The Elderly Assistance Line-Following Car is designed to support elders with limited mobility or those on bed rest by delivering essential items such as medicine and food. Utilizing an Arduino Uno, BO motors, an L298N motor driver, and IR sensors, this project ensures that the elderly have easy access to their necessities without needing to move frequently.
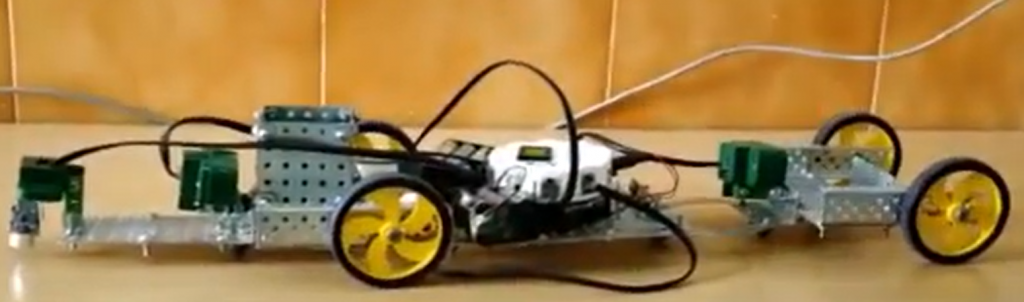
Circuit Connection
- IR Sensors:
- Line Following (LF): Connected to Arduino digital pin 2.
- Object Detection (OD1 & OD2): Connected to Arduino digital pins 3 and 4.
- Motor Driver (L298N):
- IN1 & IN2: Connected to Arduino digital pins 8 and 9.
- IN3 & IN4: Connected to Arduino digital pins 10 and 11.
- Enable A (ENA) & Enable B (ENB): Connected to Arduino PWM pins 5 and 6.
- BO Motors:
- Two motors connected to output A (left side).
- Two motors connected to output B (right side).
The circuit diagram is given below:
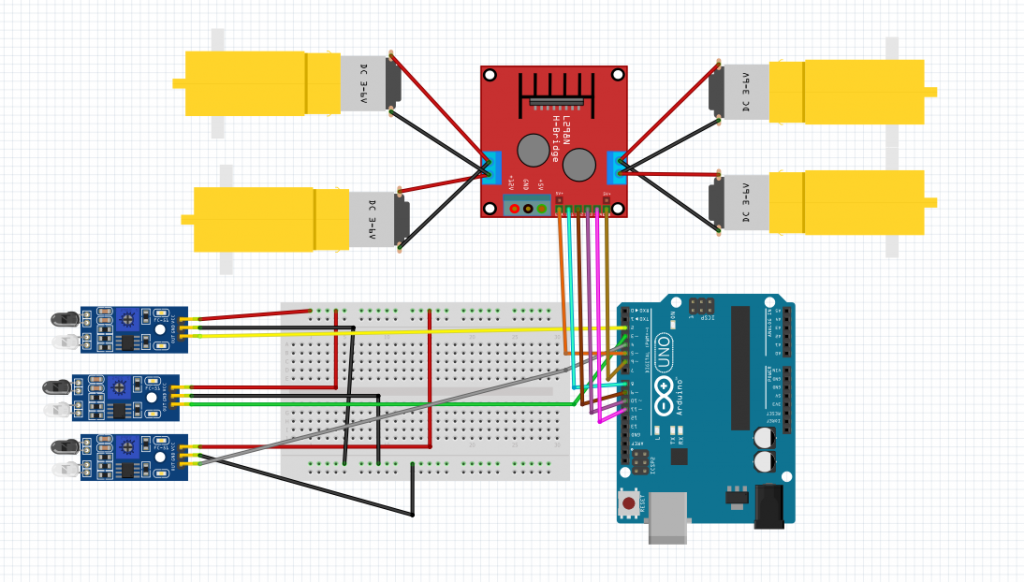
Working
The car follows a line on the floor using the LF IR sensor. OD1 and OD2 IR sensors detect if the essential items are on the car. When both items are present, the car moves forward. If any item is removed, the car stops immediately. It resumes movement only when both items are placed back, ensuring safety and convenience for the elder.
