The Life Saviour Project aims to develop a robotic hand to aid individuals with hand disabilities or those who have lost hand functionality. This innovative solution uses flex sensors, an Arduino microcontroller, and servomotors to create a functional robotic hand hand that copies natural movements. The Life Saviour Project offers a practical and impactful solution for individuals with hand disabilities, empowering them to live more independently and perform daily activities with greater ease.
Components Required:
- Flex Sensor x5
- Arduino Uno x1
- Servomotors x5
- Breadboard and Jumper wires
Components and their functions:
- Flex Sensors: These sensors are attached to a glove wear on the user’s functional hand. They measure the degree of bending in the fingers, converting physical movements into electrical signals.
- Arduino: The Arduino microcontroller processes the signals from the flex sensors. It acts as the brain of the project, interpreting the sensor data and sending commands to the servomotors.
- Servomotors: These motors are responsible for moving the fingers of the robotic hand. They receive signals from the Arduino and adjust their positions accordingly to replicate the bending of the user’s fingers.
Connections:
Servos:
servo_1: Signal pin to Arduino digital pin 3, power to 5V, ground to GND.
servo_2: Signal pin to Arduino digital pin 5, power to 5V, ground to GND.
servo_3: Signal pin to Arduino digital pin 6, power to 5V, ground to GND.
servo_4: Signal pin to Arduino digital pin 9, power to 5V, ground to GND.
servo_5: Signal pin to Arduino digital pin 10, power to 5V, ground to GND.
Flex Sensors:
flex_1: One end to Arduino analog pin A0, the other end to 5V and GND through a 10kΩ resistor.
flex_2: One end to Arduino analog pin A1, the other end to 5V and GND through a 10kΩ resistor.
flex_3: One end to Arduino analog pin A2, the other end to 5V and GND through a 10kΩ resistor.
flex_4: One end to Arduino analog pin A3, the other end to 5V and GND through a 10kΩ resistor.
flex_5: One end to Arduino analog pin A4, the other end to 5V and GND through a 10kΩ resistor.
Working:
- Movement Detection: The user wears a glove fitted with flex sensors on their functional hand. As the user bends their fingers, the flex sensors detect the degree of bending.
- Signal Processing: The flex sensors send the bending data to the Arduino, which processes this information and determines how the servomotors should move.
- Copying Movements: The servomotors in the robotic hand receive commands from the Arduino, adjusting their positions to the movements of the user’s fingers in real-time. This allows the robotic hand to perform actions similar to a natural hand.
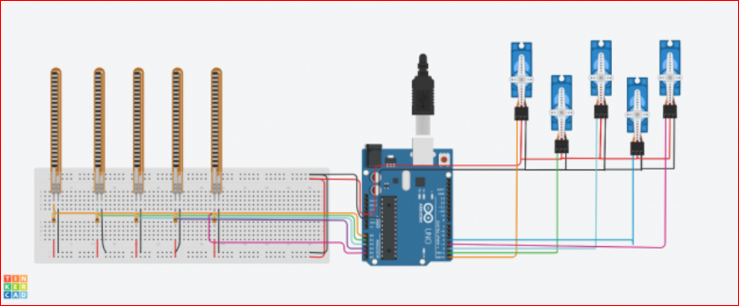
#include <Servo.h>
Servo servo_1;
Servo servo_2;
Servo servo_3;
Servo servo_4;
Servo servo_5;
const int flex_1 = A0;
const int flex_2 = A1;
const int flex_3 = A2;
const int flex_4 = A3;
const int flex_5 = A4;
void setup() {
servo_1.attach(2); // Adjust these pin numbers to match your wiring
servo_2.attach(3); // Adjust these pin numbers to match your wiring
servo_3.attach(4); // Adjust these pin numbers to match your wiring
servo_4.attach(5); // Adjust these pin numbers to match your wiring
servo_5.attach(6); // Adjust these pin numbers to match your wiring
}
void loop() {
int flex_1_val = analogRead(flex_1);
int servo_1_pos = map(flex_1_val, 800, 900, 0, 180);
servo_1_pos = constrain(servo_1_pos, 0, 180);
servo_1.write(servo_1_pos);
int flex_2_val = analogRead(flex_2);
int servo_2_pos = map(flex_2_val, 800, 900, 0, 180);
servo_2_pos = constrain(servo_2_pos, 0, 180);
servo_2.write(servo_2_pos);
int flex_3_val = analogRead(flex_3);
int servo_3_pos = map(flex_3_val, 800, 900, 0, 180);
servo_3_pos = constrain(servo_3_pos, 0, 180);
servo_3.write(servo_3_pos);
int flex_4_val = analogRead(flex_4);
int servo_4_pos = map(flex_4_val, 800, 900, 0, 180);
servo_4_pos = constrain(servo_4_pos, 0, 180);
servo_4.write(servo_4_pos);
int flex_5_val = analogRead(flex_5);
int servo_5_pos = map(flex_5_val, 800, 900, 0, 180);
servo_5_pos = constrain(servo_5_pos, 0, 180);
servo_5.write(servo_5_pos);
delay(100); // Adjust the delay as needed
}