The term “HydroBot” generally refers to a type of robotic system designed for applications involving water, but its specific functionalities can vary depending on the context in which it’s used:
HydroBot for automatic plant care with integrated fire detection and alarming offers the following features:
Automatic Watering: HydroBot can autonomously manage the watering schedule for plants, using soil moisture sensors to deliver the right amount of water at the right time, ensuring optimal hydration without overwatering.
Soil Moisture Monitoring: The robot continuously monitors soil moisture levels, adjusting watering frequency and volume based on real-time data to maintain ideal growing conditions for various plants.
Fire Detection System: Equipped with advanced fire detection sensors, HydroBot can detect early signs of fire such as smoke, heat, and rapid temperature changes in the garden or forest.
Alarm and Alert Mechanism: Upon detecting a potential fire, HydroBot triggers an alarm and sends immediate alerts to designated personnel or systems, enabling swift response and minimizing damage.
Environmental Monitoring: HydroBot tracks additional environmental factors such as temperature, humidity, and light levels, ensuring plants are growing in their optimal conditions.
Adaptability: HydroBot can be programmed to cater to the specific needs of different plants and environments, from home gardens to large agricultural fields and forested areas, providing tailored care and safety solutions.
Sustainable Water Use: By optimizing watering schedules and volumes, HydroBot helps conserve water, promoting sustainable gardening and agricultural practices.
CIRCUIT DIAGRAM
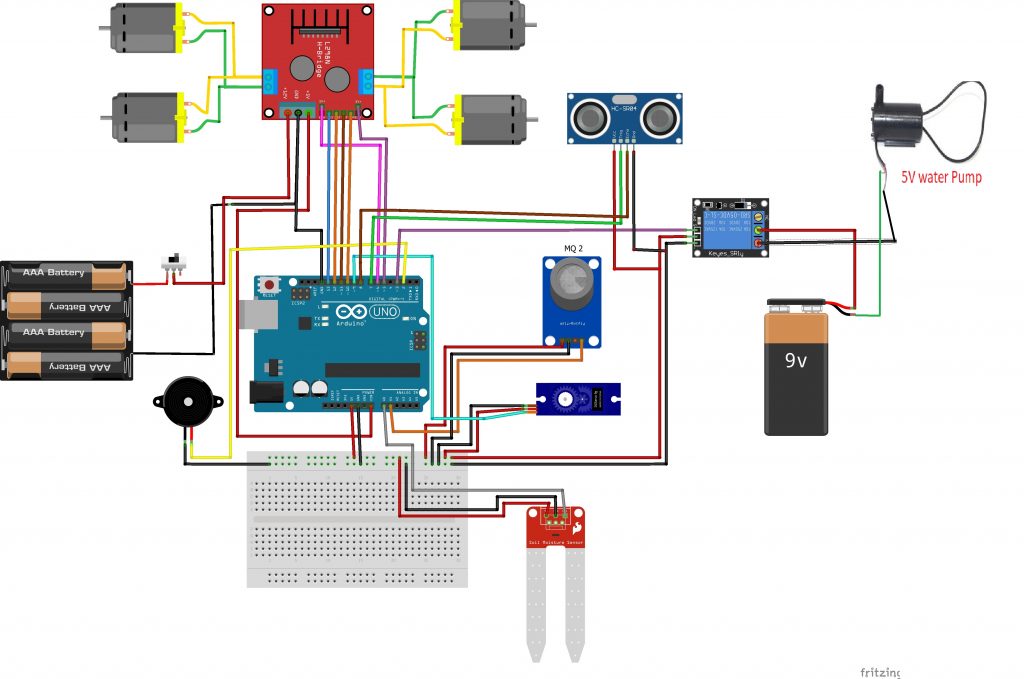
CODE
//Hydrobot Robot _ Rahul Mishra file
#include <Servo.h>
Servo myservo;
#define SOIL_MOISTURE_PIN A0 // Analog pin connected to the soil moisture sensor
#define SMOKE_SENSOR_PIN A1 // Analog pin connected to the smoke sensor
const int buzzerPin = 2;
const int PUMP_PIN = 3; // Digital pin connected to the water pump relay
// Define moisture thresholds for watering
const int dryThreshold = 700; // Adjust this value according to your soil moisture sensor
const int wetThreshold = 500; // Adjust this value according to your soil moisture sensor
const int smokeThreshold = 105;// Adjust this value according to your smoke sensor value
const int trigPin = 7;
const int echoPin = 8;
const int motor1Pin1 = 13;
const int motor1Pin2 = 12;
const int motor2Pin1 = 11;
const int motor2Pin2 = 10;
// Define the maximum distance to detect obstacles
const int maxDistance = 20; // in centimeters
int pos = 0; // variable to store the servo position
