The Car Parking System project features an Arduino Uno, a servo motor, an LCD I2C display, and two IR sensors to improve parking management. This innovative system boosts parking efficiency by reducing the time drivers spend searching for available spots, effectively addressing urban parking issues. The Arduino Uno acts as the central processing unit, managing inputs from the IR sensors that detect vehicles in parking spaces. When the sensors detect a car, the system updates the availability status on the LCD I2C display, providing drivers with real-time information. The servo motor operates a barrier or gate, granting access to available spots only when they are confirmed free by the sensors. This setup maximizes the utilization of each parking spot, prevents unauthorized parking, and reduces obstructions. By integrating these components, the project streamlines the parking process and enhances urban traffic flow efficiency. The reduced search time for parking spaces leads to lower fuel consumption and emissions, offering an environmentally friendly solution. Overall, this Car Parking System significantly advances urban parking management, presenting a practical, technology-driven approach to solving a common problem for drivers in busy cities.
Components used in this project are:
- Arduino UNO with cable
- Servo motor SG-90s
- IR sensor x 2
- LCD I2C display
- Jumper wire – Male to Male x 3
Male to female x 10
Featured Image:
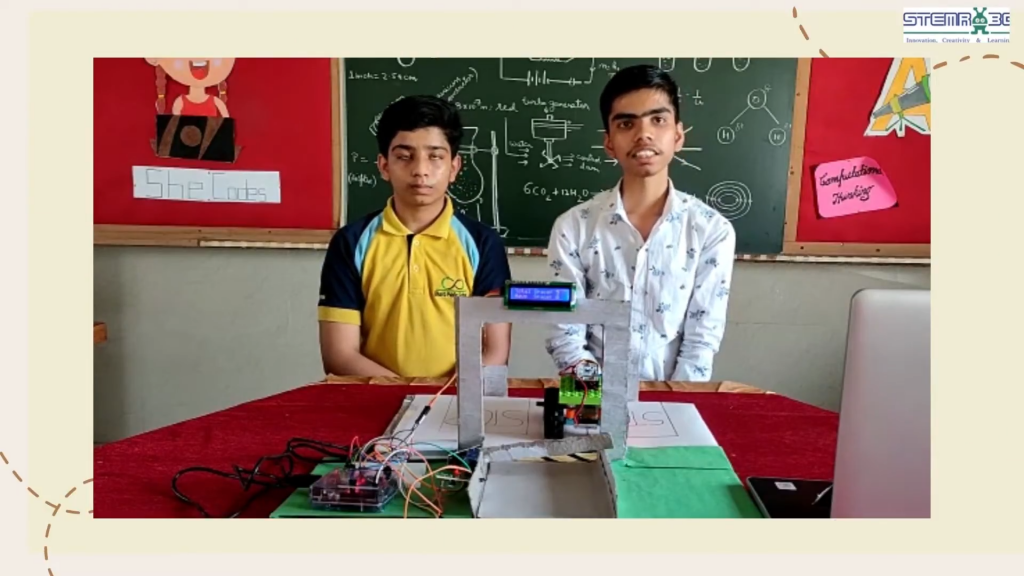
Pin Connections:
- IR Sensor 1:
- VCC -> Arduino 5V
- GND -> Arduino GND
- OUT -> Arduino Digital Pin 2
- IR Sensor 2:
- VCC -> Arduino 5V
- GND -> Arduino GND
- OUT -> Arduino Digital Pin 3
- Servo Motor:
- VCC -> Arduino 5V
- GND -> Arduino GND
- Signal -> Arduino Digital Pin 3
- LCD I2C Display:
- VCC -> Arduino 5V
- GND -> Arduino GND
- SDA -> Arduino A4
- SCL -> Arduino A5
Circuit Diagram:
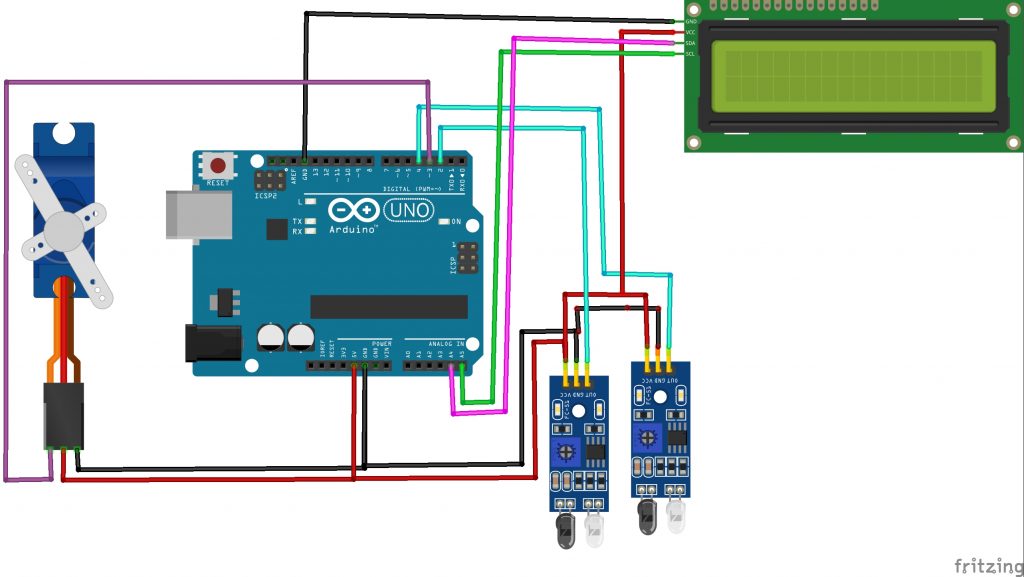
Code:
// Install "LiquidCrystal I2C library by Marco Schwartz" from library manager
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27, 16, 2); // set the LCD address to 0x27 for a 16 chars and 2 line display
#include <Servo.h> //includes the servo library
Servo myservo1;
int ir_s1 = 2;
int ir_s2 = 4;
int Total = 5;
int Space;
int flag1 = 0;
int flag2 = 0;
void setup() {
pinMode(ir_s1, INPUT);
pinMode(ir_s2, INPUT);
myservo1.attach(3);
myservo1.write(100);
lcd.init(); // initialize the lcd
lcd.clear(); // clear LCD screen
lcd.backlight();
lcd.setCursor (0, 0);
lcd.print(" Car Parking ");
lcd.setCursor (0, 1);
lcd.print(" System ");
delay (2000);
lcd.clear();
Space = Total;
}
void loop() {
if (digitalRead (ir_s1) == LOW && flag1 == 0) {
if (Space > 0) {
flag1 = 1;
if (flag2 == 0) {
myservo1.write(0);
Space = Space - 1;
}
} else {
lcd.setCursor (0, 0);
lcd.print(" Sorry no Space ");
lcd.setCursor (0, 1);
lcd.print(" Available ");
delay (1000);
lcd.clear();
}
}
if (digitalRead (ir_s2) == LOW && flag2 == 0) {
flag2 = 1;
if (flag1 == 0) {
myservo1.write(0);
Space = Space + 1;
}
}
if (flag1 == 1 && flag2 == 1) {
delay (1000);
myservo1.write(100);
flag1 = 0, flag2 = 0;
}
lcd.setCursor (0, 0);
lcd.print("Total Space: ");
lcd.print(Total);
lcd.setCursor (0, 1);
lcd.print("Have Space: ");
lcd.print(Space);
}