Description:
In this project, students have developed an innovative system designed to enhance road safety by restricting underage individuals from driving and preventing drunk driving. The system employs RFID technology to verify the identity and age of the driver. When a driver enters the vehicle, they must scan their RFID card. The system checks if the card belongs to an authorized, age-appropriate individual.
In addition to verifying age, the system includes an alcohol sensor that continuously monitors the driver’s breath for alcohol. If the sensor detects alcohol above a safe threshold, the system immediately alerts the driver and prevents the vehicle from starting. This dual-check mechanism ensures that only authorized, sober drivers can operate the vehicle.
A touch sensor is also integrated to ensure that the driver is ready and in control before the car starts moving. An I2C LCD display provides real-time feedback to the driver, indicating whether they are authorized, sober, and ready to drive. LED indicators and a buzzer provide additional alerts for unauthorized or drunk driving attempts.
This smart car driving system not only promotes responsible driving behavior but also significantly reduces the risks associated with underage and drunk driving, thereby contributing to safer roads and communities.
Components and Connections
- RFID Module (MFRC522):
- SDA (SS) to Arduino pin A1
- SCK to Arduino pin 13
- MOSI to Arduino pin 11
- MISO to Arduino pin 12
- RST to Arduino pin 10
- 3.3V to 3.3V
- GND to GND
- I2C LCD Display:
- SDA to Arduino A4
- SCL to Arduino A5
- VCC to 5V
- GND to GND
- Motor Driver (L298N):
- IN1 to Arduino pin 3
- IN2 to Arduino pin 4
- ENA to Arduino pin 5
- IN3 to Arduino pin 7
- IN4 to Arduino pin 8
- ENB to Arduino pin 6
- OUT1 and OUT2 to the motor leads
- VCC to external power supply
- GND to GND
- 12V to external power supply (if needed for the motor)
- Alcohol Sensor (e.g., MQ-3):
- AO to Arduino A3
- VCC to 5V
- GND to GND
- Touch Sensor:
- Output to Arduino pin 2
- VCC to 5V
- GND to GND
- LED:
- Positive to Arduino pin 9
- Negative to GND
- RESISTOR: [220 Ohm]
- WIRE:[Male to Male X14, Male to Female X8 ]
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <MFRC522.h>
#include <Servo.h>
// Define RFID pins
#define SS_PIN A1
#define RST_PIN 10
// Initialize RFID and LCD
MFRC522 rfid(SS_PIN, RST_PIN);
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Define pins
const int greenLED = 9;
