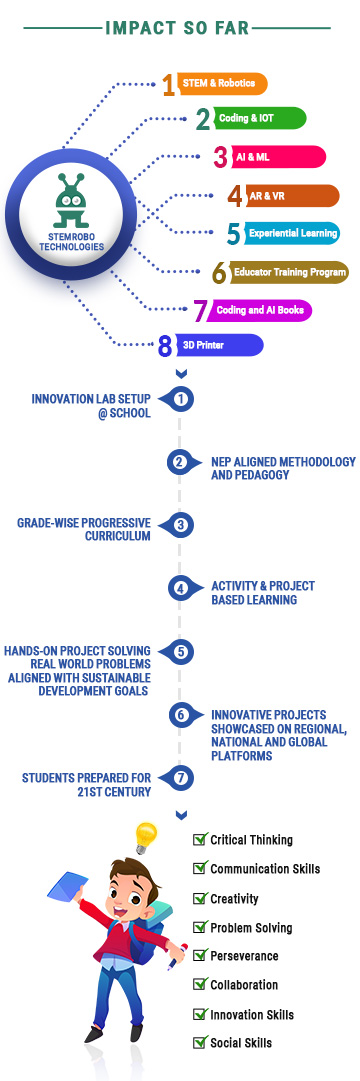
Welcome to the STEMROBO Projects Gallery, a hub of innovation and creativity. Our students, using tools like Tinker Orbit, Bitli, STEMBOT, and more, are developing projects that address community issues and explore sustainable development goals. The STEMROBO community, spanning over 30+ countries, is fostering 21st-century skills and nurturing future tech entrepreneurs. Join us in this journey of problem-solving and learning. Welcome to the future!