“Drive Aware Notifier” is an innovative project designed to enhance safety on mountain roads, where visibility is often limited and accidents are prevalent due to the inability to see oncoming vehicles. Utilizing cutting-edge technology, this system integrates an Arduino Nano microcontroller, an OLED display, an ultrasonic sensor, and a buzzer to provide real-time awareness to drivers about potential hazards on the road.
At its core, the Drive Aware Notifier acts as a proactive guardian, constantly scanning the surroundings with the ultrasonic sensor. This sensor detects the presence of vehicles or obstacles approaching from either direction, even when they’re out of the driver’s line of sight. The Arduino Nano processes this data and triggers visual and auditory alerts on the OLED display and the buzzer, respectively.
The OLED display serves as the primary interface, presenting vital information such as the distance and direction of the detected object, ensuring that drivers are fully informed about their surroundings. Meanwhile, the buzzer emits distinct warning tones, intensifying as the proximity of the detected object increases, alerting the driver to take immediate action.
This system is not just a passive notifier but a dynamic aid, capable of adapting to varying road conditions and environments. Its compact design and low power consumption make it ideal for installation in vehicles navigating challenging mountainous terrain.
By providing timely and accurate alerts, the Drive Aware Notifier empowers drivers to make informed decisions, significantly reducing the risk of accidents and enhancing overall road safety on mountainous routes. With this innovative solution, drivers can navigate with confidence, knowing that they have a vigilant companion watching over their journey.
COMPONENTS LIST –
- ARDUINO UNO
- ULTRASONIC SENSOR
- OLED DISPLAY
- BUZZER
- JUMPER WIRES – M-M(15)
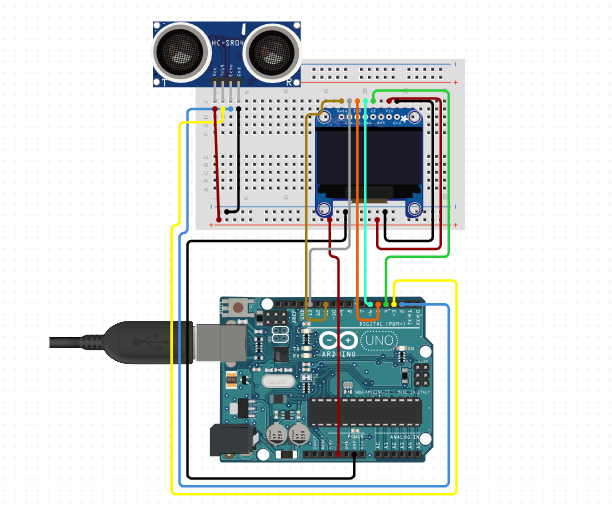
// Include Libraries
#include "Arduino.h"
#include "Buzzer.h"
#include "NewPing.h"
#include "Wire.h"
#include "SPI.h"
#include "Adafruit_SSD1306.h"
#include "Adafruit_GFX.h"
// Pin Definitions
#define BUZZER_PIN_SIG 2
#define HCSR04_PIN_TRIG 4
#define HCSR04_PIN_ECHO 3
#define OLED128X64_PIN_RST 7
#define OLED128X64_PIN_DC 6
#define OLED128X64_PIN_CS 5
// Global variables and defines
// object initialization
Buzzer buzzer(BUZZER_PIN_SIG);
NewPing hcsr04(HCSR04_PIN_TRIG,HCSR04_PIN_ECHO);
#define SSD1306_LCDHEIGHT 64
Adafruit_SSD1306 oLed128x64(OLED128X64_PIN_DC, OLED128X64_PIN_RST, OLED128X64_PIN_CS);
// define vars for testing menu
const int timeout = 10000; //define timeout of 10 sec
char menuOption = 0;
long time0;
// Setup the essentials for your circuit to work. It runs first every time your circuit is powered with electricity.
void setup()
{
// Setup Serial which is useful for debugging
// Use the Serial Monitor to view printed messages
Serial.begin(9600);
while (!Serial) ; // wait for serial port to connect. Needed for native USB
Serial.println("start");
oLed128x64.begin(SSD1306_SWITCHCAPVCC); // by default, we'll generate the high voltage from the 3.3v line internally! (neat!)
oLed128x64.clearDisplay(); // Clear the buffer.
oLed128x64.display();
menuOption = menu();
}
// Main logic of your circuit. It defines the interaction between the components you selected. After setup, it runs over and over again, in an eternal loop.
void loop()
{
if(menuOption == '1') {
// Buzzer - Test Code
// The buzzer will turn on and off for 500ms (0.5 sec)
buzzer.on(); // 1. turns on
delay(500); // 2. waits 500 milliseconds (0.5 sec). Change the value in the brackets (500) for a longer or shorter delay in milliseconds.
buzzer.off(); // 3. turns off.
delay(500); // 4. waits 500 milliseconds (0.5 sec). Change the value in the brackets (500) for a longer or shorter delay in milliseconds.
}
else if(menuOption == '2') {
// Ultrasonic Sensor - HC-SR04 - Test Code
// Read distance measurment from UltraSonic sensor
int hcsr04Dist = hcsr04.ping_cm();
delay(10);
Serial.print(F("Distance: ")); Serial.print(hcsr04Dist); Serial.println(F("[cm]"));
}
else if(menuOption == '3') {
// Monochrome 1.3 inch 128x64 OLED graphic display - Test Code
oLed128x64.setTextSize(1);
oLed128x64.setTextColor(WHITE);
oLed128x64.setCursor(0, 10);
oLed128x64.clearDisplay();
oLed128x64.println("Circuito.io Rocks!");
oLed128x64.display();
delay(1);
oLed128x64.startscrollright(0x00, 0x0F);
delay(2000);
oLed128x64.stopscroll();
delay(1000);
oLed128x64.startscrollleft(0x00, 0x0F);
delay(2000);
oLed128x64.stopscroll();
}
if (millis() - time0 > timeout)
{
menuOption = menu();
}
}
// Menu function for selecting the components to be tested
// Follow serial monitor for instrcutions
char menu()
{
Serial.println(F("\nWhich component would you like to test?"));
Serial.println(F("(1) Buzzer"));
Serial.println(F("(2) Ultrasonic Sensor - HC-SR04"));
Serial.println(F("(3) Monochrome 1.3 inch 128x64 OLED graphic display"));
Serial.println(F("(menu) send anything else or press on board reset button\n"));
while (!Serial.available());
// Read data from serial monitor if received
while (Serial.available())
{
char c = Serial.read();
if (isAlphaNumeric(c))
{
if(c == '1')
Serial.println(F("Now Testing Buzzer"));
else if(c == '2')
Serial.println(F("Now Testing Ultrasonic Sensor - HC-SR04"));
else if(c == '3')
Serial.println(F("Now Testing Monochrome 1.3 inch 128x64 OLED graphic display"));
else
{
Serial.println(F("illegal input!"));
return 0;
}
time0 = millis();
return c;
}
}
}