Conserve water efficiently with an advanced Automatic Irrigation System powered by Arduino UNO, designed to optimize plant watering. This smart system includes several key components: a soil moisture sensor, a mini water pump, a relay module, an I2C LCD display, and jumper wires, all working together to ensure your plants get just the right amount of water, reducing waste.
Arduino UNO: The Brain Module, Arduino UNO, processes inputs from the soil moisture sensor and sends commands to the relay module to operate the water pump.
Soil Moisture Sensor: This sensor continuously monitors the moisture level in the soil. Inserted into the soil, it has two probes that detect the presence of water. The sensor sends an analog signal to the Arduino UNO, reflecting the moisture level. When the soil is dry, the signal value decreases.
Mini Water Pump: The water pump is responsible for delivering water to the plants. Connected to a water source, the pump is activated by the relay module when the soil moisture sensor detects low moisture levels, ensuring efficient water usage by watering only when necessary.
Relay Module: Acting as a switch, the relay module controls the water pump. Since the pump requires more current than the Arduino can supply, the relay module safely connects and disconnects the pump from the power source based on the Arduino’s commands.
I2C LCD Display: The display provides real-time updates on the system’s status, such as the current soil moisture level and the operational status of the pump. This allows for easy monitoring and adjustments.
Jumper Wires: These wires connect all the components to the Arduino, enabling communication between the sensor, relay module, pump, and display. They ensure seamless data transmission and power distribution throughout the system.
Together, these components create a fully automated irrigation system that precisely monitors soil moisture and waters plants only when needed. This not only promotes healthier plant growth but also significantly reduces water waste, making your garden more sustainable and environmentally friendly.
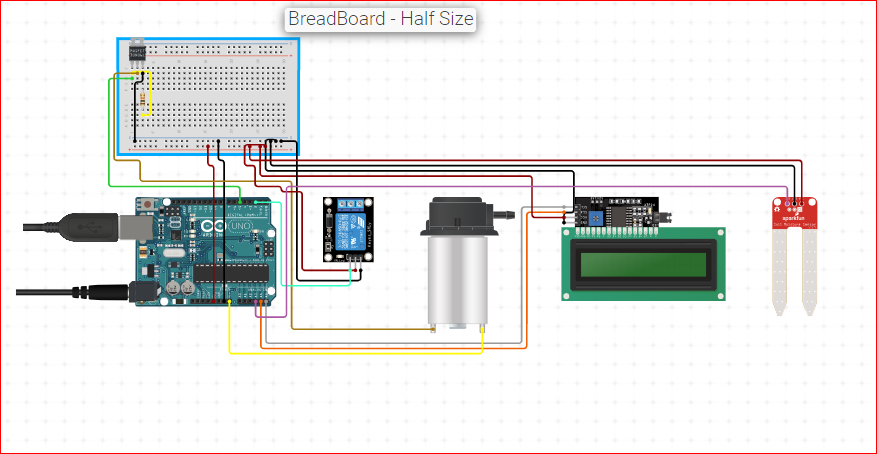
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
int soilMoistureValue = 0;
int percentage = 0;
LiquidCrystal_I2C lcd(0x27, 16, 2); // Adjust the I2C address (0x27) if needed
void setup() {
pinMode(3, OUTPUT);
Serial.begin(9600);
lcd.begin();
lcd.backlight();
lcd.print("Soil Moisture");
}
void loop() {
soilMoistureValue = analogRead(A3); // Read from A3 instead of A0
percentage = map(soilMoistureValue, 490, 1023, 100, 0);
Serial.println(percentage);
lcd.setCursor(0, 1); // Move cursor to the second row
lcd.print("Moisture: ");
lcd.print(percentage);
lcd.print("% ");
if (percentage < 10) {
Serial.println("Pump on");
lcd.print("Pump on ");
digitalWrite(3, LOW); // Relay ON
} else if (percentage > 80) {
Serial.println("Pump off");
lcd.print("Pump off");
digitalWrite(3, HIGH); // Relay OFF
} else {
lcd.print(" "); // Clear the message area
}
delay(1000); // Update every second
}