Project Description:
License Based Locking System project involves creating a smart verification system that uses RFID technology to ensure that only authorized individuals with valid licenses can start a vehicle. Additionally, the system includes an alcohol detection feature using the MQ sensor to prevent drunk driving. The project integrates an I2C LCD to display the status of the license verification and alcohol detection, ensuring enhanced safety and compliance.
Components Required
- Arduino uno with cable x 1
- RFID Reader MFRC-522 x 1
- RFID tags x 1
- MQ-3 Alcohol sensor x 1
- 16×2 LCD I2C display x 1
- Buzzer x 1
- Jumper wires – Male to Female x 13
Applications
- Vehicle Start Authorization: Ensures only drivers with valid licenses can start the vehicle.
- Prevention of Drunk Driving: Detects alcohol presence and prevents the vehicle from starting if the driver is intoxicated.
- Enhanced Road Safety: Reduces the likelihood of accidents caused by underage driving and driving under the influence (DUI).
Benefits
- Improved Safety: Reduces the risk of accidents caused by unauthorized or intoxicated drivers.
- Compliance: Ensures adherence to legal requirements regarding driving licenses and alcohol consumption.
- User-Friendly: Provides clear feedback to users via the LCD.
Conclusion
This RFID-based system enhances vehicle safety by ensuring that only authorized and sober drivers can start the vehicle. By addressing critical issues such as underage driving and drunk driving, the project promotes road safety helps prevent accidents, and saves lives. The integration of RFID technology, an alcohol sensor, and a user-friendly display makes this system a practical solution for modern vehicular safety challenges.
IMAGE:
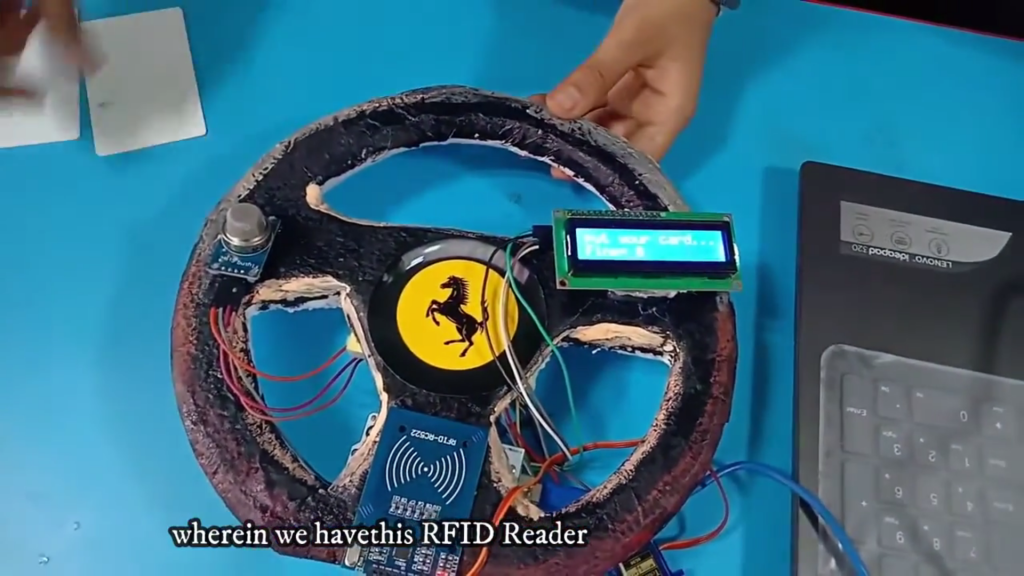
RFID Reader image:
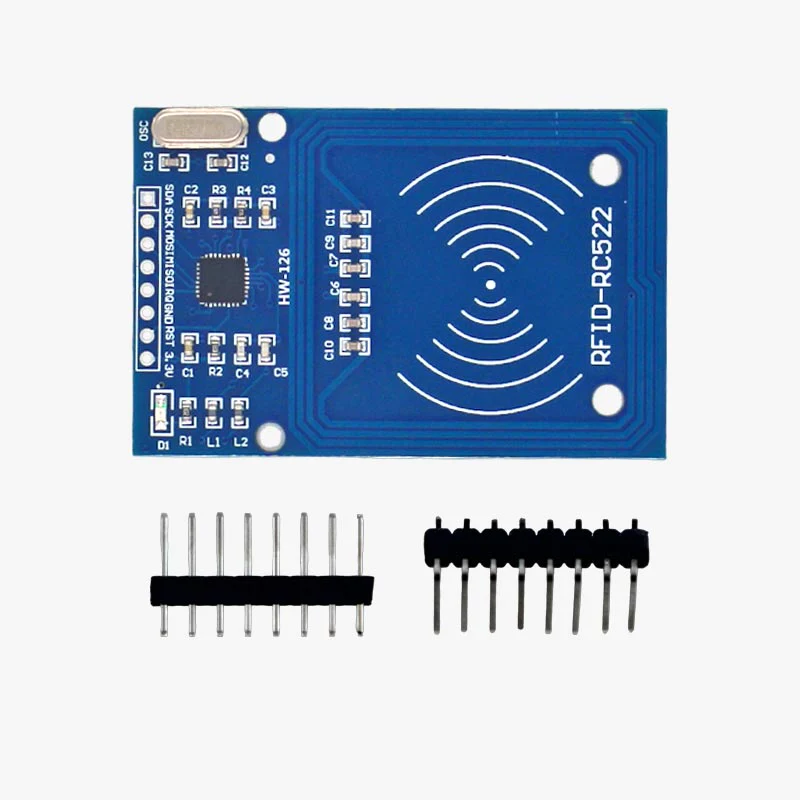
MQ-3 sensor:
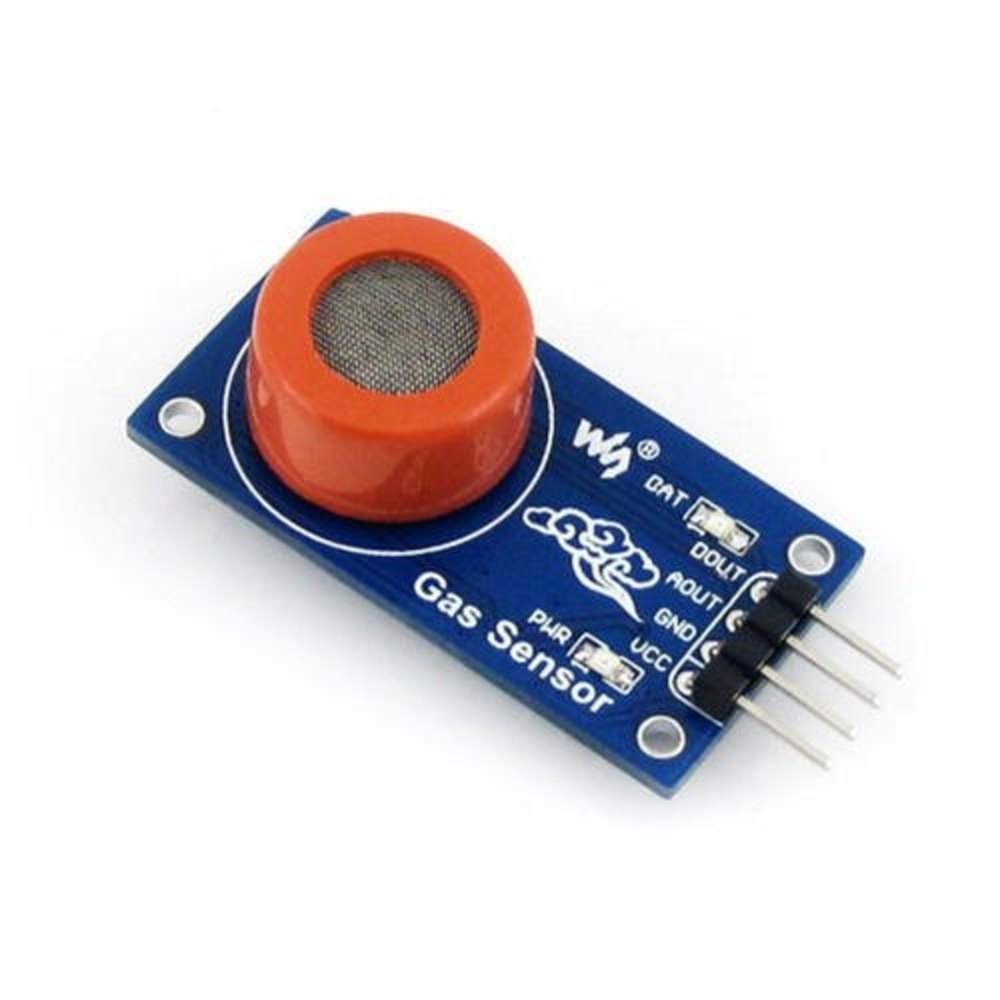
CIRCUIT DIAGRAM:
Connections:
- RFID Reader to Arduino:
- VCC to 3.3V
- GND to GND
- RST to Pin 9
- MISO to Pin 12
- MOSI to Pin 11
- SCK to Pin 13
- SDA to Pin 10
- MQ-3 Alcohol Sensor to Arduino:
- VCC to 5V
- GND to GND
- A0 to A0 (Analog pin)
- I2C LCD to Arduino:
- VCC to 5V
- GND to GND
- SDA to A4
- SCL to A5
- Buzzer to Arduino:
- Positive leg to Pin 8
- Negative leg to GND
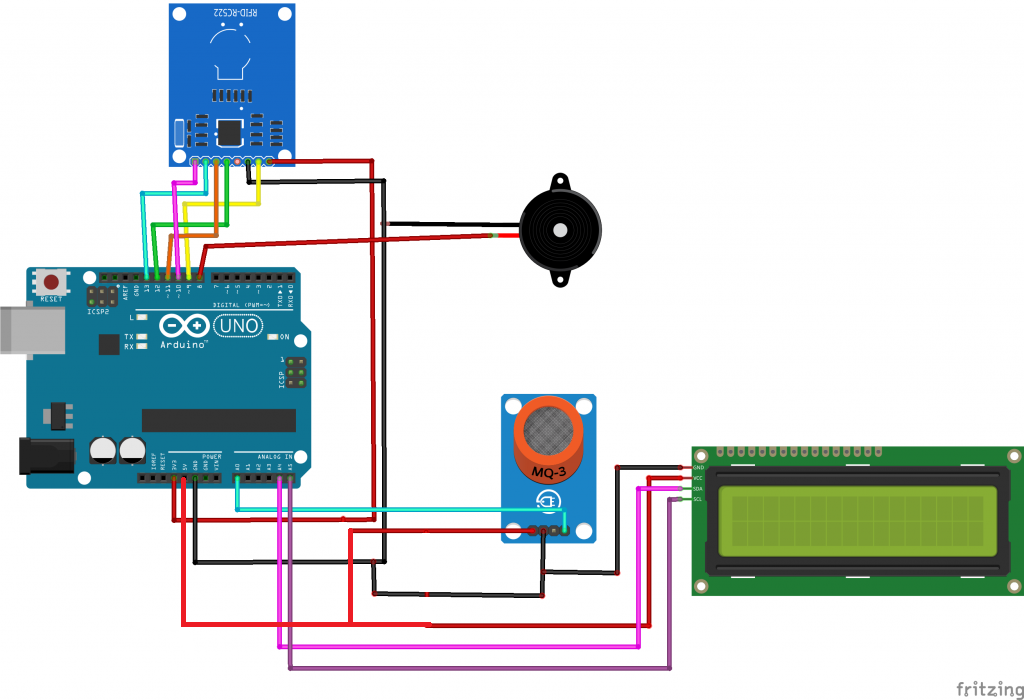
NOTE:
Before uploading and running the code IN ARDUINO IDE:
Go to > Sketch > Include Library > Manage Libraries… > Type the library name
OR Go to > Sketch > Include Library > Add .ZIP Library… download the zip library from the link given below.
LiquidCrystal – https://github.com/fdebrabander/Arduino-LiquidCrystal-I2C-library
MFRC522 – https://github.com/miguelbalboa/rfid
CODE:
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <MFRC522.h>
#define RST_PIN 9
#define SS_PIN 10
#define MQ3_PIN A0
#define BUZZER_PIN 8
