Hover Car Floating Car project aims to create a hover floating car that navigates on water using motors and fans, detects obstacles using two ultrasonic sensors, and can be controlled via a Bluetooth app. The car includes an alcohol detector to stop the motor when alcohol is detected, an RFID system with a servo motor for secure locking inside the car, and an I2C display to show messages.
Components Required
- Arduino MEGA – 1
- Ultrasonic Sensors (e.g., HC-SR04) – 2
- Bluetooth Module (e.g., HC-05) – 1
- DC Motors – 4 (2 for propulsion with motor driver, 2 for auxiliary functions with switches)
- Motor Driver (e.g., L298N) – 1
- Alcohol Sensor (e.g., MQ-3) – 1
- RFID Module (e.g., MFRC522) – 1
- Servo Motor – 1
- I2C LCD Display – 1
- Switches – 2 (for controlling auxiliary motors)
- Breadboard and Jumper Wires – Several
- Power Supply – For Arduino and motors
- Hovercraft body – For the floating base
Connections
Arduino to Ultrasonic Sensors:
- Ultrasonic Sensor 1:
- Trig to D2
- Echo to D3
- VCC to 5V
- GND to GND
- Ultrasonic Sensor 2:
- Trig to D4
- Echo to D5
- VCC to 5V
- GND to GND
Arduino to Bluetooth Module (HC-05/HC-06):
- Bluetooth TX to Arduino RX (pin 11)
- Bluetooth RX to Arduino TX (pin 10)
- Bluetooth VCC to 5V
- Bluetooth GND to GND
Arduino to Motor Driver (L298N):
- Motor 1:
- Connected to Motor A output of L298N
- Motor 2:
- Connected to Motor B output of L298N
- L298N Inputs:
- IN1 to 6
- IN2 to 3
- IN3 to 7
- IN4 to 2
- L298N Enable Pins:
- ENA to D8
- ENB to D9
Arduino to Alcohol Sensor:
- AO (Analog Output) to A3
- VCC to 5V
- GND to GND
Arduino to RFID Module:
- SDA to 53
- SCK to D13
- MOSI to D11
- MISO to D12
- RST to 15
- VCC to 3.3V
- GND to GND
Arduino to Servo Motor:
- Signal to D12
- VCC to 5V
- GND to GND
Arduino to I2C Display:
- SDA to A4
- SCL to A5
- VCC to 5V
- GND to GND
Switches to Additional DC Motors:
- DC_MOTOR_Switch 1:
- One terminal to motor 1 positive terminal
- Others terminal to motor 1 negative terminal and 5V through a pull-down resistor
- Switch pin to Arduino D14
- DC_MOTOR_Switch 2:
- One terminal to motor 2 positive terminals
- Others terminal to motor 2 negative terminal and 5V through a pull-down resistor
- Switch pin to Arduino D16
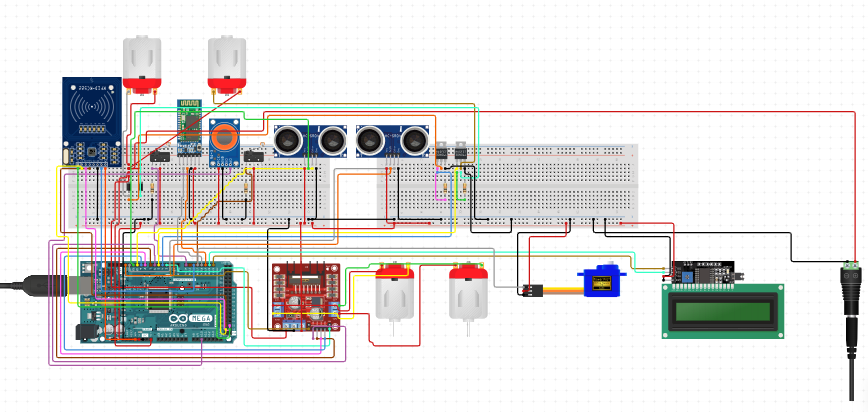
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#include <SoftwareSerial.h>
#include <Servo.h>
#include <SPI.h>
#include <MFRC522.h>
#define trigPin1 11
#define echoPin1 10
#define trigPin2 13
#define echoPin2 12
#define in1 6
#define in2 3
#define in3 7
#define in4 2
#define enA 8
#define enB 9
#define alcoholSensor A10
#define SS_PIN 53
#define RST_PIN 15
#define SERVO_PIN 17
#define SWITCH1 14
#define SWITCH2 16
#define ALCOHOL_THRESHOLD 300 // Adjust as necessary
SoftwareSerial bluetooth(11, 10);
Servo myServo;
MFRC522 mfrc522(SS_PIN, RST_PIN);
LiquidCrystal_I2C lcd(0x27, 16, 2); // I2C address 0x27, 16 column and 2 rows
