The All Terrain Rover (ATR) is a robust, remotely controlled vehicle designed for navigating diverse terrains. The core of the system is the ESP8266 NodeMCU, which enables wireless control via Wi-Fi. This microcontroller processes commands and directs the rover’s movement. The rover is powered by a 12V battery, providing sufficient energy for extended operation. It utilizes BO motors, known for their compact design and efficient power output, to drive the wheels and maneuver through rough and uneven surfaces. These motors are controlled by the L298N motor driver, which acts as an interface between the ESP8266 and the motors, regulating their speed and direction.
The ATR’s design makes it capable of handling various environments, making it ideal for exploration, search-and-rescue missions, and remote monitoring in rugged terrains. With the ESP8266, the rover can be controlled wirelessly using a smartphone or web interface, allowing real-time monitoring and control.
Components List:
1. ESP8266 NodeMCU – For wireless control and microcontroller functions.
2. BO Motors – Provide movement and terrain adaptability.
3. L298N Motor Driver – Controls motor speed and direction.
4. 12V Battery – Power source for the rover.
5. Chassis/Wheels – For supporting and moving the rover.
Applications:
– Remote exploration of tough terrains.
– Agriculture for monitoring fields.
– Search and rescue operations in disaster zones.
/*Nodemcu ESP8266 WIFI control car.
* This code created by sritu hobby team.
* https://srituhobby.com
*/
#define BLYNK_TEMPLATE_ID "TMPL3ROy2KbEi"
#define BLYNK_TEMPLATE_NAME "Blynk smart dustbin"
#define BLYNK_AUTH_TOKEN "zfwHfwapRvVyeVBVq_nhhf9qOSr2vUZI"
#define BLYNK_PRINT Serial
#include <ESP8266WiFi.h>
#include <BlynkSimpleEsp8266.h>
//Motor PINs
#define ENA D0
#define IN1 D1
#define IN2 D2
#define IN3 D3
#define IN4 D4
#define ENB D5
bool forward = 0;
bool backward = 0;
bool left = 0;
bool right = 0;
int Speed;
//char auth[] = ""; //Enter your Blynk application auth token
char ssid[] = "Tanya Realme 8 Pro";
char pass[] = "aditya raj"; //192.168.0.128
void setup() {
Serial.begin(9600);
pinMode(ENA, OUTPUT);
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
pinMode(ENB, OUTPUT);
Blynk.begin( BLYNK_AUTH_TOKEN, ssid, pass);
}
BLYNK_WRITE(V3) {
forward = param.asInt();
}
BLYNK_WRITE(V4) {
backward = param.asInt();
}
BLYNK_WRITE(V5) {
left = param.asInt();
}
BLYNK_WRITE(V8) {
right = param.asInt();
}
BLYNK_WRITE(V6) {
Speed = param.asInt();
}
void smartcar() {
if (forward == 1) {
carforward();
Serial.println("carforward");
} else if (backward == 1) {
carbackward();
Serial.println("carbackward");
} else if (left == 1) {
carturnleft();
Serial.println("carfleft");
} else if (right == 1) {
carturnright();
Serial.println("carright");
} else if (forward == 0 && backward == 0 && left == 0 && right == 0) {
carStop();
Serial.println("carstop");
}
}
void loop() {
Blynk.run();
smartcar();
}
void carforward() {
analogWrite(ENA, Speed);
analogWrite(ENB, Speed);
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
}
void carbackward() {
analogWrite(ENA, Speed);
analogWrite(ENB, Speed);
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
}
void carturnleft() {
analogWrite(ENA, Speed);
analogWrite(ENB, Speed);
digitalWrite(IN1, HIGH);
digitalWrite(IN2, LOW);
digitalWrite(IN3, HIGH);
digitalWrite(IN4, LOW);
}
void carturnright() {
analogWrite(ENA, Speed);
analogWrite(ENB, Speed);
digitalWrite(IN1, LOW);
digitalWrite(IN2, HIGH);
digitalWrite(IN3, LOW);
digitalWrite(IN4, HIGH);
}
void carStop() {
digitalWrite(IN1, LOW);
digitalWrite(IN2, LOW);
digitalWrite(IN3, LOW);
digitalWrite(IN4, LOW);
}
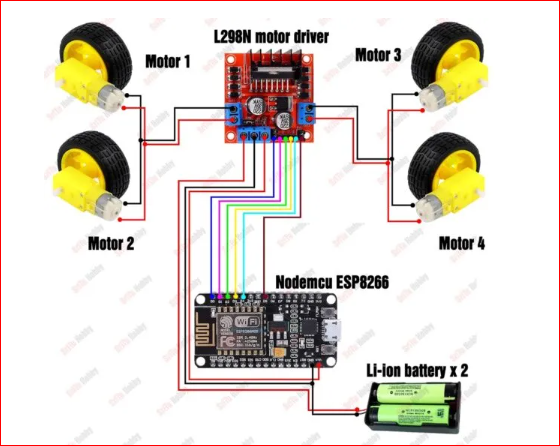