This project aims to demonstrate a system that can read RFID tags, extract their unique IDs, display this information, and send it as an SMS using GSM communication. It showcases how different technologies like RFID and GSM can be integrated with an Arduino to create a simple yet effective information-sharing system. Adjustments and enhancements can be made for specific applications, such as security access control or tracking systems.
Functionality:
RFID Tag Detection: The system scans for RFID tags within its range, detecting and reading the unique IDs of vehicles equipped with RFID tags.
SMS Transmission: Upon detecting a vehicle’s RFID tag, the system triggers the GSM module to compose and dispatch an SMS. This SMS includes the vehicle’s unique ID and relevant details to a predefined phone number.
Real-time Display: The LCD screen presents the scanned vehicle’s unique ID for visual validation.
Status Indication: LED indicators provide visual cues throughout the detection and SMS transmission process, enhancing system feedback.
Conclusion:
The RFID-GSM Vehicle Identification System provides a foundation for RFID-based vehicle identification and communication. It showcases the potential for integrating RFID technology with GSM communication, offering a versatile solution for vehicle tracking, security, and automated information sharing.
#include <SPI.h>
#include <MFRC522.h>
#include <SoftwareSerial.h>
#include <LiquidCrystal_I2C.h>
#define SS_PIN 10
#define RST_PIN 9
String UID;
String textForSMS;
SoftwareSerial sim800l(2, 3); // Connect Tx and Rx of SIM800L to Arduino pins.
MFRC522 mfrc522(SS_PIN, RST_PIN);
LiquidCrystal_I2C lcd(0x27,16,2);
void setup() {
Serial.begin(9600);
sim800l.begin(9600);
sim800l.println("AT");
delay(1000);
if (sim800l.find("OK")) {
Serial.println("SIM800L module is responding");
} else {
Serial.println("Error: SIM800L module is not responding");
}
lcd.init();
lcd.begin(16, 2);
lcd.clear();
lcd.backlight();
lcd.print("TrafficRuleObey");
delay(1000);
lcd.clear();
SPI.begin();
mfrc522.PCD_Init();
pinMode(4, OUTPUT);
pinMode(5, OUTPUT);
pinMode(6, OUTPUT);
}
void loop() {
for (int last = 0; last <= 400; last++) {
scan();
digitalWrite(4, HIGH);
digitalWrite(5, LOW);
digitalWrite(6, LOW);
mfrc();
}
for (int last = 0; last <= 400; last++) {
scan();
digitalWrite(4, LOW);
digitalWrite(5, HIGH);
digitalWrite(6, LOW);
mfrc();
}
digitalWrite(4, LOW);
digitalWrite(5, LOW);
digitalWrite(6, HIGH);
delay(5000);
for (int last = 0; last <= 400; last++) {
scan();
digitalWrite(4, LOW);
digitalWrite(5, HIGH);
digitalWrite(6, LOW);
mfrc();
}
}
void mfrc() {
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) {
lcd.clear();
Serial.print("RFID tag detected: ");
for (byte i = 0; i < mfrc522.uid.size; i++) {
Serial.print(mfrc522.uid.uidByte[i], HEX);
UID += String(mfrc522.uid.uidByte[i], HEX);
UID.toUpperCase();
}
lcd.print("Veh. No.:");
lcd.setCursor(0, 1);
lcd.print(UID);
sim800l.println("AT+CMGF=1");
delay(100);
if (sim800l.find("OK")) {
sim800l.println("AT+CMGS=\"+917011757775\""); // Replace with your desired phone number
delay(100);
sim800l.print("Veh.No.: ");
sim800l.print(UID);
sim800l.print(" has impound of Rs: 3000/-. CheckPoint: SgNo. 12, Rt: BBP Schl.");
sim800l.print("\nRequested to drive responsibly.\nTeam BAL BHARTI PUBLIC SCHOOL, DELHI.");
sim800l.write(0x1A);
delay(100);
Serial.println("");
Serial.print("Message Sent.");
}
lcd.clear();
UID = "";
Serial.println();
mfrc522.PICC_HaltA();
}
}
void scan() {
lcd.setCursor(0,0);
lcd.print("Scanning");
}
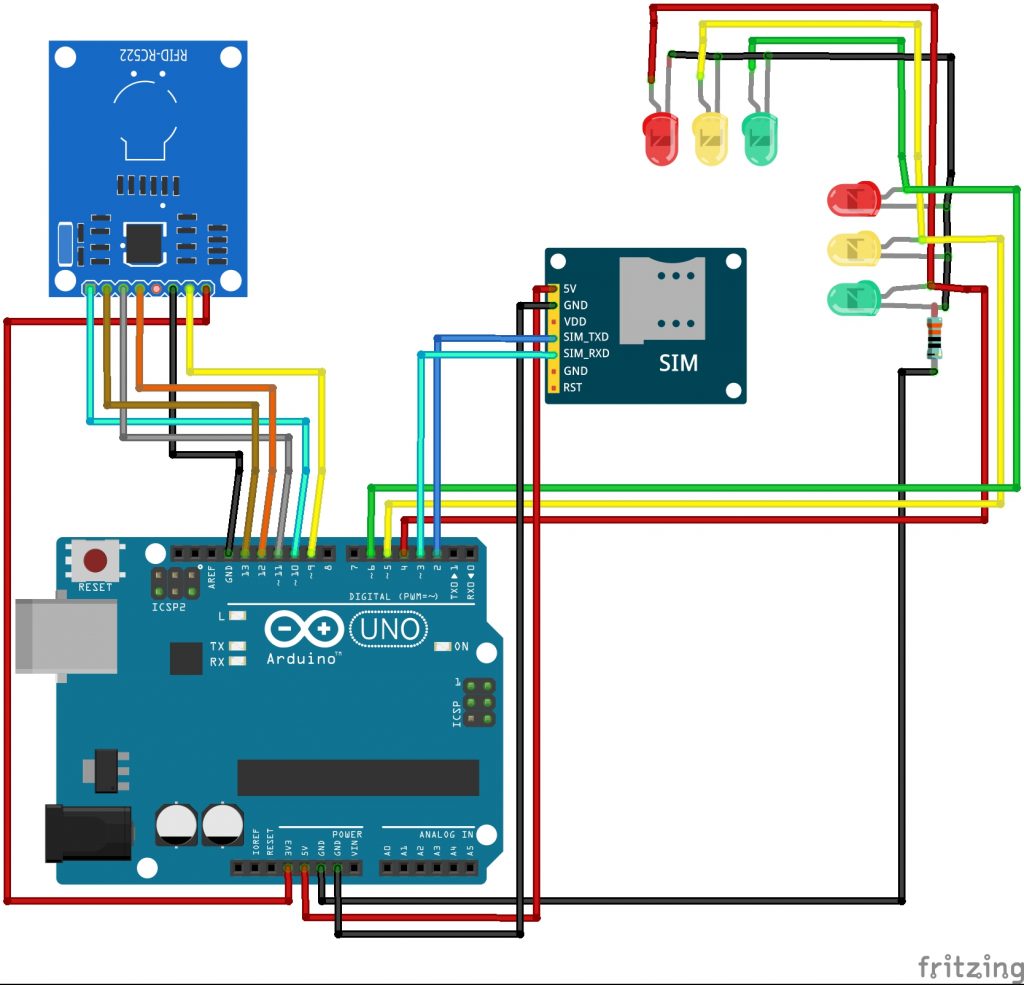