void setup() {
Serial.begin(9600);
bluetooth.begin(9600);
lcd.begin(16, 2);
lcd.init();
lcd.backlight();
pinMode(trigPin1, OUTPUT);
pinMode(echoPin1, INPUT);
pinMode(trigPin2, OUTPUT);
pinMode(echoPin2, INPUT);
pinMode(in1, OUTPUT);
pinMode(in2, OUTPUT);
pinMode(in3, OUTPUT);
pinMode(in4, OUTPUT);
pinMode(alcoholSensor, INPUT);
pinMode(SWITCH1, INPUT_PULLUP);
pinMode(SWITCH2, INPUT_PULLUP);
SPI.begin();
mfrc522.PCD_Init();
myServo.attach(SERVO_PIN);
myServo.write(0); // Lock initially
}
void loop() {
long duration1, distance1, duration2, distance2;
// Ultrasonic sensor 1
digitalWrite(trigPin1, LOW);
delayMicroseconds(2);
digitalWrite(trigPin1, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin1, LOW);
duration1 = pulseIn(echoPin1, HIGH);
distance1 = (duration1 / 2) / 29.1;
// Ultrasonic sensor 2
digitalWrite(trigPin2, LOW);
delayMicroseconds(2);
digitalWrite(trigPin2, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin2, LOW);
duration2 = pulseIn(echoPin2, HIGH);
distance2 = (duration2 / 2) / 29.1;
// Obstacle avoidance
if (distance1 < 30 || distance2 < 30) {
stopCar();
} else {
// Normal operation, control via Bluetooth
if (bluetooth.available()) {
char command = bluetooth.read();
controlCar(command);
}
}
// Alcohol detection
int alcoholLevel = analogRead(alcoholSensor);
if (alcoholLevel > ALCOHOL_THRESHOLD) {
stopCar();
lcd.clear();
lcd.print("Alcohol Detected");
} else {
lcd.clear();
lcd.print("All Clear");
}
// RFID check for lock
if (mfrc522.PICC_IsNewCardPresent() && mfrc522.PICC_ReadCardSerial()) {
unlockCar();
}
// Control auxiliary motors with switches
if (digitalRead(SWITCH1) == LOW) {
// Code to control motor 1
digitalWrite(motor1Pin, HIGH); // Adjust motor1Pin accordingly
} else {
digitalWrite(motor1Pin, LOW); // Adjust motor1Pin accordingly
}
if (digitalRead(SWITCH2) == LOW) {
// Code to control motor 2
digitalWrite(motor2Pin, HIGH); // Adjust motor2Pin accordingly
} else {
digitalWrite(motor2Pin, LOW); // Adjust motor2Pin accordingly
}
delay(100);
}
void controlCar(char command) {
switch (command) {
case 'F':
moveForward();
break;
case 'B':
moveBackward();
break;
case 'L':
turnLeft();
break;
case 'R':
turnRight();
break;
case 'S':
stopCar();
break;
}
}
void moveForward() {
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
digitalWrite(in3, HIGH);
digitalWrite(in4, LOW);
}
void moveBackward() {
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
digitalWrite(in3, LOW);
digitalWrite(in4, HIGH);
}
void turnLeft() {
digitalWrite(in1, LOW);
digitalWrite(in2, HIGH);
digitalWrite(in3, HIGH);
digitalWrite(in4, LOW);
}
void turnRight() {
digitalWrite(in1, HIGH);
digitalWrite(in2, LOW);
digitalWrite(in3, LOW);
digitalWrite(in4, HIGH);
}
void stopCar() {
digitalWrite(in1, LOW);
digitalWrite(in2, LOW);
digitalWrite(in3, LOW);
digitalWrite(in4, LOW);
}
void unlockCar() {
myServo.write(90); // Unlock position
delay(3000);
myServo.write(0); // Lock position
}