Behold is a device designed to help blind people make phone calls easily. It uses an Arduino board, a GSM module (like SIM800L), a button, a power supply (batteries), wires, and a small case. When the button is pressed and held for 3 seconds, the device makes a call to a pre-set phone number. To set it up, you need to connect the GSM module and the button to the Arduino, upload the code to the Arduino, and power the device. Once it’s ready, you can wear it on your finger. Pressing the button will automatically call the pre-set number, making communication easier for the blind. Behold Mobile For Blind project for student
Components:
- Arduino board-1
- GSM module (SIM800L)-1
- Button-1
- Power supply (batteries)-1
- Wires(M-M)-10
Connections
- GSM Module (SIM800L) to Arduino:
- RX Pin of GSM Module to TX Pin (8) of Arduino
- TX Pin of GSM Module to RX Pin (7) of Arduino
- VCC Pin of GSM Module to 5V Pin of Arduino
- GND Pin of GSM Module to GND Pin of Arduino
- Button to Arduino:
- One leg of the button to Digital Pin 2 (BUTTON_PIN in the code)
- The other leg of the button to GND
- Power Supply:
- Connect a suitable battery pack or power source to power both the Arduino and the GSM module. Ensure it provides stable voltage and sufficient current (GSM modules may require bursts of current during operation).
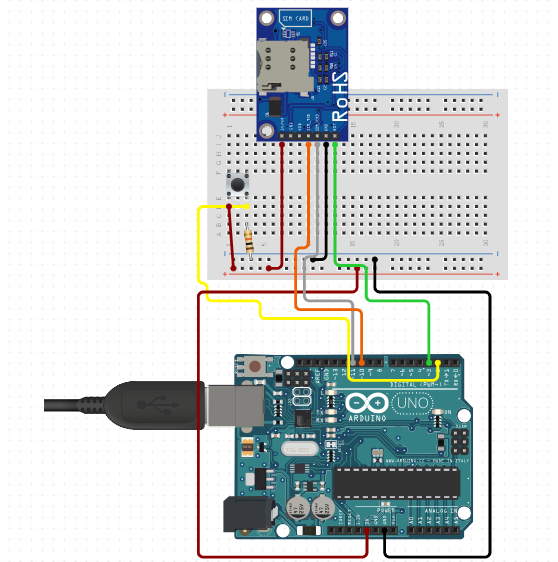
#include <SoftwareSerial.h>
// Define the pins for the GSM module
#define RX_PIN 11
#define TX_PIN 10
SoftwareSerial gsmSerial(RX_PIN, TX_PIN);
// Define the button pin
#define BUTTON_PIN 2
// Phone number to call
const char phoneNumber[] = "+1234567890"; // Replace with the desired phone number
// Time to hold the button (in milliseconds) before placing the call
const unsigned long buttonHoldTime = 3000;
void setup() {
// Start serial communication with the GSM module
gsmSerial.begin(9600);
// Initialize the button pin as input
pinMode(BUTTON_PIN, INPUT_PULLUP);
// Allow time for the GSM module to initialize
delay(10000);
}
void loop() {
unsigned long buttonPressedTime = 0;
bool callInitiated = false;
// Check if the button is pressed
if (digitalRead(BUTTON_PIN) == LOW) {
// Record the time when the button was pressed
buttonPressedTime = millis();
// Wait until the button is released or the hold time is reached
while (digitalRead(BUTTON_PIN) == LOW) {
// Check if the button has been held long enough
if (millis() - buttonPressedTime >= buttonHoldTime) {
// Place a call
placeCall(phoneNumber);
callInitiated = true;
break;
}
}
// Small delay to avoid multiple triggers if the button was released
delay(500);
}
// If the button was released before the hold time, do not place the call
if (!callInitiated && buttonPressedTime > 0) {
Serial.println("Button press too short, call not initiated.");
}
}
void placeCall(const char* number) {
// Send the AT command to dial the number
gsmSerial.print("ATD");
gsmSerial.print(number);
gsmSerial.println(";");
// Optionally, you can add code to wait for a response or handle errors
}