The Sewer Gas Detector and Combustor System is a crucial safety measure aimed at monitoring and controlling dangerous gases such as methane (CH4) and hydrogen sulfide (H2S) within sewer systems or enclosed spaces. It integrates gas sensors to detect these hazardous gases, an alarm system to alert about dangerous levels, and a combustor unit to safely eliminate the gases, thus ensuring environmental safety and mitigating the risk of explosions or health hazards. This system is essential for maintaining a safe working environment and preventing potential accidents, making it an important topic for students to understand in environmental and safety studies.
Components:
- Arduino Uno: Central microcontroller for data processing and control.
- I2C LCD Display: Displays real-time data including air quality, temperature, and humidity.
- MQ-4 Gas Sensor: Detects methane (CH4) gas.
- MQ-2 Gas Sensor: Detects flammable gases and smoke.MQ-7 Gas Sensor: Detects carbon monoxide (CO) gas.
- MQ-135 Gas Sensor: Detects a variety of harmful gases including ammonia (NH3), nitrogen oxides (NOx), alcohol, benzene, smoke, and carbon dioxide (CO2).
- Breadboard: A reusable platform for building and testing circuits without soldering, ideal for prototyping and adjustments.
Setup and Installation:
- Install MQ-4 and MQ-136 gas sensors strategically in sewer systems or confined spaces to continuously monitor gas levels.
- Connect gas sensors to an alarm system for real-time notifications.
- Incorporate a combustor unit to neutralize hazardous gases and prevent their accumulation.
Operation/Circuit :
- MQ-4 and MQ-136 gas sensors continuously monitor methane (CH4) and hydrogen sulfide (H2S) levels.
- Upon detecting unsafe levels, gas sensors trigger the alarm system to alert operators or residents.
- The combustor unit activates to safely burn off hazardous gases, minimizing risks and ensuring environmental safety.
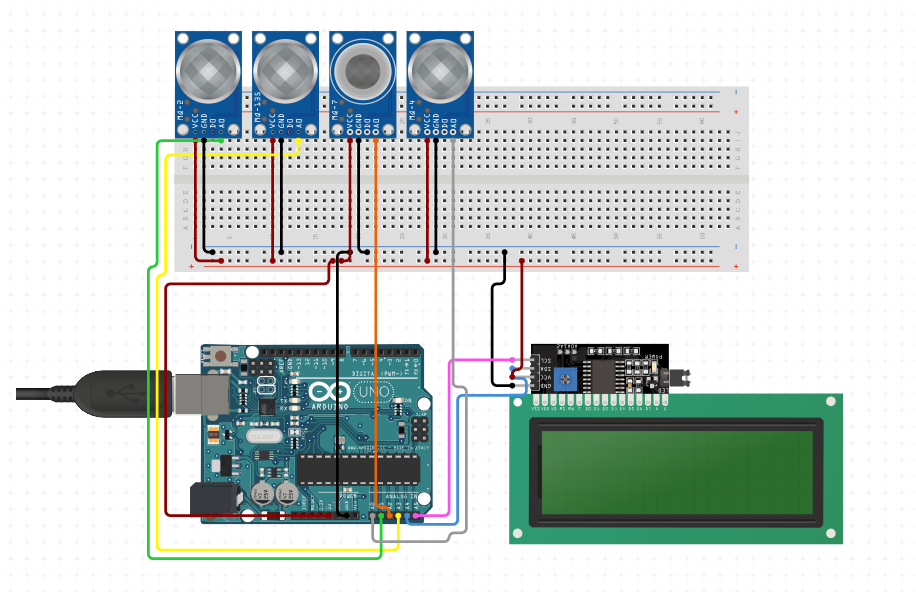
CODE
// Include the necessary libraries
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Define the I2C address and the LCD dimensions
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Define the analog input pins for each sensor
const int methanePin = A0;
const int airQualityPin = A1;
const int smokeLpgCOPin = A2;
const int coLevelPin = A3;
// Define threshold values for each sensor
const int methaneThreshold = 500; // Example threshold for methane
const int airQualityThreshold = 300; // Example threshold for air quality
const int smokeLpgCOThreshold = 400; // Example threshold for smoke/LPG/CO
const int coLevelThreshold = 200; // Example threshold for CO level
void setup() {
// Initialize the LCD
lcd.begin();
lcd.backlight();
lcd.setCursor(0, 0);
lcd.print("Gas Sensors:");
// Initialize serial communication for debugging
Serial.begin(9600);
}
void loop() {
// Read the analog values from the sensors
int methaneValue = analogRead(methanePin);
int airQualityValue = analogRead(airQualityPin);
int smokeLpgCOValue = analogRead(smokeLpgCOPin);
int coLevelValue = analogRead(coLevelPin);
// Display the sensor readings on the LCD
lcd.setCursor(0, 1);
lcd.print("Methane: ");
lcd.print(methaneValue);
lcd.print(" ");
lcd.print(methaneValue > methaneThreshold ? "HIGH" : "LOW");
lcd.setCursor(0, 2);
lcd.print("Air Qual: ");
lcd.print(airQualityValue);
lcd.print(" ");
lcd.print(airQualityValue > airQualityThreshold ? "HIGH" : "LOW");
lcd.setCursor(0, 3);
lcd.print("Smoke/LPG: ");
lcd.print(smokeLpgCOValue);
lcd.print(" ");
lcd.print(smokeLpgCOValue > smokeLpgCOThreshold ? "HIGH" : "LOW");
lcd.setCursor(0, 4);
lcd.print("CO Level: ");
lcd.print(coLevelValue);
lcd.print(" ");
lcd.print(coLevelValue > coLevelThreshold ? "HIGH" : "LOW");
// Print sensor values to serial monitor for debugging
Serial.print("Methane: ");
Serial.print(methaneValue);
Serial.print(" ");
Serial.println(methaneValue > methaneThreshold ? "HIGH" : "LOW");
Serial.print("Air Quality: ");
Serial.print(airQualityValue);
Serial.print(" ");
Serial.println(airQualityValue > airQualityThreshold ? "HIGH" : "LOW");
Serial.print("Smoke/LPG/CO: ");
Serial.print(smokeLpgCOValue);
Serial.print(" ");
Serial.println(smokeLpgCOValue > smokeLpgCOThreshold ? "HIGH" : "LOW");
Serial.print("CO Level: ");
Serial.print(coLevelValue);
Serial.print(" ");
Serial.println(coLevelValue > coLevelThreshold ? "HIGH" : "LOW");
// Delay before next reading
delay(1000);
}
Testing and Calibration:
- Conduct thorough testing to validate the system’s effectiveness in detecting and managing hazardous gases.
- Calibrate MQ-4 and MQ-136 gas sensors and the combustor unit as needed for optimal performance and reliability.
Benefits:
- Enhances safety by monitoring and managing hazardous gases in sewer systems or confined spaces.
- Prevents explosions, health hazards, and environmental pollution caused by gas leaks.
- Provides real-time notifications to prompt timely action and minimize risks.
- Promotes a safer and healthier living and working environment for residents and personnel.