The Arduino 3 LED Blinking project demonstrates basic digital output control using an Arduino microcontroller. It involves programming the Arduino to sequentially blink three LEDs connected to its digital pins. This project is an excellent starting point for beginners to learn about Arduino programming and basic electronics.
Components:
- Arduino Uno (or any compatible Arduino board)
- 3 LEDs (any color)
- 3 current-limiting resistors (typically 220 ohms)
- Breadboard
- Jumper wires
- USB cable for Arduino power and programming
Description:
The Arduino is programmed to turn each LED on and off in sequence with specific time delays between transitions. For example, LED 1 lights up for a second, then turns off while LED 2 lights up, followed by LED 3. This sequence repeats, creating a blinking effect.
Applications:
- Learning Tool: Ideal for beginners to understand Arduino programming basics, such as digital output and timing.
- Indicator Lights: Can be used as simple status indicators in various projects, indicating states like power on/off, operation mode, or error conditions.
- Prototyping: Forms a foundation for more complex projects involving multiple outputs and timed operations, common in IoT prototypes and automation systems.
- Education: Used in STEM education to teach electronics and programming fundamentals in a hands-on, engaging manner.
- Decoration: Provides basic LED blinking effects for decorative purposes in DIY projects or displays.
This project lays the groundwork for more advanced Arduino projects by introducing fundamental concepts of digital output control and timing, making it an essential starting point for electronics enthusiasts and students alike.
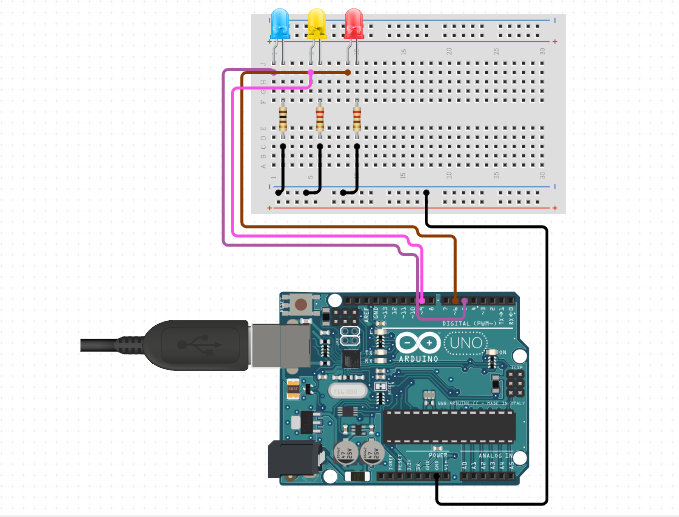
// Include Libraries
#include "Arduino.h"
#include "LED.h"
// Pin Definitions
#define LEDB_PIN_VIN 5
#define LEDR_PIN_VIN 6
#define LEDY_PIN_VIN 9
// Global variables and defines
// object initialization
LED ledB(LEDB_PIN_VIN);
LED ledR(LEDR_PIN_VIN);
LED ledY(LEDY_PIN_VIN);
// define vars for testing menu
const int timeout = 10000; //define timeout of 10 sec
char menuOption = 0;
long time0;
// Setup the essentials for your circuit to work. It runs first every time your circuit is powered with electricity.
void setup()
{
// Setup Serial which is useful for debugging
// Use the Serial Monitor to view printed messages
Serial.begin(9600);
while (!Serial) ; // wait for serial port to connect. Needed for native USB
Serial.println("start");
menuOption = menu();
}
// Main logic of your circuit. It defines the interaction between the components you selected. After setup, it runs over and over again, in an eternal loop.
void loop()
{
if(menuOption == '1') {
// LED - Basic Blue 5mm - Test Code
// The LED will turn on and fade till it is off
for(int i=255 ; i> 0 ; i -= 5)
{
ledB.dim(i); // 1. Dim Led
delay(15); // 2. waits 5 milliseconds (0.5 sec). Change the value in the brackets (500) for a longer or shorter delay in milliseconds.
}
ledB.off(); // 3. turns off
}
else if(menuOption == '2') {
// LED - Basic Red 5mm - Test Code
// The LED will turn on and fade till it is off
for(int i=255 ; i> 0 ; i -= 5)
{
ledR.dim(i); // 1. Dim Led
delay(15); // 2. waits 5 milliseconds (0.5 sec). Change the value in the brackets (500) for a longer or shorter delay in milliseconds.
}
ledR.off(); // 3. turns off
}
else if(menuOption == '3') {
// LED - Basic Yellow 5mm - Test Code
// The LED will turn on and fade till it is off
for(int i=255 ; i> 0 ; i -= 5)
{
ledY.dim(i); // 1. Dim Led
delay(15); // 2. waits 5 milliseconds (0.5 sec). Change the value in the brackets (500) for a longer or shorter delay in milliseconds.
}
ledY.off(); // 3. turns off
}
if (millis() - time0 > timeout)
{
menuOption = menu();
}
}
// Menu function for selecting the components to be tested
// Follow serial monitor for instrcutions
char menu()
{
Serial.println(F("\nWhich component would you like to test?"));
Serial.println(F("(1) LED - Basic Blue 5mm"));
Serial.println(F("(2) LED - Basic Red 5mm"));
Serial.println(F("(3) LED - Basic Yellow 5mm"));
Serial.println(F("(menu) send anything else or press on board reset button\n"));
while (!Serial.available());
// Read data from serial monitor if received
while (Serial.available())
{
char c = Serial.read();
if (isAlphaNumeric(c))
{
if(c == '1')
Serial.println(F("Now Testing LED - Basic Blue 5mm"));
else if(c == '2')
Serial.println(F("Now Testing LED - Basic Red 5mm"));
else if(c == '3')
Serial.println(F("Now Testing LED - Basic Yellow 5mm"));
else
{
Serial.println(F("illegal input!"));
return 0;
}
time0 = millis();
return c;
}
}
}