In this project, our Tech Car is designed to enhance road safety and protect wildlife. This innovative vehicle uses ultrasonic sensors to detect animals on the road, helping to avoid collisions and ensuring both driver safety and wildlife protection.
Components Required:
- Arduino UNO and its Cable x 1
- L298N Motor driver x 1
- Ultrasonic Sensor x 1
- Buzzer x 1
- NPN Transistor x 1
- BO Motor x 2
- Jumper Wires(male to male & male to female)
Component explanation
- Ultrasonic Sensors:
- The car is equipped with ultrasonic sensors that emit sound waves. These sensors measure the time it takes for the sound waves to bounce back after hitting an object, such as an animal. This allows the car to determine the distance to the object.
- L298N Motor Driver:
- The L298N motor driver is the component that controls the car’s motors. It receives signals from the car’s control system, which processes information from the ultrasonic sensors, and then adjusts the motors’ speed and direction to avoid obstacles.
- BO Motors:
- BO motors are used to drive the wheels of the car. These motors receive power and control signals from the L298N motor driver, enabling the car to move forward, reverse, and turn as needed to avoid obstacles.
Detection and Avoidance:
- When the ultrasonic sensors detect an animal in the car’s path, they send a signal to the car’s control system.
- Control System Response:
- The control system processes this information and sends appropriate signals to the L298N motor driver.
- Motor Adjustment:
- The L298N motor driver then adjusts the speed and direction of the BO motors to steer the car away from the animal, preventing a collision.
Protecting Wildlife:
- Preventing Collisions:
- By detecting animals early and taking evasive action, our car helps prevent accidents that could harm both the driver and the wildlife. This reduces the number of animals injured or killed on roads.
- Promoting Safe Habitats:
- Roads often cut through natural habitats, posing a significant risk to wildlife. Our technology helps ensure animals can cross roads safely, maintaining the integrity of their habitats and ecosystems.
- Raising Awareness:
- The use of this technology raises awareness about the impact of driving on wildlife. It encourages drivers to be more mindful of animals and supports broader conservation efforts.
Why It’s Important:
Protecting wildlife is crucial for maintaining biodiversity and healthy ecosystems. Our Tech car showcases how technology can be used to create safer environments for both humans and animals. By integrating ultrasonic sensors, L298N motor drivers, and BO motors, we can significantly reduce wildlife casualties and promote coexistence between road users and nature.
This project demonstrates a practical solution to a common problem, highlighting the potential of technology to make the world a safer place for all living beings.