MFRC522 mfrc522(SS_PIN, RST_PIN);
LiquidCrystal_I2C lcd(0x27, 16, 2);
void setup() {
Serial.begin(9600);
SPI.begin();
mfrc522.PCD_Init();
lcd.init();
lcd.backlight();
pinMode(MQ3_PIN, INPUT);
pinMode(BUZZER_PIN, OUTPUT);
digitalWrite(BUZZER_PIN, LOW);
lcd.setCursor(0, 0);
lcd.print("Place License...");
}
void loop() {
if (!mfrc522.PICC_IsNewCardPresent() || !mfrc522.PICC_ReadCardSerial()) {
return;
}
Serial.print("RFID UID:");
String content = "";
for (byte i = 0; i < mfrc522.uid.size; i++) {
content.concat(String(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " "));
content.concat(String(mfrc522.uid.uidByte[i], HEX));
}
content.toUpperCase();
Serial.println(content);
lcd.clear();
lcd.setCursor(0, 0);
// Compare the RFID tag's UID with the
if (content.substring(1) == "YOUR_RFID_UID_HERE") { // Replace with your actual RFID UID
lcd.print("License OK");
int alcoholLevel = analogRead(MQ3_PIN);
Serial.print("Alcohol Level: ");
Serial.println(alcoholLevel);
if (alcoholLevel > 300) { // Threshold value for alcohol detection
lcd.setCursor(0, 1);
lcd.print("Alcohol Detected");
digitalWrite(BUZZER_PIN, HIGH);
} else {
lcd.setCursor(0, 1);
lcd.print("Good to Go");
digitalWrite(BUZZER_PIN, LOW);
}
} else {
lcd.print("Invalid License");
digitalWrite(BUZZER_PIN, HIGH);
}
delay(3000); // Wait for 3 seconds before next scan
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Place License...");
digitalWrite(BUZZER_PIN, LOW);
}