This project uses a device that automatically turns off power to save electricity once a device finishes charging. It’s controlled through a phone app, which makes it easy to use. This helps prevent wasting electricity and ensures safety by stopping unnecessary power flow. By managing energy usage effectively, this setup promotes environmentally-friendly habits and reduces electricity costs. It’s a practical solution for households and schools to adopt smart energy practices and contribute to a sustainable environment.
Components Required:
- Arduino Uno – 1
- Bluetooth Module (e.g., HC-05 or HC-06) – 1
- Servo Motor – 1
- Switch – 1
- Battery(9V) – 1
- Battery – 1
- Jumper Wires-10-15(M-M)
- Power Supply for Arduino (USB or External)
- For controlling Smartphone with Bluetooth and Mobile App
Connections:
Arduino Uno:
- Bluetooth Module (HC-05/HC-06):
- VCC to 5V on Arduino
- GND to GND on Arduino
- TX to RX (pin 8) on Arduino
- RX to TX (pin 7) on Arduino
- Servo Motor:
- Signal wire (usually yellow or white) to a PWM pin A0 on Arduino
- VCC and GND to 5V and GND on Arduino respectively
- Switch:
- One terminal connected to the battery positive
- The other terminal connected to the relay module’s normally open (NO) contact
- Battery:
- Connected to the battery for on the breadboard for taking the common of positive and negative power supply
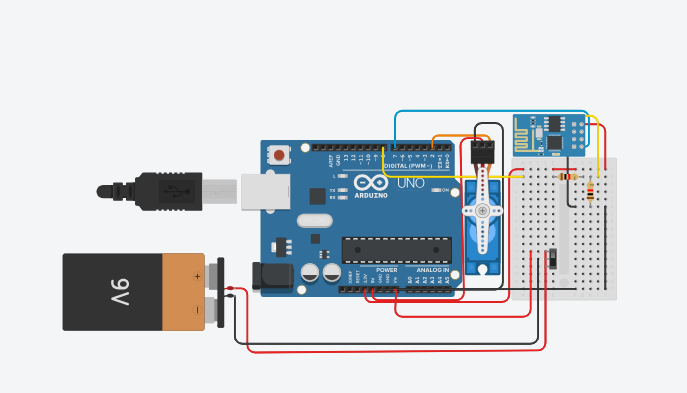
#include <SoftwareSerial.h>
#include <Servo.h>
// Bluetooth module pins
#define RX 7 // Arduino RX to Bluetooth TX
#define TX 8 // Arduino TX to Bluetooth RX
// Servo motor pin
#define SERVO_PIN A0
// Variables
SoftwareSerial bluetooth(RX, TX);
Servo servo;
void setup() {
Serial.begin(9600); // Initialize serial communication for debugging
bluetooth.begin(9600); // Initialize Bluetooth communication
servo.attach(SERVO_PIN); // Attach servo to SERVO_PIN
pinMode(LED_BUILTIN, OUTPUT); // Built-in LED as output for status
// Initialize servo to default position (off state)
servo.write(0);
}
void loop() {
if (bluetooth.available()) {
char command = bluetooth.read();
if (command == '1') {
// Start charging process (simulated)
digitalWrite(LED_BUILTIN, HIGH); // Turn on built-in LED for indication
// Simulate charging completion after 10 seconds
delay(10000);
// Charging complete, activate servo to turn off switch
servo.write(90); // Adjust servo position based on mechanical setup
// Send confirmation message to mobile app
bluetooth.print("Charging complete. Switch turned off.");
}
}
}
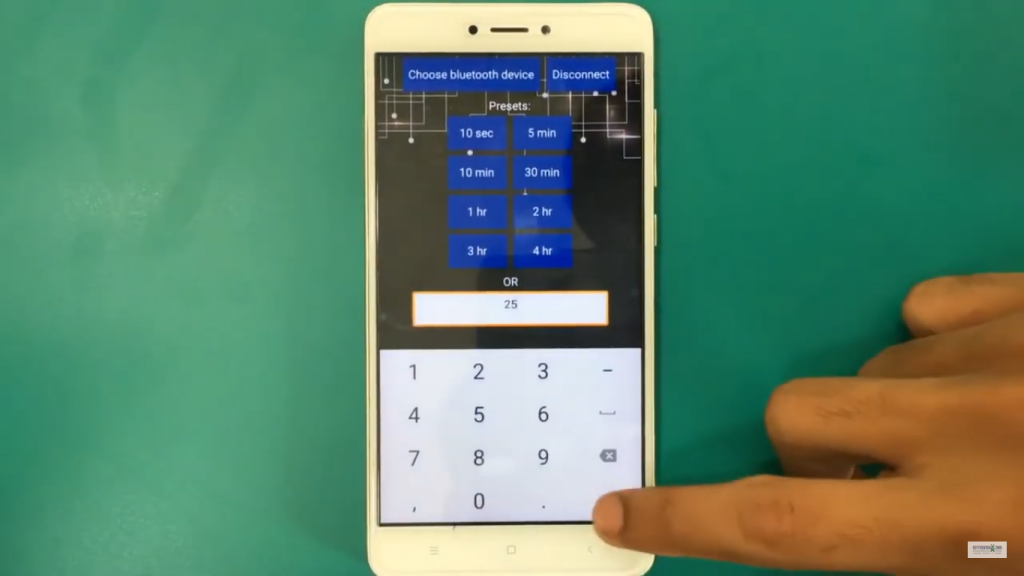
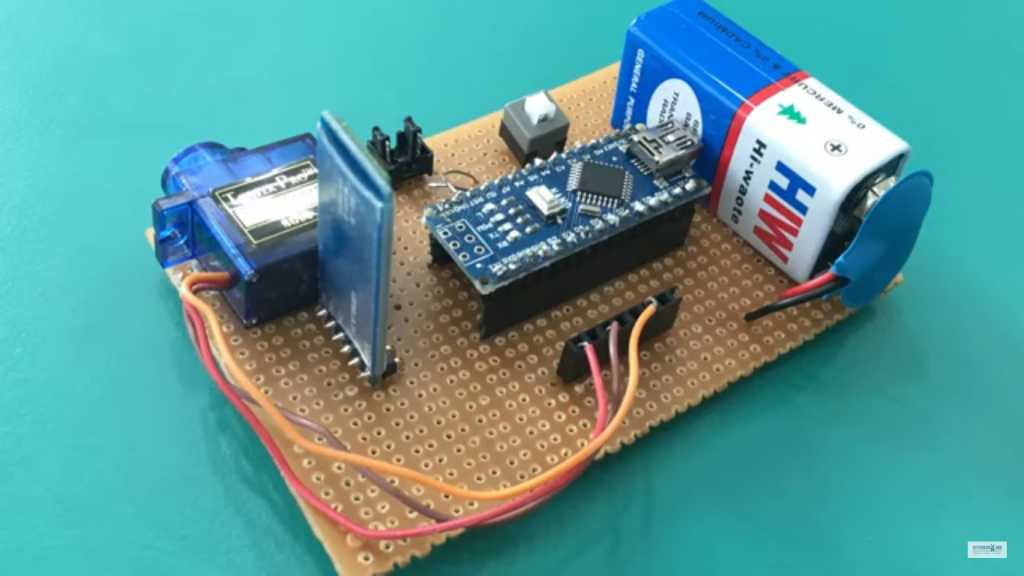