Overview
Our Advanced Water Conservation System helps you efficiently manage water usage for your garden or farm. Using smart technology, this system ensures your plants receive the right amount of water while conserving resources.
Key Features
- Smart Soil Moisture Monitoring: Continuously checks soil moisture levels.
- Automated Watering: Turns on the water pump when the soil is dry.
- Customizable Settings: Adjust moisture levels for different plants.
- Real-Time Alerts: Get notified when soil moisture is low.
- User-Friendly Interface: Monitor and control the system from your smartphone or computer.
Benefits
- Water Conservation: Saves water by watering only when needed.
- Cost Savings: Reduces water bills.
- Healthier Plants: Ensures plants get the right amount of water.
- Easy to Use: Simple to install and maintain.
Components Used
- Arduino: The brain of the system.
- Soil Moisture Sensor: Detects soil moisture levels.
- Relay Module: Controls the water pump.
- Water Pump: Waters the plants.
- Web Interface: Allows remote monitoring and control.
How It Works
- Detect Soil Moisture: The soil moisture sensor checks the moisture level in the soil.
- Analyze Data: If the soil is too dry, the system decides to water the plants.
- Automate Watering: The relay turns on the water pump to water the plants.
- Monitor Remotely: Use the web interface to see real-time data and adjust settings.
Applications
- Home Gardens: Keeps your flowers, vegetables, and lawns well-watered.
- Farms: Helps farmers manage irrigation efficiently.
- Commercial Landscapes: Maintains parks and golf courses with less water.
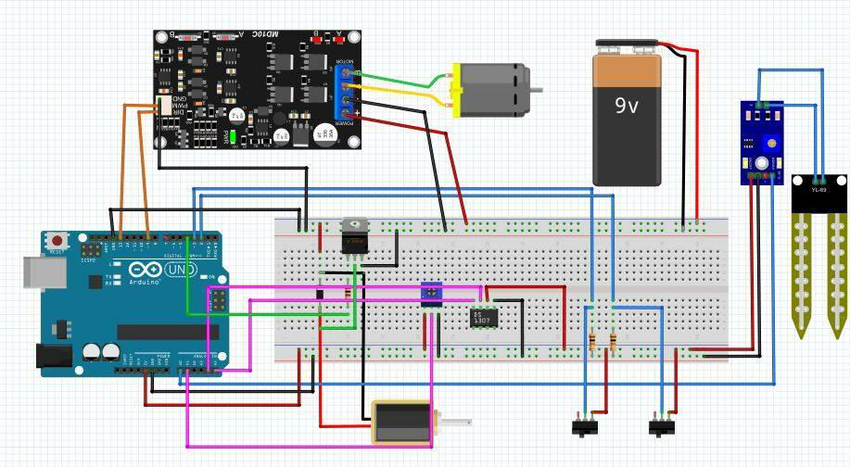
// Include necessary libraries
#include <SPI.h>
// Define pin connections
const int soilMoisturePin = A0; // Soil moisture sensor connected to analog pin A0
const int relayPin = 7; // Relay module connected to digital pin 7
// Define moisture threshold
const int moistureThreshold = 400; // Adjust this value according to your soil moisture sensor
void setup() {
// Initialize serial communication
Serial.begin(9600);
// Initialize relay pin as output
pinMode(relayPin, OUTPUT);
// Ensure the relay is off at startup
digitalWrite(relayPin, LOW);
}
void loop() {
// Read the value from the soil moisture sensor
int soilMoistureValue = analogRead(soilMoisturePin);
// Print the moisture value to the serial monitor
Serial.print("Soil Moisture Value: ");
Serial.println(soilMoistureValue);
// Check if the soil moisture level is below the threshold
if (soilMoistureValue < moistureThreshold) {
// If below threshold, turn on the relay to water the plants
digitalWrite(relayPin, HIGH);
Serial.println("Watering the plants...");
} else {
// If above threshold, turn off the relay
digitalWrite(relayPin, LOW);
Serial.println("Soil moisture level is adequate.");
}
// Wait for a second before taking another reading
delay(1000);
}