The Khet Guru project is an advanced agricultural solution designed to help farmers monitor and manage soil conditions using modern technology. This system employs an Arduino microcontroller, a soil moisture sensor, a soil pH sensor, and three LCD displays to provide real-time data on soil health, ensuring optimal crop growth and yield. The Arduino microcontroller acts as the central unit, processing data from various sensors. The soil moisture sensor measures the water content in the soil, while the soil pH sensor determines the soil’s acidity or alkalinity. These sensors provide crucial information necessary for making informed decisions about irrigation and soil treatment. Three LCD displays are used to show the collected data: one display shows the soil moisture level, another indicates the pH value, and the third presents the N, P, K (nitrogen, phosphorus, potassium) values, which are critical nutrients for plant growth. This setup allows farmers to quickly and easily assess the condition of their soil at a glance. In addition to the hardware components, the Khet Guru project includes interfacing with specialized software. This software analyzes the sensor data and provides recommendations on the types and quantities of fertilizers needed to optimize soil health. The software also calculates the cost of the recommended fertilizers, helping farmers budget effectively. Components required for this project include: – Arduino Uno – Soil moisture sensor – Soil pH sensor – I2C LCD – Breadboard and jumper wires – Power supply (batteries or power adapter) Software interface for data analysis and fertilizer recommendations The Khet Guru project represents a fusion of traditional farming practices with modern technology. By providing detailed and actionable soil health information, it empowers farmers to make data-driven decisions, ultimately improving crop productivity and sustainability. |
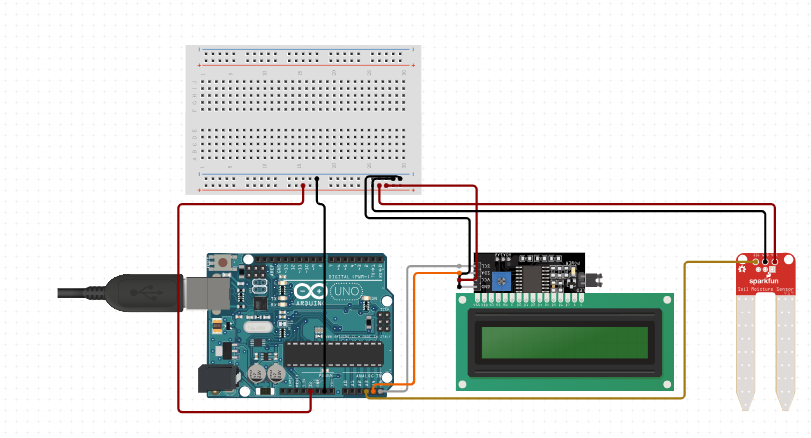
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Set the LCD address to 0x27 for a 16 chars and 2 line display
LiquidCrystal_I2C lcd(0x27, 16, 2);
// Define the analog pins for the sensors
const int soilMoisturePin = A0;
const int soilPhPin = A1;
void setup() {
// Initialize the LCD with 16 columns and 2 rows
lcd.begin(16, 2);
// Print a message to the LCD.
lcd.print("Soil Moisture:");
lcd.setCursor(0, 1);
lcd.print("Soil pH:");
delay(2000); // Display the initial message for 2 seconds
}
void loop() {
// Read the value from the soil moisture sensor
int soilMoistureValue = analogRead(soilMoisturePin);
// Read the value from the soil pH sensor
int soilPhValue = analogRead(soilPhPin);
// Convert the analog readings to a percentage for soil moisture
int soilMoisturePercent = map(soilMoistureValue, 0, 1023, 0, 100);
// Convert the analog readings to a pH value
// Assuming the sensor provides a value between 0-14 for pH, you might need to calibrate this
float soilPh = map(soilPhValue, 0, 1023, 0, 14);
// Display the soil moisture percentage on the LCD
lcd.setCursor(0, 0);
lcd.print("Moisture: ");
lcd.print(soilMoisturePercent);
lcd.print("% "); // Clear any leftover characters
// Display the soil pH value on the LCD
lcd.setCursor(0, 1);
lcd.print("Soil pH: ");
lcd.print(soilPh);
lcd.print(" "); // Clear any leftover characters
// Wait a bit before the next reading
delay(2000);
}