The Air Care project is an advanced Air Quality Index (AQI) monitoring device designed to improve indoor air quality and promote healthier living environments. This innovative project utilizes components such as an Arduino UNO, an MQ-135 air quality sensor, a buzzer, an LCD display, separate red, green, and blue LEDs, and a charcoal-activated exhaust fan. The Air Care project addresses the critical issue of indoor air pollution, which can lead to various health problems such as respiratory issues and allergies. By providing real-time monitoring and alerts, it empowers users to take immediate action to improve air quality. The integration of the charcoal-activated exhaust fan adds a layer of active air purification, ensuring a healthier living environment. This innovative solution not only raises awareness about air quality but also actively contributes to cleaner air, enhancing overall well-being.
Components Required:
- Arduino UNO
- MQ-135 Air Quality Sensor
- LCD Display
- Red LED
- Green LED
- Blue LED
- Buzzer
- Charcoal-Activated Exhaust Fan
- Breadboard and Jumper wires
Component Descriptions
- Arduino UNO: A microcontroller board used for building digital devices and interactive objects that can sense and control physical devices.
- MQ-135 Air Quality Sensor: A sensor capable of detecting various harmful gases like CO2, ammonia, benzene, and smoke, providing a measure of air quality.
- 16×2 LCD Display: A liquid crystal display that can show up to 16 characters per line on two lines, used to display real-time air quality readings.
- Red LED: Indicates poor air quality when lit, alerting users to take action.
- Green LED: Indicates good air quality when lit, assuring users that the environment is safe.
- Blue LED: Indicates moderate air quality when lit, suggesting that air quality is acceptable but could be improved.
- Buzzer: Emits an audible alert when air quality reaches hazardous levels, prompting immediate attention.
- Charcoal-Activated Exhaust Fan: An exhaust fan equipped with charcoal filters that purifies the air by removing contaminants when poor air quality is detected.
- Breadboard: A tool for prototyping and testing circuits without soldering, used to connect all components together.
- Jumper Wires: Wires used to make connections between different components on the breadboard and the Arduino.
Circuit Connections:
- LCD (16×2) Display:
- RS to Arduino Pin 2
- EN to Arduino Pin 3
- D4 to Arduino Pin 4
- D5 to Arduino Pin 5
- D6 to Arduino Pin 6
- D7 to Arduino Pin 7
- VSS to GND
- VDD to 5V
- V0 (Contrast) to the middle pin of a 10k potentiometer
- A (Anode, backlight) to 5V
- K (Cathode, backlight) to GND
- MQ-135 Air Quality Sensor:
- VCC to 5V
- GND to GND
- A0 (Analog Output) to Arduino Analog Pin A0
- Green LED:
- Long leg (anode) to Arduino Pin 8
- Short leg (cathode) to GND through a 220-ohm resistor
- Blue LED:
- Long leg (anode) to Arduino Pin 9
- Short leg (cathode) to GND through a 220-ohm resistor
- Red LED:
- Long leg (anode) to Arduino Pin 10
- Short leg (cathode) to GND through a 220-ohm resistor
- Buzzer:
- Positive leg to Arduino Pin 11
- Negative leg to GND
- Power Connections:
- Connect Arduino to a power source (USB or external power supply)
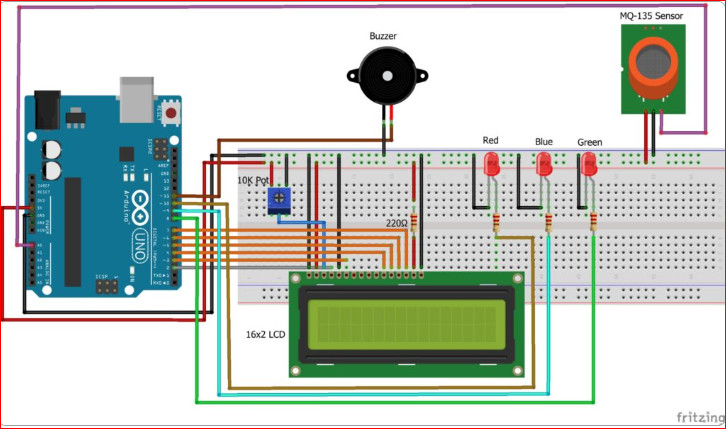
// Include library for LCD and define pins
#include "LiquidCrystal.h"
const int rs = 2, en = 3, d4 = 4, d5 = 5, d6 = 6, d7 = 7;
LiquidCrystal lcd(rs, en, d4, d5, d6, d7);
// Define pins and variable for input sensor and output led and buzzer
const int mq135_aqi_sensor = A0;
const int green_led = 8;
const int blue_led = 9;
const int red_led = 10;
const int buzzer = 11;
// Set threshold for AQI
int aqi_ppm = 0;
void setup() {
// Set direction of input-output pins
pinMode (mq135_aqi_sensor, INPUT);
pinMode (green_led, OUTPUT);
pinMode (blue_led, OUTPUT);
pinMode (red_led, OUTPUT);
pinMode (buzzer, OUTPUT);
digitalWrite(green_led, LOW);
digitalWrite(blue_led, LOW);
digitalWrite(red_led, LOW);
digitalWrite(buzzer, LOW);
// Initiate serial and lcd communication
Serial.begin (9600);
lcd.clear();
lcd.begin (16, 2);
Serial.println("AQI Alert System");
lcd.setCursor(0, 0);
lcd.print("AQI Alert System");
delay(1000);
}
void loop() {
aqi_ppm = analogRead(mq135_aqi_sensor);
Serial.print("Air Quality: ");
Serial.println(aqi_ppm);
lcd.setCursor(0, 0);
lcd.print("Air Quality: ");
lcd.print(aqi_ppm);
if ((aqi_ppm >= 0) && (aqi_ppm <= 50))
{
lcd.setCursor(0, 1);
lcd.print("AQI Good");
Serial.println("AQI Good");
digitalWrite(green_led, HIGH);
digitalWrite(blue_led, LOW);
digitalWrite(red_led, LOW);
digitalWrite(buzzer, LOW);
}
else if ((aqi_ppm >= 51) && (aqi_ppm <= 100))
{
lcd.setCursor(0, 1);
lcd.print("AQI Moderate");
Serial.println("AQI Moderate");
tone(green_led, 1000, 200);
digitalWrite(blue_led, HIGH);
digitalWrite(red_led, LOW);
digitalWrite(buzzer, LOW);
}
else if ((aqi_ppm >= 101) && (aqi_ppm <= 200))
{
lcd.setCursor(0, 1);
lcd.print("AQI Unhealthy");
Serial.println("AQI Unhealthy");
digitalWrite(green_led, LOW);
digitalWrite(blue_led, HIGH);
digitalWrite(red_led, LOW);
digitalWrite(buzzer, LOW);
}
else if ((aqi_ppm >= 201) && (aqi_ppm <= 300))
{
lcd.setCursor(0, 1);
lcd.print("AQI V. Unhealthy");
Serial.println("AQI V. Unhealthy");
digitalWrite(green_led, LOW);
tone(blue_led, 1000, 200);
digitalWrite(red_led, HIGH);
digitalWrite(buzzer, LOW);
}
else if (aqi_ppm >= 301)
{
lcd.setCursor(0, 1);
lcd.print("AQI Hazardous");
Serial.println("AQI Hazardous");
digitalWrite(green_led, LOW);
digitalWrite(blue_led, LOW);
digitalWrite(red_led, HIGH);
digitalWrite(buzzer, HIGH);
}
delay (700);
}