This project uses an Arduino, an LPG gas sensor, a buzzer, and a fan to detect gas leaks. If gas is detected, the buzzer makes a sound and the fan turns on to warn you. This helps keep your home safe by alerting you quickly to dangerous gas leaks, so you can act right away. It’s an easy way to improve safety and prevent accidents from gas leaks.
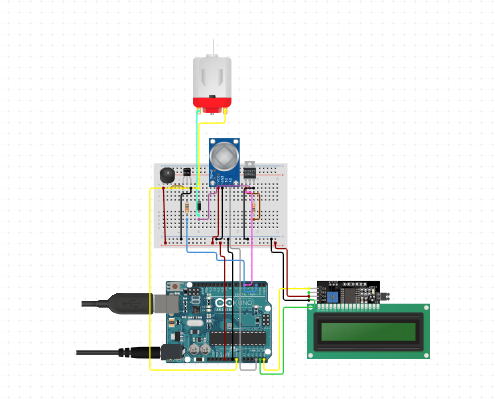
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Define I2C address for LCD display (change if different address)
#define LCD_ADDRESS 0x27
// Initialize the LCD display
LiquidCrystal_I2C lcd(LCD_ADDRESS, 16, 2);
// Define pins
const int gasSensorPin = A3; // Analog pin for LPG gas sensor
const int buzzerPin = 2; // Digital pin for buzzer
const int fanPin = 5; // Digital pin for fan
const int gasThreshold = 300; // Threshold value for gas detection (adjust as needed)
void setup() {
pinMode(gasSensorPin, INPUT);
pinMode(buzzerPin, OUTPUT);
pinMode(fanPin, OUTPUT);
// Initialize LCD
lcd.begin();
lcd.backlight(); // Turn on backlight
// Print initial message on LCD
lcd.setCursor(0, 0);
lcd.print("Gas Detector");
lcd.setCursor(0, 1);
lcd.print("Initializing...");
Serial.begin(9600); // Initialize serial communication for debugging
}
void loop() {
int gasLevel = analogRead(gasSensorPin); // Read gas sensor value
// Print gas sensor value to serial monitor
Serial.print("Gas Level: ");
Serial.println(gasLevel);
// Display gas level on LCD
lcd.setCursor(0, 1);
lcd.print("Gas Level: ");
lcd.print(gasLevel);
// Check if gas level exceeds the threshold
if (gasLevel > gasThreshold) {
digitalWrite(buzzerPin, HIGH); // Turn on buzzer
digitalWrite(fanPin, HIGH); // Turn on fan
lcd.setCursor(0, 0);
lcd.print("ALERT! Gas Leak ");
} else {
digitalWrite(buzzerPin, LOW); // Turn off buzzer
digitalWrite(fanPin, LOW); // Turn off fan
lcd.setCursor(0, 0);
lcd.print("Safe: Gas Level ");
}
delay(1000); // Delay for 1 second before next reading
}