Project Description:
The RFID-Based Med Bot Project is an innovative project designed to help people manage their medicine schedules efficiently and easily. Using RFID technology, the Med Bot identifies different medicine tags. It displays the corresponding medicine details on an LCD screen. This ensures that the user takes the correct medicine at the right time as prescribed by the doctor and promotes better health outcomes, RFID-Based Med Bot project for students
Components Required:
- Arduino uno with cable
- RFID reader MFRC-522 x 1
- 16×2 LCD I2C display
- Buzzer
- Reak time clock RTC module DS 1307
- Breadboard small
- Jumper wires – Male to Female x 17
Circuit Connection:
- RFID Reader (MFRC522) to Arduino:
- SDA to Pin 10
- SCK to Pin 13
- MOSI to Pin 11
- MISO to Pin 12
- IRQ to No Connection
- GND to GND
- RST to Pin 9
- 3.3V to 3.3V
- LCD I2C to Arduino:
- VCC to 5V
- GND to GND
- SDA to A4
- SCL to A5
- RTC Module to Arduino:
- VCC to 5V
- GND to GND
- SDA to A4
- SCL to A5
- Buzzer to Arduino:
- Positive to Pin 8
- Negative to GND
Conclusion:
The RFID-based Med Bot project offers a reliable and user-friendly solution for managing medication schedules. By leveraging RFID technology and real-time tracking, this system ensures that users are reminded to take the correct medicine at the right time, promoting better health management and adherence to medical regimens.
IMAGE:
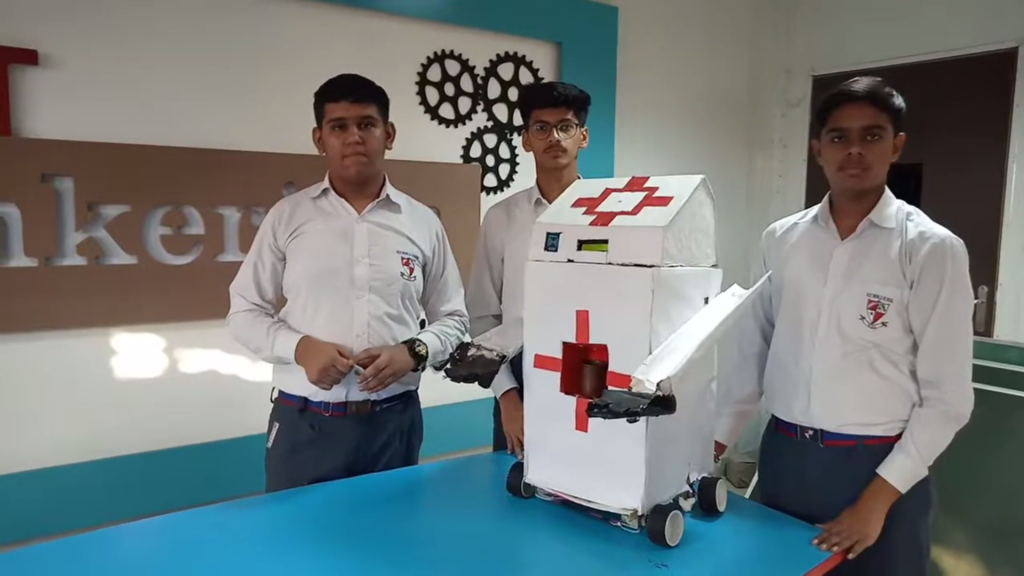
CIRCUIT DIAGRAM:
RFID Reader MFRC-522
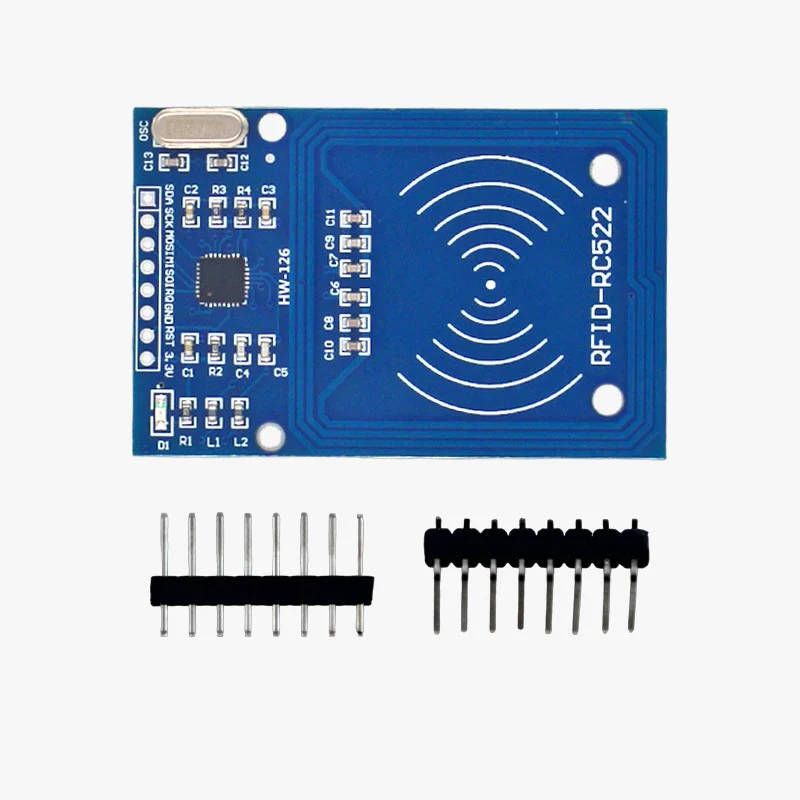
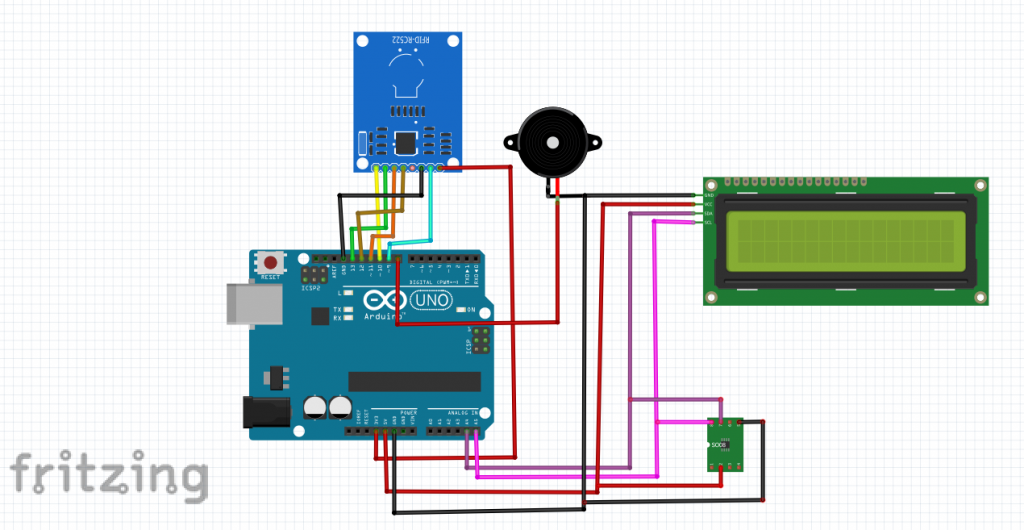
NOTE:
NOTE: Before uploading and running the code IN ARDUINO IDE:
Go to > Sketch > Include Library > Manage Libraries… > Type the library name