void setup() {
// Initialize serial communication
Serial.begin(9600);
pinMode(buzzerPin,OUTPUT);
// Initialize motor control pins
pinMode(motor1Pin1, OUTPUT);
pinMode(motor1Pin2, OUTPUT);
pinMode(motor2Pin1, OUTPUT);
pinMode(motor2Pin2, OUTPUT);
pinMode (5, OUTPUT);// enb2
pinMode (6, OUTPUT);//enb1
// Initialize the ultrasonic sensor pins
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(SOIL_MOISTURE_PIN, INPUT);
pinMode(PUMP_PIN, OUTPUT);
myservo.attach(9);
}
void loop() {
// Measure the distance using the ultrasonic sensor
smoke();
long duration, distance;
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1; // Convert duration to distance in centimeters
// Print the distance to the serial monitor
Serial.print("Distance: ");
Serial.println(distance);
delay(200);
int soilMoisture = analogRead(SOIL_MOISTURE_PIN);
Serial.println("soilMoisture");
Serial.println(soilMoisture);
int smokeLevel = analogRead(SMOKE_SENSOR_PIN);
// Print the smoke level to the serial monitor
Serial.print("Smoke Level: ");
Serial.println(smokeLevel); // If an obstacle is detected within the maximum distance
if (distance <= maxDistance) {
// Move backward and turn right to avoid the obstacle
analogWrite (5, 120);
analogWrite(6, 120);
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, LOW);
delay(100); // Adjust delay as needed
for (pos = 0; pos <= 130; pos += 1)
myservo.write(pos);
delay(15);
if (soilMoisture >= dryThreshold) {
// Soil is dry, turn on the water pump
digitalWrite(PUMP_PIN, HIGH);
Serial.println("Soil is dry. Watering...");
delay(3000); // Run the pump for 5 seconds (adjust as needed)
digitalWrite(PUMP_PIN, LOW);
}
else if (soilMoisture <= wetThreshold) {
// Soil is wet enough, no need to water
for (pos = 130; pos >= 0; pos -= 1)
myservo.write(pos);
delay(15);
digitalWrite(PUMP_PIN, LOW);
Serial.println("Soil is wet enough. No watering needed.");
delay(3000);
digitalWrite(motor1Pin1, 0);
digitalWrite(motor1Pin2, 1);
digitalWrite(motor2Pin1, 1);
digitalWrite(motor2Pin2, 0);
delay(2000);
}
}
if (distance > maxDistance ){
// Move forward
analogWrite (3, 150);
analogWrite(5, 150);
digitalWrite(motor1Pin1, 0);
digitalWrite(motor1Pin2, 1);
digitalWrite(motor2Pin1, 1);
digitalWrite(motor2Pin2, 0);
myservo.write(0);
}
}
void smoke()
{ int smokeLevel = analogRead(SMOKE_SENSOR_PIN);
digitalWrite(buzzerPin,HIGH);
// Print the smoke level to the serial monitor
Serial.print("Smoke Level: ");
Serial.println(smokeLevel);
// Check if the smoke level exceeds the threshold
if (smokeLevel > smokeThreshold) {
// Play high frequency
digitalWrite(buzzerPin,HIGH);
delay(200);
digitalWrite(buzzerPin,LOW);
delay(200);
}
else
{digitalWrite(buzzerPin,LOW);
}}