const int touchSensor = 2;
const int alcoholSensor = A3;
const int motorPin1 = 3;
const int motorPin2 = 4;
const int ena = 5;
const int motorPin3 = 7;
const int motorPin4 = 8;
const int enb = 6;
// Authorized RFID tag UID
byte authorizedUID[] = {0xDE, 0xAD, 0xBE, 0xEF};
void setup() {
// Initialize serial communication
Serial.begin(9600);
SPI.begin();
rfid.PCD_Init();
lcd.init();
lcd.backlight();
// Set pin modes
pinMode(greenLED, OUTPUT);
pinMode(touchSensor, INPUT);
pinMode(alcoholSensor, INPUT);
pinMode(motorPin1, OUTPUT);
pinMode(motorPin2, OUTPUT);
pinMode(ena, OUTPUT);
pinMode(motorPin3, OUTPUT);
pinMode(motorPin4, OUTPUT);
pinMode(enb, OUTPUT);
// Initialize motors to off
digitalWrite(motorPin1, LOW);
digitalWrite(motorPin2, LOW);
digitalWrite(motorPin3, LOW);
digitalWrite(motorPin4, LOW);
analogWrite(ena, 0);
analogWrite(enb, 0);
}
void loop() {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Scan RFID");
if (!rfid.PICC_IsNewCardPresent() || !rfid.PICC_ReadCardSerial()) {
return;
}
if (isAuthorized(rfid.uid.uidByte, rfid.uid.size)) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Authorized");
int alcoholLevel = analogRead(alcoholSensor);
if (alcoholLevel < 200) { // Adjust threshold based on your sensor
digitalWrite(greenLED, HIGH);
lcd.setCursor(0, 1);
lcd.print("Get Ready");
delay(2000); // Wait for 2 seconds
if (digitalRead(touchSensor) == HIGH) {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Driving Mode");
startMotor();
} else {
lcd.clear();
lcd.setCursor(0, 1);
lcd.print("Touch to Start");
digitalWrite(greenLED, LOW);
stopMotor();
}
} else {
digitalWrite(greenLED, LOW);
lcd.setCursor(0, 1);
lcd.print("Driver Drunk");
stopMotor();
}
} else {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Unauthorized");
digitalWrite(greenLED, LOW);
stopMotor();
}
delay(1000);
rfid.PICC_HaltA();
rfid.PCD_StopCrypto1();
}
bool isAuthorized(byte *uid, byte uidSize) {
if (uidSize != 4) {
return false;
}
for (byte i = 0; i < uidSize; i++) {
if (uid[i] != authorizedUID[i]) {
return false;
}
}
return true;
}
void startMotor() {
analogWrite(ena, 255); // Set speed
analogWrite(enb, 255); // Set speed
digitalWrite(motorPin1, HIGH);
digitalWrite(motorPin2, LOW);
digitalWrite(motorPin3, HIGH);
digitalWrite(motorPin4, LOW);
}
void stopMotor() {
digitalWrite(motorPin1, LOW);
digitalWrite(motorPin2, LOW);
digitalWrite(motorPin3, LOW);
digitalWrite(motorPin4, LOW);
analogWrite(ena, 0);
analogWrite(enb, 0);
}
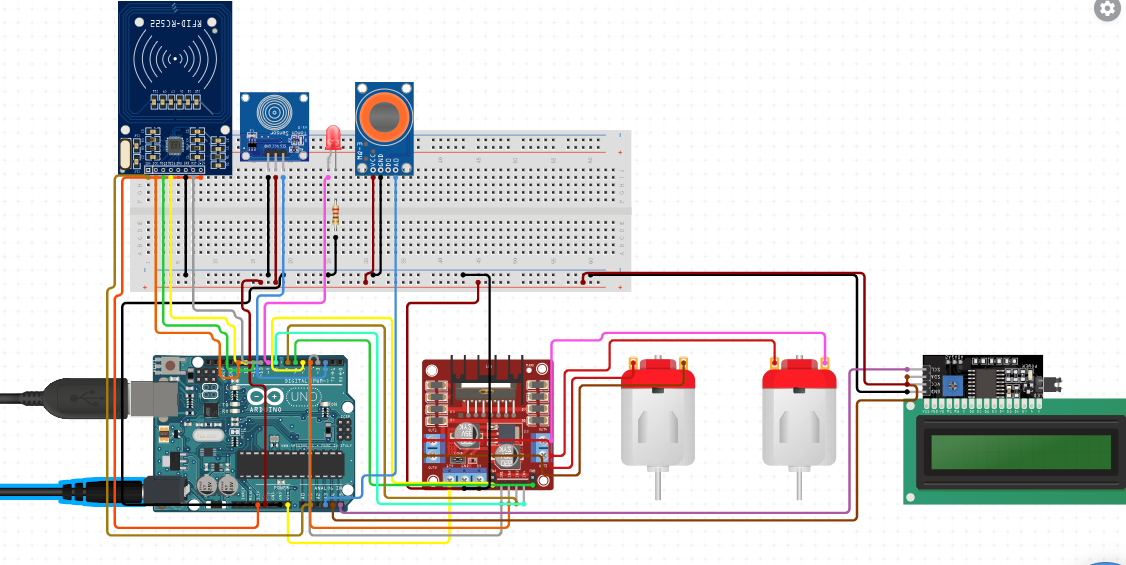