OR Go to > Sketch > Include Library > Add .ZIP Library… download the zip library from the link given below.
LiquidCrystal_I2C – https://github.com/fdebrabander/Arduino-LiquidCrystal-I2C-library
MFRC522 – https://github.com/miguelbalboa/rfid
RTC LIB – https://github.com/adafruit/RTClib
CODE:
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <SPI.h>
#include <MFRC522.h>
#include <RTClib.h>
#define SS_PIN 10
#define RST_PIN 9
MFRC522 rfid(SS_PIN, RST_PIN);
LiquidCrystal_I2C lcd(0x27, 16, 2);
RTC_DS3231 rtc;
#define BUZZER_PIN 8
void setup() {
Serial.begin(9600);
SPI.begin();
rfid.PCD_Init();
lcd.init();
lcd.backlight();
if (!rtc.begin()) {
lcd.print("RTC not found!");
while (1)
;
}
if (rtc.lostPower()) {
lcd.print("RTC lost power!");
rtc.adjust(DateTime(F(__DATE__), F(__TIME__)));
}
pinMode(BUZZER_PIN, OUTPUT);
digitalWrite(BUZZER_PIN, LOW);
}
void loop() {
DateTime now = rtc.now();
lcd.setCursor(0, 0);
lcd.print(now.hour(), DEC);
lcd.print(':');
lcd.print(now.minute(), DEC);
lcd.print(':');
lcd.print(now.second(), DEC);
if (!rfid.PICC_IsNewCardPresent() || !rfid.PICC_ReadCardSerial()) {
return;
}
String tagID = "";
for (byte i = 0; i < rfid.uid.size; i++) {
tagID += String(rfid.uid.uidByte[i], HEX);
}
tagID.toUpperCase();
String medicine = getMedicineFromTag(tagID);
if (medicine != "") {
lcd.setCursor(0, 1);
lcd.print("Take: ");
lcd.print(medicine);
soundBuzzer();
}
// Halt for a moment
delay(2000);
lcd.clear();
rfid.PICC_HaltA();
rfid.PCD_StopCrypto1();
}
String getMedicineFromTag(String tagID) {
if (tagID == "12345678") {
return "Med A";
} else if (tagID == "87654321") {
return "Med B";
}
return "";
}
void soundBuzzer() {
digitalWrite(BUZZER_PIN, HIGH);
delay(500);
digitalWrite(BUZZER_PIN, LOW);
}