The Smart Bridge project combines cutting-edge technology such as piezoelectric sensors, light-dependent resistors (LDR), RGB LEDs, servo motors, and Arduino microcontrollers. These components work together to improve road safety and manage traffic effectively. For students, this means creating a bridge system that can detect heavy vehicles precisely and respond with traffic control strategies. By doing so, the project reduces the chances of accidents caused by heavy traffic and keeps the bridge operations running smoothly and efficiently.
Components/Circuit:
- Piezoelectric Sensors: Detect pressure from heavy vehicles.
- LDR: Adjusts operations based on ambient light levels.
- RGB LED: Indicates traffic signals for efficient traffic management.
- Servo Motor: Controls barriers or mechanisms for traffic control.
- Arduino Board: Processes sensor data and controls the system.
- Resistor (220 ohms): Used to limit current and protect components in the circuit.
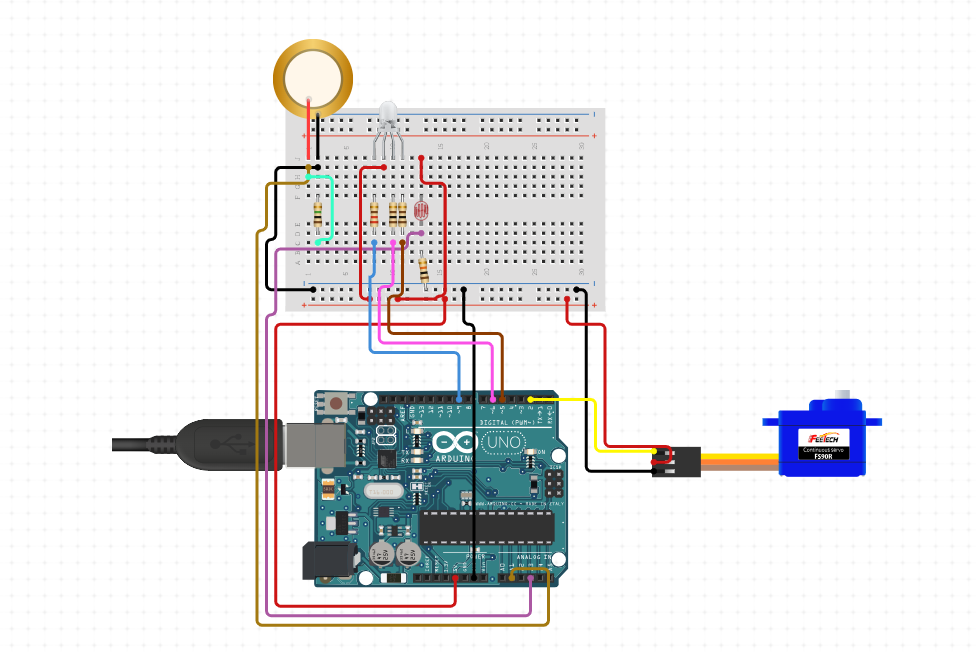
CODE
// Define pin connections
const int piezoPin = A1; // Piezoelectric sensor analog pin
const int ldrPin = A3; // LDR analog pin (changed to A3)
const int redPin = 9; // Red pin of RGB LED
const int greenPin = 6; // Green pin of RGB LED
const int bluePin = 5; // Blue pin of RGB LED
const int servoPin = 2; // Servo motor control pin
// Include necessary libraries
#include <Servo.h>
// Create servo object
Servo myServo;
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Attach servo to its pin
myServo.attach(servoPin);
// Set RGB LED pins as outputs
pinMode(redPin, OUTPUT);
pinMode(greenPin, OUTPUT);
pinMode(bluePin, OUTPUT);
}
void loop() {
// Read analog values from piezoelectric sensor and LDR
int piezoValue = analogRead(piezoPin);
int ldrValue = analogRead(ldrPin);
// Print sensor values for debugging
Serial.print("Piezo Value: ");
Serial.print(piezoValue);
Serial.print("\t LDR Value: ");
Serial.println(ldrValue);
// Check if piezo value exceeds threshold for vehicle detection
if (piezoValue > 500) {
// Vehicle detected, stop the vehicle using servo motor
stopVehicle();
// Activate red LED to indicate stopped vehicle
digitalWrite(redPin, HIGH);
digitalWrite(greenPin, LOW);
digitalWrite(bluePin, LOW);
} else {
// No vehicle detected, allow normal traffic flow
digitalWrite(redPin, LOW);
digitalWrite(greenPin, HIGH);
digitalWrite(bluePin, LOW);
}
// Check ambient light level using LDR
if (ldrValue < 500) {
// Daytime operation, turn off RGB LED
digitalWrite(redPin, LOW);
digitalWrite(greenPin, LOW);
digitalWrite(bluePin, LOW);
}
// Delay for stability
delay(1000);
}
void stopVehicle() {
// Move servo to a position to stop the vehicle
myServo.write(90); // Adjust the angle as needed
// Print message for debugging
Serial.println("Vehicle Detected! Stopping Vehicle...");
// Add additional actions as needed
// Delay to maintain servo position
delay(5000); // Delay for 5 seconds
// Reset servo position
myServo.write(0); // Move servo back to initial position
// Print message for debugging
Serial.println("Vehicle Released.");
}
How it Works:
- Heavy Vehicle Detection: Piezoelectric sensors detect heavy vehicles passing over the bridge.
- Traffic Light Control: RGB LED signals traffic lights based on detected vehicle types and traffic conditions.
- Barrier Control: The Servo motor operates barriers to manage vehicle flow and safety.
Benefits:
- Road Safety Enhancement: Prevents accidents by detecting heavy vehicles and controlling traffic flow.
- Efficient Traffic Management: Adjusts traffic signals and barriers based on real-time data.
- Environmental Adaptation: Responds to ambient light levels for energy-efficient operations.
- Cost-Effective Solution: Utilizes affordable components for effective bridge management.
- Intelligent Infrastructure: Demonstrates the potential of integrating sensors and control systems for smart infrastructure.
Application:
The Smart Bridge system enhances road safety, manages heavy vehicle traffic, and showcases intelligent infrastructure solutions. It can be used in bridge management systems, smart city projects, and transportation infrastructure development to create safer, more efficient roadways for all users.