The Unmanned Railway Crossing system is a cutting-edge safety solution designed to automatically manage railway crossings without human intervention. This system uses ultrasonic sensors to detect the presence of approaching trains from a safe distance. When a train is detected, servo motors are activated to lower the crossing gates, ensuring that no vehicles or pedestrians can cross the tracks. Simultaneously, a buzzer sounds an alarm to warn anyone nearby of the approaching train, providing an additional layer of safety. By automating the process of closing the gates and sounding the alarm, this system significantly enhances safety and helps prevent accidents at railway crossings. This technology is crucial for students to understand as it integrates principles of electronics, automation, and safety engineering, demonstrating how modern technology can be applied to real-world problems to save lives.
Components:
- Ultrasonic Sensor: Detects the presence and distance of approaching trains.
- Servo Motors: Control the opening and closing of the crossing gates.
- Arduino Uno: Central microcontroller for data processing and control.
- Buzzer: Provides an audible alert to warn nearby individuals of an approaching train.
- Power Supply: Powers the Arduino and all connected components.
Setup and Installation:
- Connect the ultrasonic sensor to the Arduino’s trigger and echo pins (e.g., pins 10 and 11).
- Connect the servo motors to the Arduino’s digital pins (e.g., pins 8 and 9).
- Connect the buzzer to a digital output pin on the Arduino (e.g., pin 12).
- Power the Arduino via a USB cable or external power source.
Operation/Circuit:
- The ultrasonic sensor continuously monitors for approaching trains.
- The Arduino processes the sensor data and calculates the distance to the train.
- If the train is within a predefined distance threshold, the Arduino activates the servo motors to close the gates and sounds the buzzer.
- Once the train has passed, the Arduino reopens the gates and silences the buzzer.
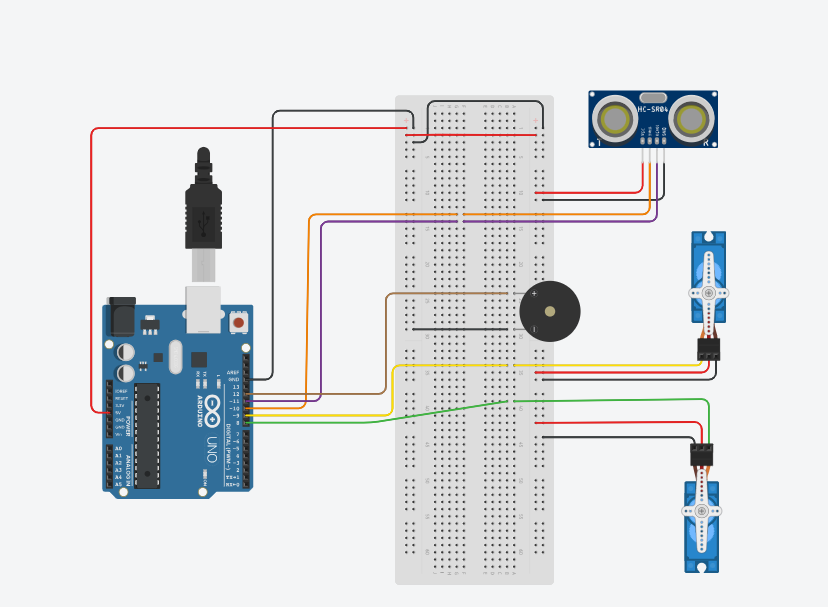
CODE
#include <Servo.h>
Servo leftGate; // Servo for the left gate
Servo rightGate; // Servo for the right gate
int trigPin = 10; // Ultrasonic sensor trigger pin
int echoPin = 11; // Ultrasonic sensor echo pin
int buzzerPin = 12; // Buzzer pin
int distanceThreshold = 10; // Distance threshold to trigger gate opening
float duration;
float distance;
void setup() {
leftGate.attach(9); // Attach left gate servo to pin 9
rightGate.attach(8); // Attach right gate servo to pin 8
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
pinMode(buzzerPin, OUTPUT);
}
void loop() {
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distance = (duration / 2) / 29.1; // Calculate distance in centimeters
if (distance < distanceThreshold) {
openGates(); // Call function to open gates
activateBuzzer(); // Call function to activate buzzer
delay(4000); // Keep gates open for 4 seconds
closeGates(); // Call function to close gates
deactivateBuzzer(); // Call function to deactivate buzzer
}
}
void openGates() {
leftGate.write(90); // Open left gate
rightGate.write(90); // Open right gate
}
void closeGates() {
leftGate.write(0); // Close left gate
rightGate.write(0); // Close right gate
}
void activateBuzzer() {
digitalWrite(buzzerPin, HIGH); // Turn on buzzer
}
void deactivateBuzzer() {
digitalWrite(buzzerPin, LOW); // Turn off buzzer
}
Testing and Calibration:
- Conduct thorough testing to ensure the system accurately detects trains and responds correctly.
- Calibrate the ultrasonic sensor for precise distance measurements.
- Test the servo motors and gate mechanisms to confirm smooth and reliable operation.
- Verify the buzzer’s effectiveness in providing clear and audible alerts.
Benefits:
- Enhances safety at railway crossings by automatically closing gates and alerting nearby individuals.
- Reduces the risk of accidents and fatalities at unmanned crossings.
- Eliminates the need for manual gate operation, making the system suitable for remote or unattended crossings.
- Provides real-time monitoring and response to approaching trains, ensuring timely action.
- Supports efficient and reliable railway operation, contributing to overall transportation safety.