The Arduino Rain Protect project is designed to automate the process of protecting household items and spaces from rain using a raindrop sensor, a servo motor, and an Arduino microcontroller. This project is particularly useful for preventing rain damage to clothes left to dry outside, open windows, or garden equipment.
At the heart of the project is the Arduino microcontroller, which serves as the central control unit. The raindrop sensor is a key component that detects the presence of rain. When raindrops fall on the sensor, it sends a signal to the Arduino, indicating that it is raining. The Arduino then processes this signal and activates a servo motor.
The servo motor is connected to a mechanical system, such as an umbrella-like cover or a sliding mechanism, designed to protect the target area or items. For instance, if the project is used to protect clothes drying on a clothesline, the servo can pull a cover over the clothes when rain is detected. This ensures that clothes remain dry even during unexpected showers. Similarly, it can be used to close windows or cover garden furniture automatically.
Components required for this project include:
– Arduino Uno
– Raindrop sensor module
– Servo motor
– Breadboard and jumper wires
– Power supply (batteries or a power adapter)
– Mechanical parts for the cover or sliding mechanism
This automated system provides a convenient and reliable way to protect household items from rain without manual intervention. It’s especially useful in scenarios where quick response to rain is crucial. By implementing this project, users can prevent water damage and reduce the inconvenience caused by sudden rain showers. The Arduino Rain Protect project showcases the practical application of microcontroller technology in everyday life, enhancing home automation and protection, particularly for drying clothes outdoors.
CIRCUIT DIAGRAM
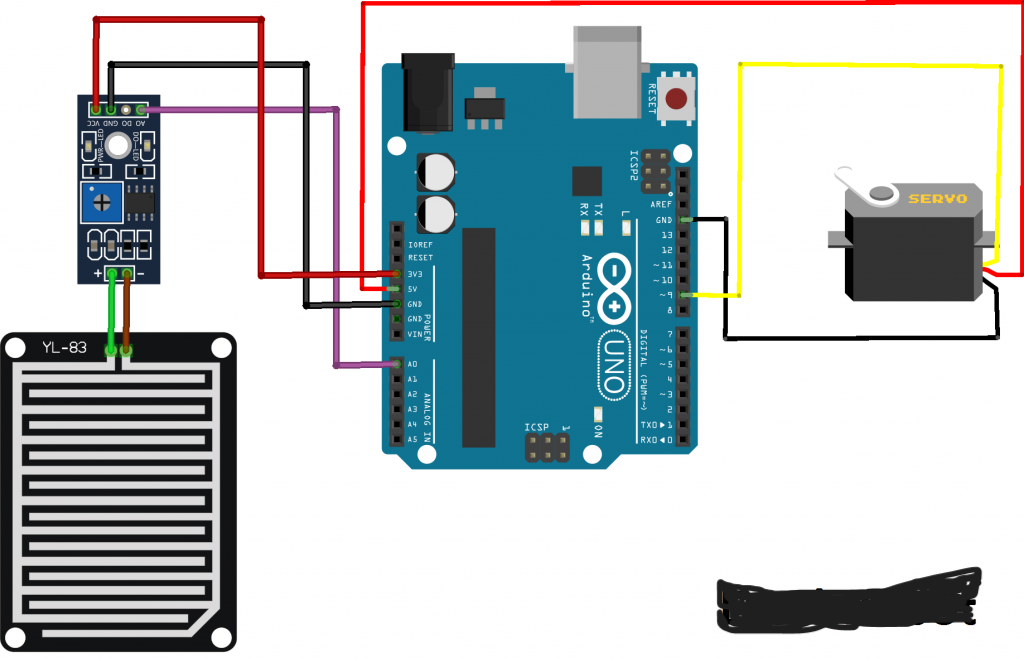
CODE
#include <Servo.h>
Servo myServo;
int servoPin = 9;
int rainSensorPin = A0;
int rainThreshold = 500; // Threshold value for rain detection
void setup() {
// Initialize the serial communication
Serial.begin(9600);
// Attach the servo to the specified pin
myServo.attach(servoPin);
// Set the initial position of the servo
myServo.write(90); // Set the servo to middle position
}
void loop() {
// Read the value from the rain sensor
int sensorValue = analogRead(rainSensorPin);
// Print the sensor value to the serial monitor for debugging
Serial.print("Rain Sensor Value: ");
Serial.println(sensorValue);
// Check if the sensor value is above the threshold
if (sensorValue > rainThreshold) {
// Rain detected, move the servo to 0 degrees
myServo.write(0);
Serial.println("Rain detected, servo moved to 0 degrees");
} else {
// No rain detected, move the servo to 180 degrees
myServo.write(180);
Serial.println("No rain detected, servo moved to 180 degrees");
}
// Add a short delay to avoid rapid movements
delay(500);
}