0).
//for debounce funtionality try also pushButton_1.onPress(), .onRelease() and .onChange().
//if debounce is not working properly try changing 'debounceDelay' variable in Button.h
bool pushButton_1Val = pushButton_1.read();
Serial.print(F("Val: ")); Serial.println(pushButton_1Val);
}
else if(menuOption == '3') {
// Mini Pushbutton Switch #2 - Test Code
//Read pushbutton state.
//if button is pressed function will return HIGH (1). if not function will return LOW (0).
//for debounce funtionality try also pushButton_2.onPress(), .onRelease() and .onChange().
//if debounce is not working properly try changing 'debounceDelay' variable in Button.h
bool pushButton_2Val = pushButton_2.read();
Serial.print(F("Val: ")); Serial.println(pushButton_2Val);
}
else if(menuOption == '4') {
// Mini Pushbutton Switch #3 - Test Code
//Read pushbutton state.
//if button is pressed function will return HIGH (1). if not function will return LOW (0).
//for debounce funtionality try also pushButton_3.onPress(), .onRelease() and .onChange().
//if debounce is not working properly try changing 'debounceDelay' variable in Button.h
bool pushButton_3Val = pushButton_3.read();
Serial.print(F("Val: ")); Serial.println(pushButton_3Val);
}
else if(menuOption == '5') {
// Mini Pushbutton Switch #4 - Test Code
//Read pushbutton state.
//if button is pressed function will return HIGH (1). if not function will return LOW (0).
//for debounce funtionality try also pushButton_4.onPress(), .onRelease() and .onChange().
//if debounce is not working properly try changing 'debounceDelay' variable in Button.h
bool pushButton_4Val = pushButton_4.read();
Serial.print(F("Val: ")); Serial.println(pushButton_4Val);
}
else if(menuOption == '6') {
// Mini Pushbutton Switch #5 - Test Code
//Read pushbutton state.
//if button is pressed function will return HIGH (1). if not function will return LOW (0).
//for debounce funtionality try also pushButton_5.onPress(), .onRelease() and .onChange().
//if debounce is not working properly try changing 'debounceDelay' variable in Button.h
bool pushButton_5Val = pushButton_5.read();
Serial.print(F("Val: ")); Serial.println(pushButton_5Val);
}
if (millis() - time0 > timeout)
{
menuOption = menu();
}
}
// Menu function for selecting the components to be tested
// Follow serial monitor for instrcutions
char menu()
{
Serial.println(F("\nWhich component would you like to test?"));
Serial.println(F("(1) LCD 16x2 I2C"));
Serial.println(F("(2) Mini Pushbutton Switch #1"));
Serial.println(F("(3) Mini Pushbutton Switch #2"));
Serial.println(F("(4) Mini Pushbutton Switch #3"));
Serial.println(F("(5) Mini Pushbutton Switch #4"));
Serial.println(F("(6) Mini Pushbutton Switch #5"));
Serial.println(F("(menu) send anything else or press on board reset button\n"));
while (!Serial.available());
// Read data from serial monitor if received
while (Serial.available())
{
char c = Serial.read();
if (isAlphaNumeric(c))
{
if(c == '1')
Serial.println(F("Now Testing LCD 16x2 I2C"));
else if(c == '2')
Serial.println(F("Now Testing Mini Pushbutton Switch #1"));
else if(c == '3')
Serial.println(F("Now Testing Mini Pushbutton Switch #2"));
else if(c == '4')
Serial.println(F("Now Testing Mini Pushbutton Switch #3"));
else if(c == '5')
Serial.println(F("Now Testing Mini Pushbutton Switch #4"));
else if(c == '6')
Serial.println(F("Now Testing Mini Pushbutton Switch #5"));
else
{
Serial.println(F("illegal input!"));
return 0;
}
time0 = millis();
return c;
}
}
}