The Smart Blind Glove is a fantastic tool for people with vision challenges. Picture wearing a glove that acts like a super-sensitive radar, detecting obstacles around you as you move. It’s like having an extra set of eyes on your hand. When the glove senses something in your way, it buzzes gently to alert you. This innovative technology is all about empowering individuals with disabilities, giving them more confidence and independence as they navigate their surroundings. This innovative glove is a game-changer, revolutionizing how individuals with visual impairments interact with the world around them. Its advanced sensors and alert system provide real-time feedback, ensuring a safer and more seamless navigation experience.
Components:
Ultrasonic Sensor: Detects obstacles by emitting sound waves and measuring their return time.
Buzzer: Issues warnings when obstacles are detected.
How it Works:
Obstacle Detection: The ultrasonic sensor sends out sound waves and analyzes their reflections to identify obstacles.
Alert System: The Arduino microcontroller processes sensor data and activates the buzzer when obstacles are too close.
Setting Up/Circuit:
Hardware: Connect the ultrasonic sensor and buzzer to the Arduino board.
Programming: Write a program for the Arduino to interpret sensor data and trigger the buzzer when necessary.
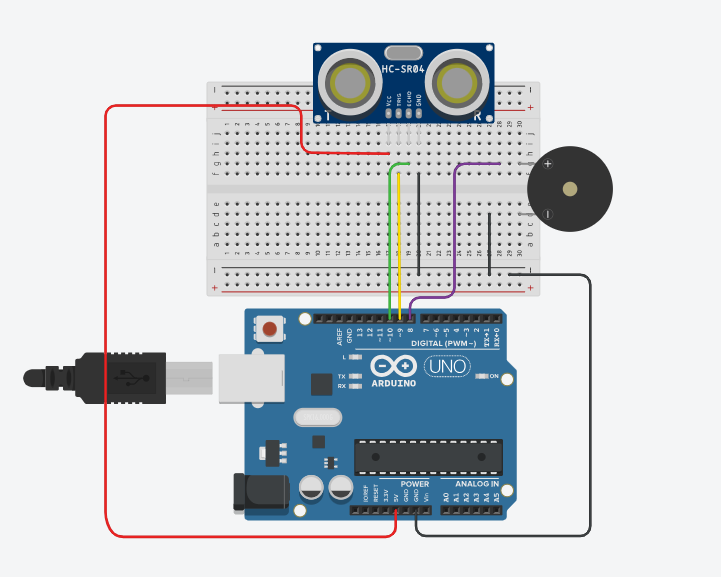
Code :
// Pin configuration
const int trigPin = 9; // Trigger pin of ultrasonic sensor
const int echoPin = 10; // Echo pin of ultrasonic sensor
const int buzzerPin = 8; // Buzzer pin
// Threshold distance to trigger alerts (adjust as needed)
const int alertDistance = 50; // in centimeters
void setup() {
Serial.begin(9600);
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(buzzerPin, OUTPUT);
}
void loop() {
// Measure distance using ultrasonic sensor
int distance = getDistance();
// Display distance on Serial Monitor
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
// Check if an obstacle is within the alert distance
if (distance < alertDistance) {
// Alert the user
alertUser();
} else {
// No obstacle, reset alerts
resetAlerts();
}
delay(500); // Adjust delay as needed
}
int getDistance() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
return pulseIn(echoPin, HIGH) * 0.034 / 2;
}
void alertUser() {
tone(buzzerPin, 1000); // Adjust frequency as needed
}
void resetAlerts() {
noTone(buzzerPin);
}
Advantages:
Enhanced Safety: Reduces the risk of collisions and accidents.
Affordable: Uses readily available components and simple programming.
Customizable: Can be tailored to individual preferences and needs.
Applications:
The Smart Blind Glove is suitable for use in various environments, including indoor and outdoor settings. It provides valuable support for visually impaired individuals during daily activities, such as walking, shopping, and navigating public spaces.