This smart parking system efficiently manages parking spaces using key components: an IR sensor, a servomotor, an LCD display, and an Arduino Uno microcontroller.
Components:
- IR Sensor: The IR sensor detects vehicles by emitting infrared light. When a car enters or exits, the reflected light changes, indicating the presence or absence of a vehicle. This change is detected and converted into an electrical signal sent to the Arduino Uno.
- Servomotor: The servomotor controls the parking gate. It receives commands from the Arduino Uno to open or close the gate based on the IR sensor’s input.
- LCD Display: The LCD display shows real-time information about available parking spaces. It updates continuously to provide drivers with accurate information as they enter the parking area.
- Arduino Uno: The Arduino Uno is the system’s microcontroller. It processes inputs from the IR sensor, controls the servomotor and updates the LCD display accordingly. It runs the logic to determine parking availability and gate control.
Working Principle:
When a vehicle approaches the parking area, the IR sensor emits infrared light, which reflects off the vehicle and is detected by the sensor. This change in the reflected light indicates a vehicle’s presence. The IR sensor then sends an electrical signal to the Arduino Uno.
The Arduino processes this signal and checks the availability of parking spots. If a spot is available, the Arduino commands the servomotor to open the gate, allowing the vehicle to enter. At the same time, the Arduino updates the LCD display to show the new count of available parking slots.
As the vehicle parks, another IR sensor near the parking spot detects the vehicle’s presence and sends a signal to the Arduino, confirming the slots occupancy. The Arduino then updates the LCD display to decrease the count of available spots.
When a vehicle leaves, the IR sensor detects the departure, and the Arduino commands the servomotor to open the gate for exit. The Arduino updates the LCD display to increase the count of available parking slots.
This smart parking system improves efficiency by providing real-time updates on parking availability and automating gate control, making it easier for drivers to find and access parking spots quickly.
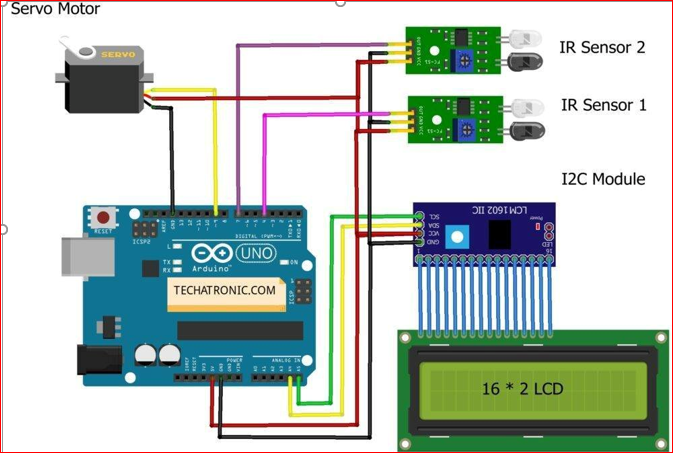
// STEM BASED PROJECT
// Arduino Car Parking System
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x3F,16,2); //Change the HEX address
#include <Servo.h>
Servo myservo1;
int IR1 = 5;
int IR2 = 7;
int Slot = 4; //Enter Total number of parking Slots
int flag1 = 0;
int flag2 = 0;
void setup() {
lcd.begin();
lcd.backlight();
pinMode(IR1, INPUT);
pinMode(IR2, INPUT);
myservo1.attach(9);
myservo1.write(100);
lcd.setCursor (0,0);
lcd.print(" ARDUINO ");
lcd.setCursor (0,1);
lcd.print(" PARKING SYSTEM ");
delay (2000);
lcd.clear();
}
void loop(){
if(digitalRead (IR1) == LOW && flag1==0){
if(Slot>0){flag1=1;
if(flag2==0){myservo1.write(0); Slot = Slot-1;}
}else{
lcd.setCursor (0,0);
lcd.print(" SORRY :( ");
lcd.setCursor (0,1);
lcd.print(" Parking Full ");
delay (3000);
lcd.clear();
}
}
if(digitalRead (IR2) == LOW && flag2==0){flag2=1;
if(flag1==0){myservo1.write(0); Slot = Slot+1;}
}
if(flag1==1 && flag2==1){
delay (1000);
myservo1.write(100);
flag1=0, flag2=0;
}
lcd.setCursor (0,0);
lcd.print(" WELCOME! ");
lcd.setCursor (0,1);
lcd.print("Slot Left: ");
lcd.print(Slot);
}