The code of the project is given below:
// Pin definitions
const int LF_SENSOR_PIN = 2;
const int OD1_SENSOR_PIN = 3;
const int OD2_SENSOR_PIN = 4;
const int MOTOR_IN1 = 8;
const int MOTOR_IN2 = 9;
const int MOTOR_IN3 = 10;
const int MOTOR_IN4 = 11;
const int MOTOR_ENA = 5;
const int MOTOR_ENB = 6;
// Motor speed (PWM)
const int MOTOR_SPEED = 200;
void setup() {
// Initialize serial communication for debugging
Serial.begin(9600);
// Initialize sensor pins
pinMode(LF_SENSOR_PIN, INPUT);
pinMode(OD1_SENSOR_PIN, INPUT);
pinMode(OD2_SENSOR_PIN, INPUT);
// Initialize motor driver pins
pinMode(MOTOR_IN1, OUTPUT);
pinMode(MOTOR_IN2, OUTPUT);
pinMode(MOTOR_IN3, OUTPUT);
pinMode(MOTOR_IN4, OUTPUT);
pinMode(MOTOR_ENA, OUTPUT);
pinMode(MOTOR_ENB, OUTPUT);
// Set initial motor speed
analogWrite(MOTOR_ENA, MOTOR_SPEED);
analogWrite(MOTOR_ENB, MOTOR_SPEED);
}
void loop() {
// Read sensor values
int lfValue = digitalRead(LF_SENSOR_PIN);
int od1Value = digitalRead(OD1_SENSOR_PIN);
int od2Value = digitalRead(OD2_SENSOR_PIN);
// Debugging output
Serial.print("LF Sensor: ");
Serial.print(lfValue);
Serial.print(" | OD1 Sensor: ");
Serial.print(od1Value);
Serial.print(" | OD2 Sensor: ");
Serial.println(od2Value);
// Check if both objects are present
if (od1Value == HIGH && od2Value == HIGH) {
// Objects are present, proceed with line following
// Line following logic
if (lfValue == LOW) {
// Line detected, move forward
moveForward();
} else {
// No line detected, stop
stopMotors();
}
} else {
// One or both objects are missing, stop the car
stopMotors();
}
delay(100); // Short delay to avoid rapid state changes
}
// Function to move the car forward
void moveForward() {
digitalWrite(MOTOR_IN1, HIGH);
digitalWrite(MOTOR_IN2, LOW);
digitalWrite(MOTOR_IN3, HIGH);
digitalWrite(MOTOR_IN4, LOW);
}
// Function to stop the motors
void stopMotors() {
digitalWrite(MOTOR_IN1, LOW);
digitalWrite(MOTOR_IN2, LOW);
digitalWrite(MOTOR_IN3, LOW);
digitalWrite(MOTOR_IN4, LOW);
}
Features
- Line Following: Ensures accurate navigation along a predefined path.
- Object Detection: Monitors the presence of essential items, stopping the car when an item is removed.
- Automatic Control: Resumes movement only when items are placed back, preventing unnecessary movement.
- Ease of Use: Provides a reliable and simple solution for delivering essential items to elders.
Application:
Home Care Facilities
In home care facilities, the serving robo can be deployed to autonomously deliver essential items such as medication and food to elderly residents. This reduces the workload on caregivers and ensures that elderly individuals receive their necessities promptly and reliably.
Assisted Living Communities
In assisted living communities, where residents may have varying degrees of mobility, the serving robo provides a valuable service by enabling independent access to essential items. Residents can rely on the car to fetch items without needing to navigate common areas themselves, thereby promoting autonomy and reducing dependency on staff assistance.
Residential Homes
In private residential homes, especially those where elderly individuals live alone or with minimal assistance, the robo serves as a reliable companion for daily tasks. It ensures that items are delivered safely and efficiently, contributing to the overall well-being and comfort of elderly residents who may face challenges in movement.
Community Centers and Senior Activity Centers
At community centers or senior activity centers, the robo can facilitate activities by transporting supplies or equipment needed for recreational or educational programs. This improves the accessibility and inclusivity of community services, fostering a supportive environment for elderly participants.