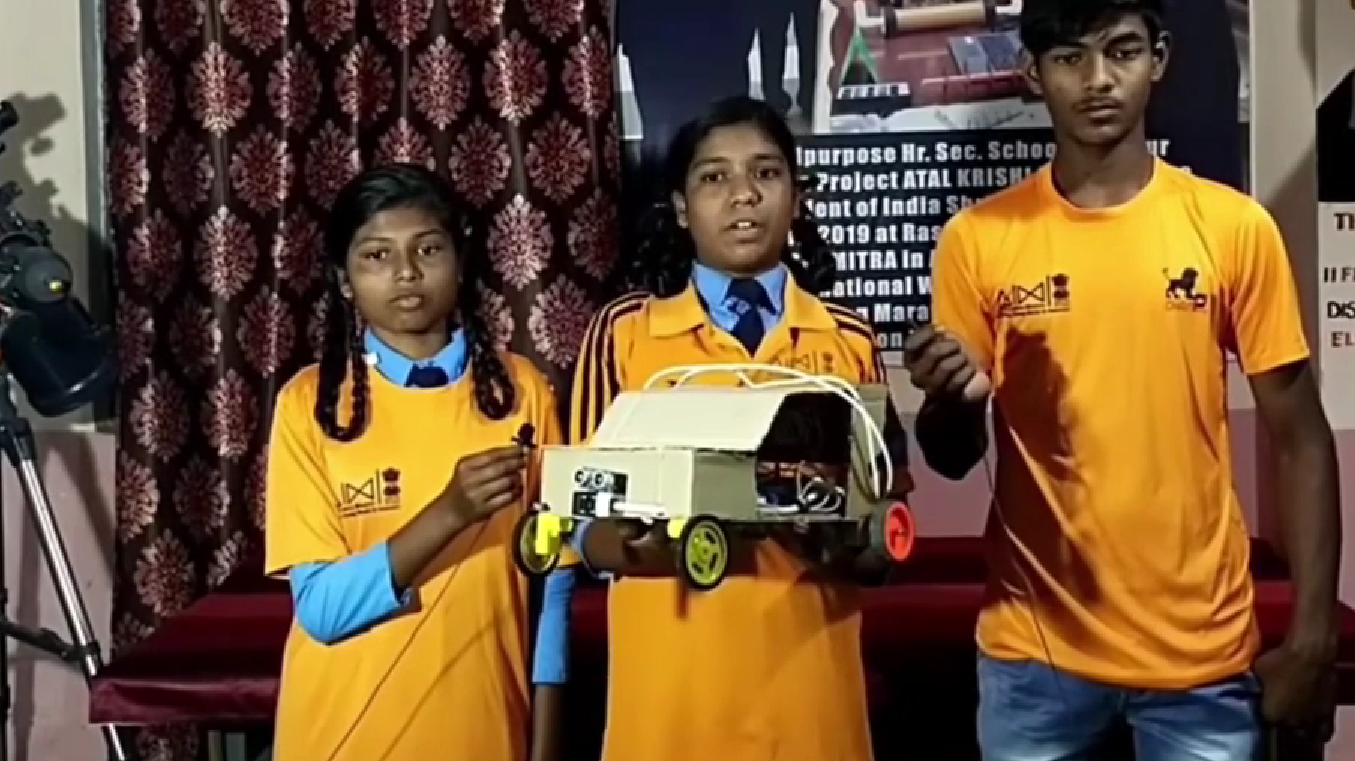
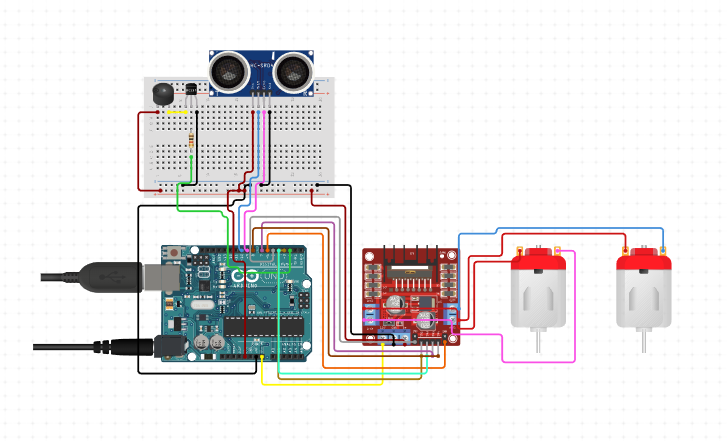
Pin Connections:
1. HC-SR04 Ultrasonic Sensor:
- VCC to 5V on Arduino
- GND to GND on Arduino
- Trig to Pin 9 on Arduino
- Echo to Pin 10 on Arduino
2. L298N Motor Driver Module:
- IN1 to Pin 8 on Arduino
- IN2 to Pin 7 on Arduino
- IN3 to Pin 6 on Arduino
- IN4 to Pin 5 on Arduino
- ENA to Pin 11 on Arduino (PWM)
- ENB to Pin 3 on Arduino (PWM)
- GND to GND on Arduino
- 12V to External Power Supply +12V
- GND to External Power Supply GND
- Out1 to Motor 1 terminal 1
- Out2 to Motor 1 terminal 2
- Out3 to Motor 2 terminal 1
- Out4 to Motor 2 terminal 2
3. DC Motors:
- Motor 1 connections to Out1 and Out2 on the L298N
- Motor 2 connections to Out3 and Out4 on the L298N
SOURCE CODE:
// Pin definitions
const int trigPin = 9;
const int echoPin = 10;
const int buzzerPin = 8;
const int motorA1 = 2;
const int motorA2 = 3;
const int motorB1 = 4;
const int motorB2 = 5;
const int enableA = 6;
const int enableB = 7;
const int safeDistance = 20; // Safe distance in centimeters
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Motor pins setup
pinMode(motorA1, OUTPUT);
pinMode(motorA2, OUTPUT);
pinMode(motorB1, OUTPUT);
pinMode(motorB2, OUTPUT);
pinMode(enableA, OUTPUT);
pinMode(enableB, OUTPUT);
// Ultrasonic sensor pins setup
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
// Buzzer pin setup
pinMode(buzzerPin, OUTPUT);
}
void loop() {
long duration, distance;
// Send ultrasonic pulse
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Read the echo
duration = pulseIn(echoPin, HIGH);
// Calculate the distance
distance = (duration / 2) / 29.1;
// Print distance for debugging
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
if (distance < safeDistance) {
// Obstacle detected, stop and avoid
stopCar();
delay(500);
buzzAlert();
avoidObstacle();
} else {
// No obstacle, move forward
moveForward();
}
delay(100);
}
void moveForward() {
digitalWrite(motorA1, HIGH);
digitalWrite(motorA2, LOW);
digitalWrite(motorB1, HIGH);
digitalWrite(motorB2, LOW);
analogWrite(enableA, 255);
analogWrite(enableB, 255);
}
void stopCar() {
digitalWrite(motorA1, LOW);
digitalWrite(motorA2, LOW);
digitalWrite(motorB1, LOW);
digitalWrite(motorB2, LOW);
analogWrite(enableA, 0);
analogWrite(enableB, 0);
}
void buzzAlert() {
digitalWrite(buzzerPin, HIGH);
delay(100);
digitalWrite(buzzerPin, LOW);
delay(100);
}
void avoidObstacle() {
// Simple right turn to avoid obstacle
digitalWrite(motorA1, LOW);
digitalWrite(motorA2, HIGH);
digitalWrite(motorB1, HIGH);
digitalWrite(motorB2, LOW);
analogWrite(enableA, 255);
analogWrite(enableB, 255);
delay(500); // Adjust delay to set turn angle
// Move forward after avoiding
moveForward();
delay(1000); // Adjust delay to clear obstacle
// Stop car
stopCar();
}