Rainwater harvesting is a sustainable and eco-friendly solution to water scarcity problems. This project aims to automate the process of rainwater collection using an Arduino Uno, a rain sensor, a motor driver, and two DC motors. When the rain sensor detects rainfall above a certain threshold, the motor driver activates the motors to rotate a shed back and forth, collecting rainwater and storing it in a tank. The motors will rotate clockwise, then counterclockwise, continuously until the rain stops.
Components
- Arduino Uno: The central microcontroller.
- Rain Sensor: Detects rainfall.
- Motor Driver (e.g., L298N): Controls the DC motors.
- DC Motors: Rotate the shed to collect rainwater.
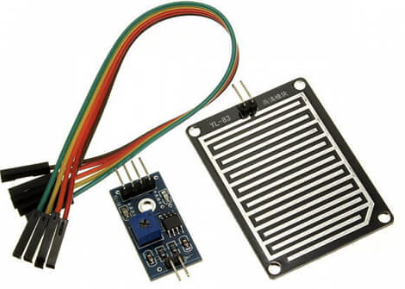
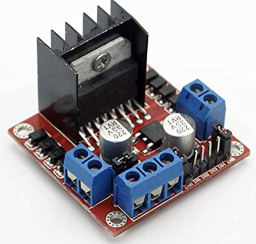
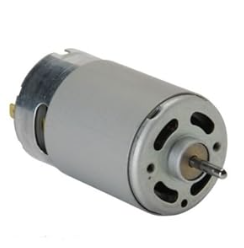
Circuit Connections
- Rain Sensor: Connect VCC to 5V, GND to GND, and OUT to an analog pin (e.g., A0) on the Arduino.
- Motor Driver (L298N):
- Connect IN1, IN2, IN3, and IN4 to digital pins on the Arduino (e.g., 2, 3, 4, 5).
- Connect the motors to the motor output pins on the driver.
- Connect VCC to 12V (or appropriate power supply for your motors) and GND to GND.
- Enable pins (EN1 and EN2) connected to 5V (or controlled via PWM pins if speed control is desired).
Circuit diagram is given below:
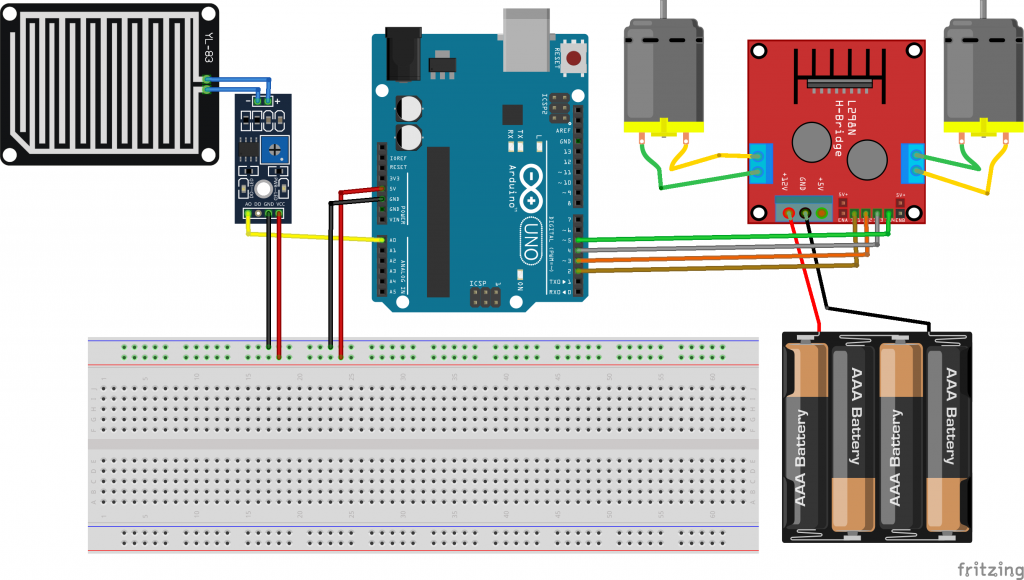
Code is given below:
// Define pins for rain sensor and motor driver
const int rainSensorPin = A0;
const int motor1Pin1 = 2;
const int motor1Pin2 = 3;
const int motor2Pin1 = 4;
const int motor2Pin2 = 5;
// Threshold value for rain detection
const int rainThreshold = 500; // Adjust based on calibration
// Function to stop the motors
void stopMotors() {
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, LOW);
}
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Set motor driver pins as output
pinMode(motor1Pin1, OUTPUT);
pinMode(motor1Pin2, OUTPUT);
pinMode(motor2Pin1, OUTPUT);
pinMode(motor2Pin2, OUTPUT);
// Set initial state of motors to stopped
stopMotors();
}
void loop() {
// Read the value from the rain sensor
int rainValue = analogRead(rainSensorPin);
Serial.print("Rain Sensor Value: ");
Serial.println(rainValue);
// Check if rain is detected above the threshold
if (rainValue > rainThreshold) {
// Rotate motors clockwise for 4 seconds
digitalWrite(motor1Pin1, HIGH);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, HIGH);
digitalWrite(motor2Pin2, LOW);
delay(4000); // Rotate for 4 seconds
// Rotate motors counterclockwise for 4 seconds
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, HIGH);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, HIGH);
delay(4000); // Rotate for 4 seconds
} else {
// Stop the motors if no rain is detected
stopMotors();
}
// Short delay before the next loop
delay(1000);
}
Benefits
- Automated Rainwater Collection: Efficiently collects and stores rainwater without manual intervention.
- Sustainable Solution: Promotes water conservation and reduces reliance on external water sources.
- Scalability: Can be scaled up for larger systems by using more powerful motors and larger collection mechanisms.
Application:
1. Residential Homes
- Individual Houses: Automatically collects rainwater for household uses such as gardening, toilet flushing, and cleaning, reducing reliance on municipal water supply.
- Apartment Complexes: Provides a communal rainwater collection system that can be used for landscape irrigation, car washing, and other non-potable water needs.
2. Agricultural Settings
- Farms and Greenhouses: Collects and stores rainwater for irrigation, reducing the dependency on groundwater and enhancing sustainable farming practices.
- Rural Areas: Provides an efficient method for storing rainwater in areas with limited access to clean water sources, supporting local agriculture and livestock.
3. Commercial Buildings
- Office Buildings: Utilizes collected rainwater for landscape irrigation, cooling towers, and other non-potable uses, reducing water consumption and operational costs.
- Shopping Malls: Enhances sustainability by using harvested rainwater for cleaning, landscaping, and restrooms, contributing to eco-friendly commercial operations.
4. Educational Institutions
- Schools and Universities: Implements rainwater harvesting to educate students about sustainable practices while providing water for gardens, sports fields, and restrooms.
- Research Facilities: Uses harvested rainwater for laboratory cooling systems and irrigation of experimental crops.
5. Public Infrastructure
- Parks and Gardens: Ensures that public green spaces are sustainably watered using harvested rainwater, reducing the strain on municipal water supplies.
- Community Centers: Provides water for community gardens and recreational areas, promoting community-based sustainability efforts.