A Bluetooth-controlled car that can be operated through a smartphone app offers a fascinating project combining multiple technological domains, including wireless communication, sensor integration, machine learning, and image processing. This advanced vehicle includes temperature and humidity sensors, obstacle detection capabilities, and a sophisticated image processing system for enhanced safety.
Key Components and Technologies:
- Bluetooth Module:
- Enables wireless communication between the car and a smartphone app.
- Commonly used module: HC-05 or HC-06.
- Microcontroller:
- Acts as the brain of the car, processing inputs from sensors and commands from the smartphone.
- Commonly used microcontroller: Arduino Uno or ESP32 (which also has built-in Bluetooth).
- Motor Driver:
- Controls the motors based on signals from the microcontroller.
- Commonly used driver: L298N or L293D.
- DC Motors and Wheels:
- Provide mobility to the car.
- Controlled by the motor driver for forward, backward, left, and right movements.
- Temperature and Humidity Sensors:
- Measure the environmental conditions.
- Commonly used sensor: DHT11 or DHT22.
- Ultrasonic Sensors:
- Detect obstacles and measure the distance to them.
- Commonly used sensor: HC-SR04.
- Camera Module:
- Captures images or video for processing.
- Commonly used camera: ESP32-CAM or Raspberry Pi Camera Module.
- Machine Learning and Image Processing:
- Analyzes images to recognize objects, signs, or potential hazards.
- Implemented using frameworks like TensorFlow, OpenCV, or pre-trained models.
Working Principle:
- Bluetooth Communication:
- The smartphone app sends control commands via Bluetooth to the microcontroller.
- The microcontroller decodes these commands and controls the motor driver to move the car accordingly.
- Sensor Data Collection:
- The temperature and humidity sensors continuously monitor the environmental conditions and send data to the microcontroller.
- The ultrasonic sensors scan for obstacles and report distances to the microcontroller.
- Obstacle Avoidance:
- When an obstacle is detected within a predefined distance, the microcontroller commands the car to stop or change direction to avoid a collision.
- Image Processing and Machine Learning:
- The camera module captures real-time video or images.
- The microcontroller or an attached processing unit (like a Raspberry Pi) processes these images to detect objects or hazards using machine learning models.
- Based on the processed data, the microcontroller can make decisions to enhance vehicle safety, such as stopping for pedestrians or recognizing traffic signs.
char t;
void setup() {
pinMode(13,OUTPUT); //left motors forward
pinMode(12,OUTPUT); //left motors reverse
pinMode(11,OUTPUT); //right motors forward
pinMode(10,OUTPUT); //right motors reverse
pinMode(9,OUTPUT); //Led
Serial.begin(9600);
}
void loop() {
if(Serial.available()){
t = Serial.read();
Serial.println(t);
}
if(t == 'F'){ //move forward(all motors rotate in forward direction)
digitalWrite(13,HIGH);
digitalWrite(11,HIGH);
}
else if(t == 'B'){ //move reverse (all motors rotate in reverse direction)
digitalWrite(12,HIGH);
digitalWrite(10,HIGH);
}
else if(t == 'L'){ //turn right (left side motors rotate in forward direction, right side motors doesn't rotate)
digitalWrite(11,HIGH);
}
else if(t == 'R'){ //turn left (right side motors rotate in forward direction, left side motors doesn't rotate)
digitalWrite(13,HIGH);
}
else if(t == 'W'){ //turn led on or off)
digitalWrite(9,HIGH);
}
else if(t == 'w'){
digitalWrite(9,LOW);
}
else if(t == 'S'){ //STOP (all motors stop)
digitalWrite(13,LOW);
digitalWrite(12,LOW);
digitalWrite(11,LOW);
digitalWrite(10,LOW);
}
delay(100);
}
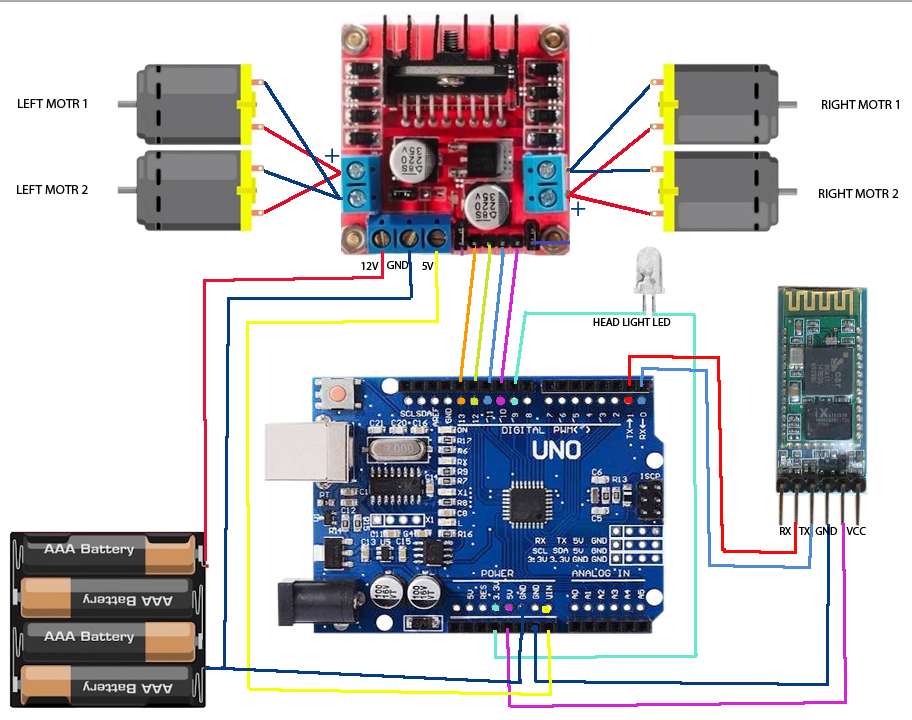