This project is a hoverboard designed for blind individuals. It uses an Arduino, an ultrasonic sensor, two motors, and a motor driver module. The ultrasonic sensor detects obstacles in the path. When an obstacle is detected, the Arduino controls the motors to turn the hoverboard away from it. This helps the blind person avoid obstacles and navigate safely.
Components Required
- Arduino Board (Arduino Uno) – 1
- Ultrasonic Sensor ( HC-SR04) – 1
- DC Motors – 2
- Motor Driver Module (e.g., L298N or L293D) – 1
- Wheels – 2
- Battery(12V) – 1
- Connecting Wires – 10-15(M-M)
- Breadboard – 1
Connections
- Ultrasonic Sensor (HC-SR04)
- VCC: Connect to Arduino 5V
- GND: Connect to Arduino GND
- Trig: Connect to Arduino digital pin D9
- Echo: Connect to Arduino digital pin D8
- Motor Driver Module (L298N or L293D)
- VCC: Connect to the positive terminal of the battery pack
- GND: Connect to the negative terminal of the battery pack and Arduino GND
- Input 1 (IN1): Connect to Arduino digital pin D2
- Input 2 (IN2): Connect to Arduino digital pin D3
- Input 3 (IN3): Connect to Arduino digital pin D4
- Input 4 (IN4): Connect to Arduino digital pin D7
- Output 1 (OUT1): Connect to the first terminal of Motor 1
- Output 2 (OUT2): Connect to the second terminal of Motor 1
- Output 3 (OUT3): Connect to the first terminal of Motor 2
- Output 4 (OUT4): Connect to the second terminal of Motor 2
// Define pins for Ultrasonic Sensor
const int trigPin = 9;
const int echoPin = 8;
// Define pins for Motor Driver
const int motor1Pin1 = 2;
const int motor1Pin2 = 3;
const int motor2Pin1 = 4;
const int motor2Pin2 = 7;
long duration;
int distance;
void setup() {
// Set up the pins for the ultrasonic sensor
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
// Set up the pins for the motor driver
pinMode(motor1Pin1, OUTPUT);
pinMode(motor1Pin2, OUTPUT);
pinMode(motor2Pin1, OUTPUT);
pinMode(motor2Pin2, OUTPUT);
Serial.begin(9600);
}
void loop() {
// Clear the trigPin by setting it LOW
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
// Trigger the ultrasonic sensor by setting the trigPin HIGH for 10 microseconds
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Read the echoPin, which returns the sound wave travel time in microseconds
duration = pulseIn(echoPin, HIGH);
// Calculate the distance
distance = duration * 0.034 / 2;
// Print the distance to the serial monitor
Serial.print("Distance: ");
Serial.println(distance);
if (distance < 20) {
// If an obstacle is detected within 20 cm, turn
stopMotors();
delay(500);
turnRight();
delay(1000);
} else {
// Move forward
moveForward();
}
delay(200);
}
void moveForward() {
digitalWrite(motor1Pin1, HIGH);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, HIGH);
digitalWrite(motor2Pin2, LOW);
}
void turnRight() {
digitalWrite(motor1Pin1, HIGH);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, HIGH);
}
void stopMotors() {
digitalWrite(motor1Pin1, LOW);
digitalWrite(motor1Pin2, LOW);
digitalWrite(motor2Pin1, LOW);
digitalWrite(motor2Pin2, LOW);
}
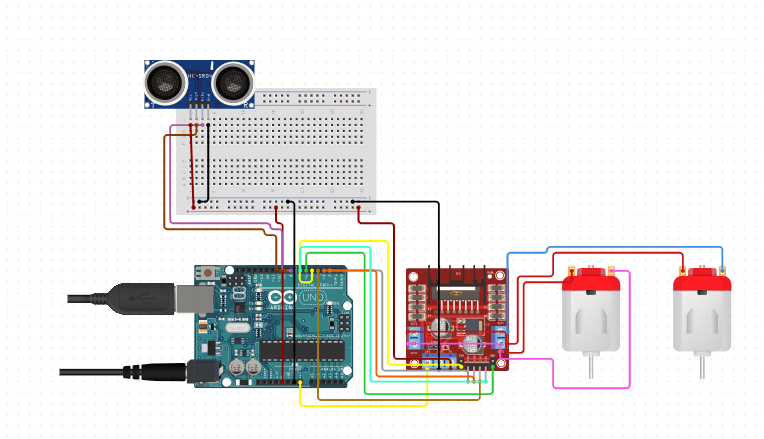