An ECG device, also known as an electrocardiogram, is a machine that measures the electrical activity of your heart. It uses electrodes placed on your chest and limbs to pick up these tiny electrical signals. The ECG translates those signals into a wavy line on a screen or printout, which helps doctors diagnose heart problems like (irregular heartbeats) or heart attacks.
Components:
- Arduino Uno: Controls the system, reads sensor data, and potentially displays information.
- Pulse Rate Sensor: Detects changes in blood volume with each heartbeat, translating them into an electrical signal.
- I2C LCD (Optional): Displays the calculated heart rate for easy visualization.
- LED (Optional): Provides a visual cue with each heartbeat blink.
- Jumper Wires: Connect all the electronic components.
- Breadboard (Optional): Useful for prototyping the circuit before final assembly.
- Power Supply: AC adapter for the Arduino.
Functionality:
- Pulse Detection: The pulse rate sensor clips onto your fingertip and detects the subtle blood volume changes with each heartbeat. It converts these changes into an electrical signal.
- Signal Processing: The Arduino receives the sensor signal and processes it to identify the peaks corresponding to heartbeats.
- Heart Rate Calculation: Based on the time intervals between the detected peaks, the Arduino calculates your heart rate in beats per minute (bpm).
- Output (Optional):
- The I2C LCD can display your calculated heart rate for easy viewing.
- The LED can blink in sync with your heartbeat for a visual representation.
const int PULSE_SENSOR_PIN = 0; // Analog PIN where the PulseSensor is connected
const int LED_PIN = 13; // On-board LED PIN
const int THRESHOLD = 550; // Threshold for detecting a heartbeat
// Create PulseSensorPlayground object
PulseSensorPlayground pulseSensor;
void setup()
{
// Initialize Serial Monitor
Serial.begin(9600);
// Configure PulseSensor
pulseSensor.analogInput(PULSE_SENSOR_PIN);
pulseSensor.blinkOnPulse(LED_PIN);
pulseSensor.setThreshold(THRESHOLD);
// Check if PulseSensor is initialized
if (pulseSensor.begin())
{
Serial.println(“PulseSensor object created successfully!”);
}
}
void loop()
{
// Get the current Beats Per Minute (BPM)
int currentBPM = pulseSensor.getBeatsPerMinute();
// Check if a heartbeat is detected
if (pulseSensor.sawStartOfBeat())
{
Serial.println(“♥ A HeartBeat Happened!”);
Serial.print(“BPM: “);
Serial.println(currentBPM);
}
// Add a small delay to reduce CPU usage
delay(20);
}
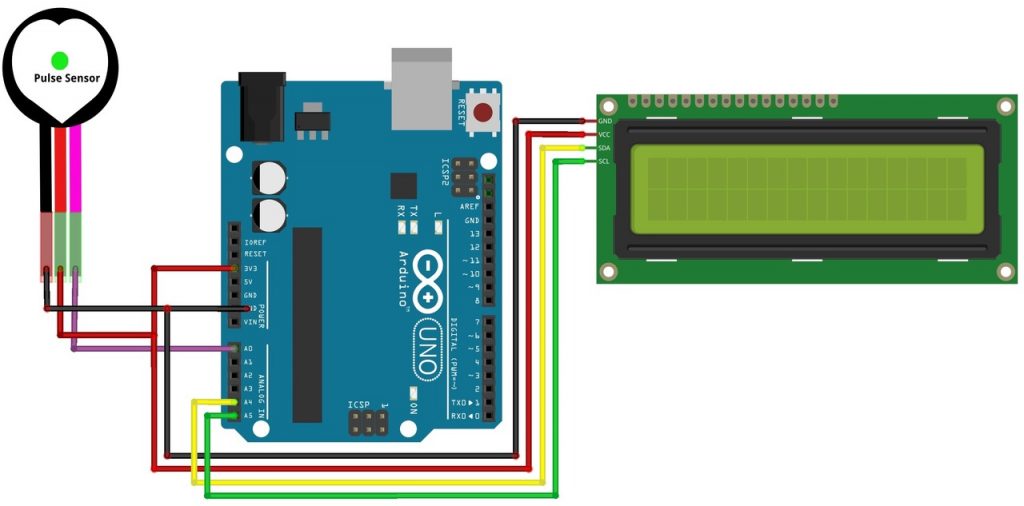