The Crop Protection and Watering System project is designed to optimize irrigation and protect crops using an Arduino-based setup. This project leverages sensors to monitor soil moisture and detect rainfall, automating the watering process to ensure crops receive the right amount of water.
Functionality:
- The system continuously monitors soil moisture levels.
- When the soil is dry, the Arduino activates the water pump to irrigate the crops.
- If the rain drop sensor detects rainfall, the system halts watering to avoid overwatering.
- This automation ensures efficient water usage and provides crops with consistent hydration, improving crop health and yield.
Components and Connections:
Components Required:
- Arduino
- Soil Sensor
- Rain Drop Sensor
- Water Pump
- Jumper Wires [Male to Male X8, Male to Female X3]
- Single channel Relay
- Soil Moisture Sensor:
- VCC to 5V
- GND to GND
- A0 to A0
- Raindrop Sensor:
- VCC to 5V
- GND to GND
- Digital Output to D2
- Water Pump (controlled by a relay):
- Relay VCC to 5V
- Relay GND to GND
- Relay IN to D3
- Water pump VCC and GND through the relay
- Servo Motor:
- VCC to 5V
- GND to GND
- Signal to D9
#include <Servo.h>
// Define pin connections
const int soilMoisturePin = A3;
const int rainDropPin = A0 ;
const int waterPumpPin = 3 ;
const int servoPin = 2;
// Define threshold values
const int dryThreshold = 600; // Adjust this value based on your soil moisture sensor readings
const int rainThreshold = 500; // Adjust this value based on your raindrop sensor readings
Servo myServo;
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Initialize pin modes
pinMode(waterPumpPin, OUTPUT);
myServo.attach(servoPin);
myServo.write(0); // Ensure servo starts at 0 degrees
}
void loop() {
// Read sensor values
int soilMoistureValue = analogRead(soilMoisturePin);
int rainDropValue = analogRead(rainDropPin);
// Print sensor values for debugging
Serial.print("Soil Moisture: ");
Serial.println(soilMoistureValue);
Serial.print("Rain Drop: ");
Serial.println(rainDropValue);
// Determine soil and rain conditions
bool isSoilDry = soilMoistureValue > dryThreshold;
bool isRaining = rainDropValue > rainThreshold;
if (isRaining) {
if (isSoilDry) {
digitalWrite(waterPumpPin, LOW); // Turn off water pump
} else {
myServo.write(90); // Move servo to 90 degrees
}
} else {
if (isSoilDry) {
digitalWrite(waterPumpPin, HIGH); // Turn on water pump
} else {
digitalWrite(waterPumpPin, LOW); // Turn off water pump
myServo.write(0); // Move servo back to 0 degrees
}
}
// Small delay for stability
delay(1000);
}
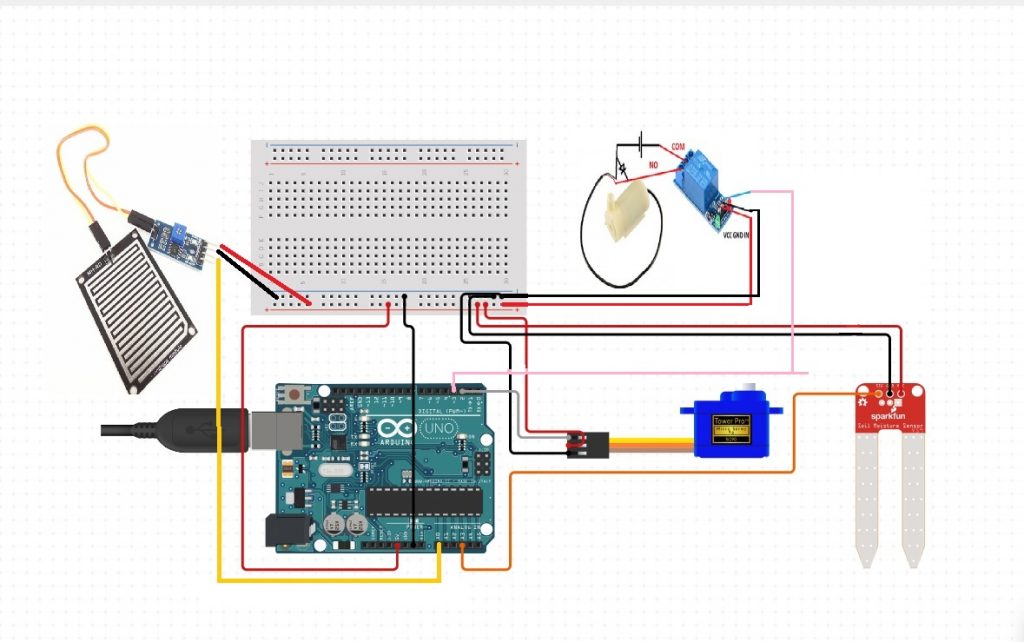